✅ (Very Begginer) - I Get errors when I can't access method within other class and syntax
I have a class " Course " and a class "University", in class Course there is a Code for each teacher (Between 1 and 15)
I need to find the MostCommon teacher that has the most Courses in the University.
$code
130 Replies
To post C# code type the following:
```cs
// code here
```
Get an example by typing
$codegif
in chat
If your code is too long, post it to: https://paste.mod.gg/
The access other class error is fixed
But there is syntax error with MostCommon
(int MostCommon)
And if you know a more efficient/better way of doing this I will be very glad to hear it
Because I'am pretty positive mine is not so good
I had to put "return y" out side the loop because it said that "not all code paths return"
And now I get syntax error with y as well
you probably want
$scopes
$scopes
thing a
is available in scope A
and scope B
thing b
is available only in scope B
You are trying to return y which was defined inside your for loop.
I assume that causes your syntax error.
But isn't it if it's inside the scopes of the whole method
it should be defined inside all of it?
You could define y at the very top so it's in scope for all of it.
It didn't change much xd
I guess the problem is that it's value is defined inside the loop
but I don't know how can I define it without getting the error
because I need the loop to define it
Give me a second, had to switch from phone to pc
Yeah I noticed that xd
Thank you
No idea how people get stuff done on their phones. Just awful all around.
Anyway, so I see now what this is supposed to do
If we start at the top, you know how many courses there are from
PlaceCourse
no?Only if you could see my screen...xd
YOu mean like what is the size of PlaceCourse?
If so, yeah that's a 1000
private Course[] PlaceCourse;
private int current=0;
public University()
{
PlaceCourse = new Course[1000];
}
done it in a constructor
previoulsy
Oh wait, I'm dumb yeah that makes more sense
Array contains up to 15 ids for each of the teachers
Are you sure they go from 1 to 15?
yes
that is declared in the question
because you are accessing the array with indices 1 to 15 then, but it only goes from 0 to 14
if GetCode ever returned 15 for any teacher, this would explode
Oh yeah, Ig it's better to write it as 16
and start from 1
you could just use
GetCode() - 1
to index into the array.
You should probably leave it starting at 0, that's pretty standard.But then it will return the index with the code-1
And that won't be the right teacher
It will be if you always access it like that
Lets say you ask "How many courses does the teacher with Id 8 have"?
its
arr[8 - 1]
i.e. the 8th index of your array.
Which by convention starts at index 0, not 1 🙂True, but the question wants me to return a number between 1 and 15
I supposed you could just increase it to 16 and just leave the 0 slot empty, but I would suggest that that's not good practice and is more likely to confuse you later.
You can still do that
So you want the most common teacher Id from all the courses.
yeah
You already define
int MostCommon
so thats almost certainly going to be what you want to return
rather than 1
Now lets see how you set itThe ' y ' is the Index (ID of the teacher)
I see you are looping once to find the actual number of courses
And then again to find the index of that count
You could probably just do that in one go
Once to find the teached with the most courses and then to find his index
yeah
something like
Deal with the teacher id like its 0 based for indexing purposes, then only add back one to make it human-readable at the end.
Something like this, I don't know.
There is probably something nicer you can do, but this should work.
Oh
wait
Let me process that real quick xd
actually, let me make a quick edit, that was dumb by me
This is better, so you can just cleanly return
mostCommon
Technically this doesn't cover a case where no teacher has any courses, not sure what you'd want to happen there.the question says that there is one for sure
$code
To post C# code type the following:
```cs
// code here
```
Get an example by typing
$codegif
in chat
If your code is too long, post it to: https://paste.mod.gg/
Won't it just returd 0+1 every time now?
return*
Only if Index never gets set.
That would be the case, if arr[0] is already the most common id
also, you are skipping 1 at the end
in your for loop condition
oy vey
forgor to delete it from previous
You dont need to do that anymore, since oyu arent comparing neighbouring indices .)
Yeah
so even tho it's value is redeclared inside a loop
It will see that?
You aren't redefining it in this case.
Index refers to a variable outside the loop
you are just assigning to it
But that doesn't need to happen, if that assignment never gets hit,
Index
just retains the value you defined at first
0, that isBut before that I refereed to a variable outside the loop as well
Wdym?
If the first teacher has the most Courses
I declared the Index (y) outside the loop before that as well, and only assigned its value inside the loop
and it had syntax error
Yeah you did
You declared it outside one of your loops
But you had two nested loops
No no, they were separated
So
y
existed inside both loops, but NOT outside the outer loop where you tried to return it.public int Mostcommon()
{
int[] arr = new int[15];
for(int i = 0; i < PlaceCourse.Length; i++)
{
arr[PlaceCourse[i].GetCode()]++;
}
int MostCommon;
for (int x = 0; x < arr.Length - 1; x++)
MostCommon = Math.Max(arr[x], arr[x + 1]);
int y;
for (int y = 0; y < arr.Length; y++)
if (MostCommon == arr[y])
{
y = arr[y];
}
return y;
}
Ah I cant read
Formatting is a bit whack
Give me a second to process that.
xd, np, thank you
Oh I see the problem
i was confused. You were right about the loops being separated.
Btw, it's probably best to always use curly braces.
I know they are optional for one-liners like this, but jesus does that make it hard to format and read 😄
xd
You are redefining
y
as your loop iterator
I'm pretty sure that's why its blowing upiterator?
wdym
You are using an int called
y
in your second for loopaaaa
omg didn't see that
I keep forgetting what the rght word for that is
you redefine it there, causing it to only exist for the scope of the second loop 🙂
I'm like 90% sure that's whats causing your syntax error.
nope
xd
I renamed it to z
and still the same
And that, kids, is why we don't use single letters for variable names 😉
except in loops 😛
and there is syntax error with MostCommon as well
True xd
Still broken? mmmh
yep xd
public int Mostcommon()
{
int[] arr = new int[15];
for (int i = 0; i < PlaceCourse.Length; i++)
{
arr[PlaceCourse[i].GetCode()]++;
}
int MostCommon;
for (int x = 0; x < arr.Length - 1; x++)
MostCommon = Math.Max(arr[x], arr[x + 1]);
int z;
for (int y = 0; y < arr.Length; y++)
if (MostCommon == arr[y])
{
z = arr[y];
}
return z;
}
sorry that I send it like this
it's just tedeous to recopy the $code thing
To post C# code type the following:
```cs
// code here
```
Get an example by typing
$codegif
in chat
If your code is too long, post it to: https://paste.mod.gg/You might need to initialize MostCommon
Before accessing it the first time
initialize?
set it to some initial value
hm
It doesnt like the comparison
Because that might be the first thing that happens, before any assignment
And it would not be set to anything valid at that point
But isn't it like, if it doesn't see that I set it to a certain value inside a loop, it won't see it changing inside a loop?
can you rephrase that? i dont quite follow
If it gets syntax error, when I declare the value of the MostCommon inside a loop, why would it see it when I change the value of it, from lets say 0?
I#m not entirely sure what you mean, still 😄
Uno momento
I try another way xd
What I suspsed the syntax error is, has to do with the comparison in the if
*suspect
jesus
yes
You aren't declaring MostCommon in the loop, just to be clear
You are simply assigning a value to it
and that ones perfectly fine.
yes
But I think what the compiler doesn't like, is the comparison in the if
That's what I did in my code as well
Because at that point, MostCommon might not yet have been set even once, making the current value kind of not valid.
aaaaaa
By setting it where you declare the variable
That makes sense now
You are letting the compiler know "hey this has been properly initialized, you can assume that whatever value it holds is valid in some way"
Yes man, you fucking gigachad xd
I think that is why you are getting the error, but you should also be able to hover over it and see the error message btw 😉
Let me see if that works real quick
Microsoft don't explain it as good as you do xd
Yeah, not to toot my own horn, but Microsoft docs aren't brilliant if you are a beginner
yep xd
They are technically good though, really thorough on a lot of things. Just a bit too dense sometimes 🙂
I mean some times they do help
but it's pretty rare
So I much prefer to speak with a human xd
yeah man, that fixed it xd
Noice
So, just for completeness sake
This is what I believe is roughly a good structure
Btw, local variables we case
likeThis
Technically not required, but easier for others to read since it is the convention 😉wut xd
Didn't understand what you mean by that
"Camel case"
It's the commonly agreed way of writing local variable names
firstLetterLowerCase
You are doing PascalCase
Oh
First letter uppercase
Really?
camel case is the convention for local variables at least
and method parameters
oh
As I said, it works either way, but if you share your code, it will probably be more readable if you stick to convention 😉
So only methods start with Upper case and every word start with upper?
And local vars are with lower then every word with upper?
Methods and Class names are generally PascalCase
So first letter upper case, yes
that's something new xd, will be sure to stick to that then
Some other naming conventions are a bit more controversial and not everyone does the sam
but these two are pretty much universal in C#
If not a secret
Where did you learn to code?
/ How
Just on my own to be honest 😄
I technically went to a sort of school for it for a little bit
But 99% of what I know I taught myself
Mostly making game stuff
Unity first, now Godot
Some WPF app stuff
general console app shenanigans
Whatever I felt like
That's cool man
Big gaps though, I know absolutely nothing about databases or networks, so... 😄
Bruh, trust me, just let me get to your level first, and that will be great already
Because like from what I know
Very flattering
There is like tons of shit that are not conventional
When I look at people who I consider great programmers, I feel like an absolute dunce
and are not even encripted in the basic visual studio
and you have to add it manualy
like " Lists "
and stuff
And like I don't know how can you remember all that
Yeah, but honestly stuff like typing out
using System.Collections.Generic
at light speed
becomes second nature after some time 😄
Amen
xd
Like that's where the programing really beggins I guess
Just stick to it, it'll come to you faster than you think.
because what I'am doing now is just math shit
and not reall things
What's even real? 😉
Really, knowing syntax is a minor detail at the end of the day.
Learn how to understand, compartmentalize and solve logical problems.
Like lets say, with the help of chatgpt I made a automate mouse clicker that clicked in certain places on screen with differnet intervals of time
ye
and I used it to aumate playing in a casino where every 15 minutes I get 0.03 cents
that's a real program
xd
automate*
I see 😄
Yep, if you can think logicaly and technicalities will come with time ig
That's why I like making games in easily accessible engines.
You get to make lots of real shit while much of the scaffolding is taken care of and you can actually get somewhere, even as a beginner.
the masterpeace of "Cntrl+c, cntrl+v"?
xd
Alright, I gotta hustle
Cya, man, thank you very much for everything
Pump some iron, bruh
welcome
Any other questions, feel free to ask.
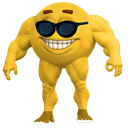
I'am sure there will be xd
Or ask in the forum like before, up to you 😉
kk good luck, have fun coding and keep at it!
♥️ ♥️ ♥️ ♥️