✅ WPF - Detect if screen resolution is resized (ex. 125% or more)
I'm writing a tool in which I need to first set the screen resolution, I currently have a 1920 x 1080, but I noticed that if in Windows screen resolution I enable the automatic resizing to 125% (or more) the tool retrieve false values.
Ex. for 125% the software think that my resolution is: 1536 x 864.
Is there an easy way to detect if is this enabled, and maybe also detect the % value?
I'd like to make something like that.
Or better:
If it's possible it will be nice, if it's not possible or too hard to make I'll go to ask to the user those data.
3 Replies
don't crosspost
Cyberrex#8052
Embed Type
Link
Quoted by
<@!263696696523882496> from #chat (click here)
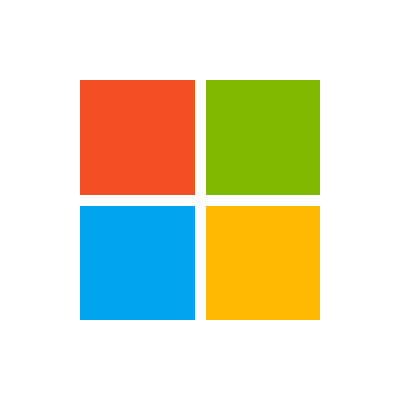
React with ❌ to remove this embed.
too hard, I don't understand it, and it report C++ code.
I solved using (from https://learn.microsoft.com/en-us/archive/blogs/jaimer/getting-system-dpi-in-wpf-app):
Thinking better on it I think I could directly remove the
else
, or even better, the whole if
statement, since if DPI scaling is not enabled, the resolution will be multiplied * 1, and so the value will be right anyway.