✅ ConsoleApp - run the .exe by cmd and catch given parameters
Well, as a little task I'm trying to code a ConsoleApp that should base64 encrypt an input string and automatically copy it to the clipboard.
The ideal result is the following, by cmd:
-
-base64.exe -e string (for encrypt)
- -base64.exe -d string (for decrypt)
First of all I played with encoding/decoding and I figured out that I have to convert the input string into a byte (array?), then after I can apply the method that convert to base64.
I succesfully "wrote" the encrypt method, now something I don't know how to do, it to catch one or more parameter, I don't know how is technically called this, I'd have googled it.
But what I finally understood, is the meaning of:
I always wondered which is the sense to write string[] args
if the program works perfectly without arguments, and now I know that the meaning of this is somehow to accept and handle cmd.exe parameters as arguments.27 Replies
I made a try and with surprise, I got this error:
Operator '==' cannot be applied to operands of type 'string[]' and 'string'
I don't understand why but when I normally make a compare with strings using ==
I don't get any errors.
Ok, it works using this form
string[] means an array of string - a collection. you got that error because it doesn't make sense to compare a collection of things to a single thing
Main()
gives you an array of strings because people can give as many arguments as they want. to parse them you have to pluck out the individual arguments you want from the array, as you've doneYeah I solved specifying the position of the array to check.
In my case i checked position 0 for -e and position 1 for -d, but I have a doubt.
In all consoleapps you can type arguments in whatever order you want, did they use some existing function, like "if array contains" ?
the Array class has a bunch of static helpers
Something I don't understand, is why the compiler force me to set
base64encode()
and base64decode()
as static
instead of public
, when I studied modifiers, I read that public
is the most open modifier that make an object accessible from everyone from everywhere, and static
make the same thing, but only if you remain in the same class, so public
should works anyway with this logic.things can be
public static
public has nothing to do with static
that's ok, but the point is that the program require
static
as mandatorybecause the main method is static
oh
so all change now
damn, most of guides about modifiers just explain the effect they have on variables
you should either learn some basics of classes, or just ignore it for now and use static everywhere
also don't declare your variables up front
it's not C99
I always used public everywhere till now, but I was told that's ok but not a good way to write the code
public is completely independent of static
it's a completely different feature
so I should declare and assign at the same time
or not declare at all
you never use
plainTextBytes
in that method btwI thought about this solution, idk if is always a good practice to reduce the code as max I can
absolutely not
if you feel like making the declarations, that's fine
first to understand of args[] works I just put all in the main() for try base64encoding, and I was able to reduce all in one row, it was cool but maybe less clear, I made this but I reverted it
I also putted Console.ReadLine() in the same row
I learned this reduction method by writing Excel formula
yeah i'd say try to avoid that
it's a nuance and there's no real rule for it
you just have to do what feels right
ok
I added this little check to the Main() since I noticed that the app immediately crashes if I try to debug from VS since find the array empty (since the console call the Main() method without args).
My doubt is, if for some reason I don't handle every possible errors, and the app crashes, is there a way to generate a
.txt
or a .log
that contains the error description?
I remember a dev of a program, in the first releases putted try catch + clipboard.settext(exception)
literally everywhere, telling users to forward him errors, but I don't know if is a good practice to spam this statement everywhere.again, setting the clipboard of the user is bad practice already
there are libraries which handle option parameters like this for you
yeah I know this, I mean that this dev catched all errors with try catch, maybe instead of set the text to clipboard I could generate a .log
I believe you've learned about guard clauses. make one that displays how to call the program correctly if there are no args
you can just print it to the console and pipe it into a file
program.exe -e > file
i would just rely on others to do the work i don't feel like doing
like Spectre.Console
can't mess up user input with that
very cool^^ now I understand much things I seen on some beauty consoleapps I seen
btw this is an example, but what about if my app crashes for another error that I have not considered?
normally, if I'm not wrong, the user playing the app will just see "application.exe stopped working" without any explanations, so he'll never able to tell me what really happened.
a solution (maybe dirty) would be to put try catch on every method and every actions the app make, in the paste I did it creating some random codes, example code error 1, code error 2, and so, in that way when the user tell me the code error I can identify the bugged piece of code easily.
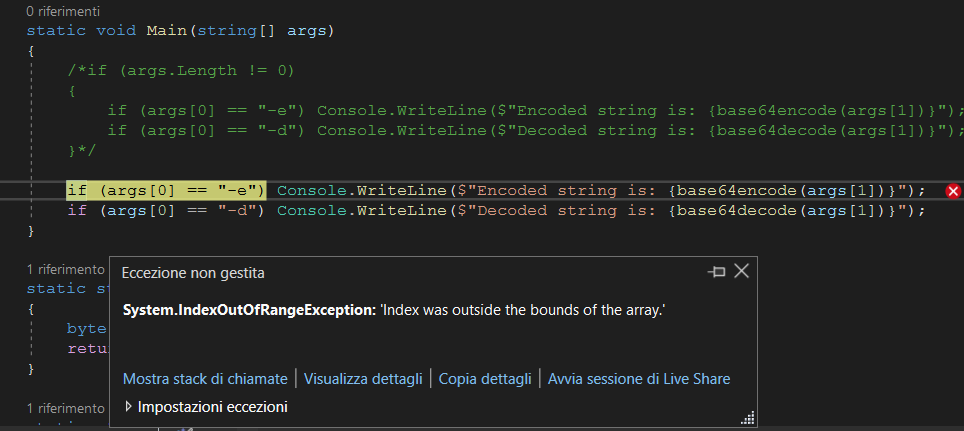
https://learn.microsoft.com/en-us/dotnet/api/system.diagnostics.trace?redirectedfrom=MSDN&view=net-7.0
Idk if this could works for what I'm looking for.
I read also about logging libraries like Serilog, but I think it's not for errors reporting.
Trace Class (System.Diagnostics)
Provides a set of methods and properties that help you trace the execution of your code. This class cannot be inherited.