❔ Why Serilog doesn't loggin into a MSSQL Database
Hi dear friends,
I'm working on a solution that contains 5 projects, (
Core
, IdentityCore
, EFCore
, CQRSCore
and WEB
), the WEB
project is an ASP.NET Core 7 API
project.
Recently I tried to integrate the Serilog
logger in the project.
I created the following entity that represents a Log
:
AppUser
is a class that Inherits
from IdentityUser
.
in the AppDbContext
I add the Log
as a DbSet<Log> Logs
.
And I configured in the Serilog
in the Program.cs
of the web
project like this :
And to log from Controllers
I use like the following example :
With all these and no logs stored in the DB, please from your experience how do I can solve this issue ?36 Replies
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
no
everything goes fine but no logs stored in the
Logs
tableare the logs also emitted to a file/console? Maybe serilog logs why it cannot write to the DB
From this expression:
I think I forced it to log in a database
you can add additional sinks right? e.g. the console
yeah
But my goal is to log in a database
yes but maybe the logs in the console will tell you why it's not able to write to the database
and then you can remove that afterwards
hmmm, I was thinking about this idea
I will try it now and see
@Tvde1 it loggs fine in the
Console
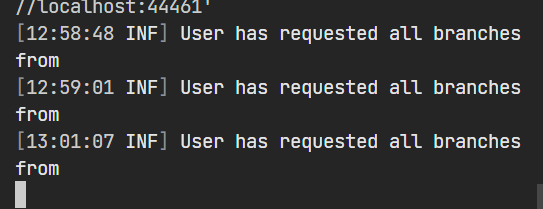
IDK why it not loggs in Database
hmm and it doesn't say in the console that db logging doesn't work?
no
Do you have any idea ?
are there any interesting options in MSSqlServerSinkOptions?
maybe on the DB you can see a log of connection attempts
should serilog create the table in the DB or is it already created? Does it match the columns that serilog expects?
I used
SQL Server Profiler
but I see only the used query to get Branches
but no query related to `Logs'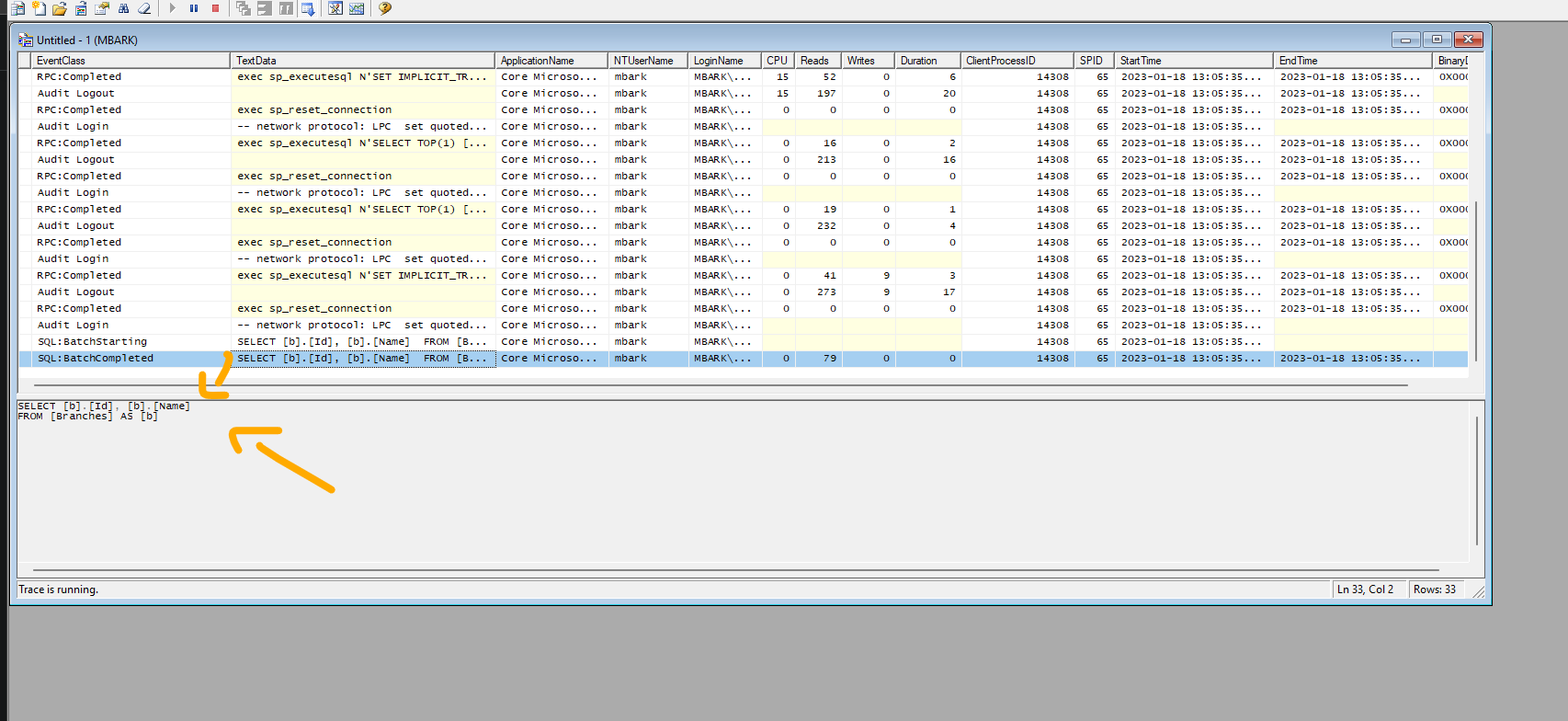
Yeah there is an option
I used the following configs but still nothing
and the
DefaultConnection
is a valid connection string that can create tables?Yeah, it is the same
Connection String
I used with EF Core
and by the time this code runs, the
configuration
already contains appsettings.json
and user secrets etc
you can verify this by Debug.WriteLine the configuration.GetConnectionString("DefaultConnection")
at that point
it has happened to me before where I used configuration
before the configuration was actually filled with appsettings and user secretsThe same with me I already worked before in a project with this method and it works but I used a different technique of configs
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
I actually not want to real log, I want to use
Serilog
for a simple Audit
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
I never had any experience with
ElasticSearch/Kibana
is they simple to implement and understood ?Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
so
ElasticSearch/Kibana
used to store Loggs
?Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
hmmm interesting, is it simple to implement it?
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
Yeah, but I want to practice
ElasticSearch/Kibana
as you said, I want to take this new experience tooUnknown User•2y ago
Message Not Public
Sign In & Join Server To View
I mean by simple is it simple to setup and register thier services (ElasticSearch/Kibana) and hsing them too, or it needs some architectural considerations or something like taht ?
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
hmmm, looks interesting, I will take this experience after finishing the current issue with
Serilog
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.