❔ ✅ is it possible to ''lock'' a var after the first assignment?
I'm making some base practice with C# with some exercises.
I completed the first one, and it have a second part that ask an additional answer.
Here's the code:
65 Replies
I'm not sure that in the second part it wants both answers, I mean including the answer of the first step, but I'd like to make it in this way for learn better.
I know that consts wont work since it can be assigned only while declaration.
Also ass the
x
value to i
wont work since it will change every time until the cycle is not end, and it will print the last one, and the exercise want to know the first one.
And I can't even stop the cycle when I get -1
value, since it's necessary for give in output the first answer.
The only solution I actually thought functional is to use an array for collecting all values, and then just printing the first one putted in, but I don't know if is a legit way to achieve it^^
this seems work, I can improve it by not using an array but another collection items that doesn't require a fixed amount of items, so I don't have to put in some random values.
it will remain the fact that I'll use it for store multiple values also when I just need the first one.
I don't understand what the last if do.
Also basement is never affected by the cycle.
If I'm not wrong, in this way you'll print the last -1 position, instead printing the first one.
that's exactly what the if prevents
only set
basement
if it has never been set beforeoh right right
definitely more clear than my solution
I thought to improve this replacing the array with an arraylist but your solution seems more clean
isn't better to define
int
vars as short
in order to consume less resources?and btw why the second way to declare an
ArrayList
is flagged as recommended? it doesn't explain why.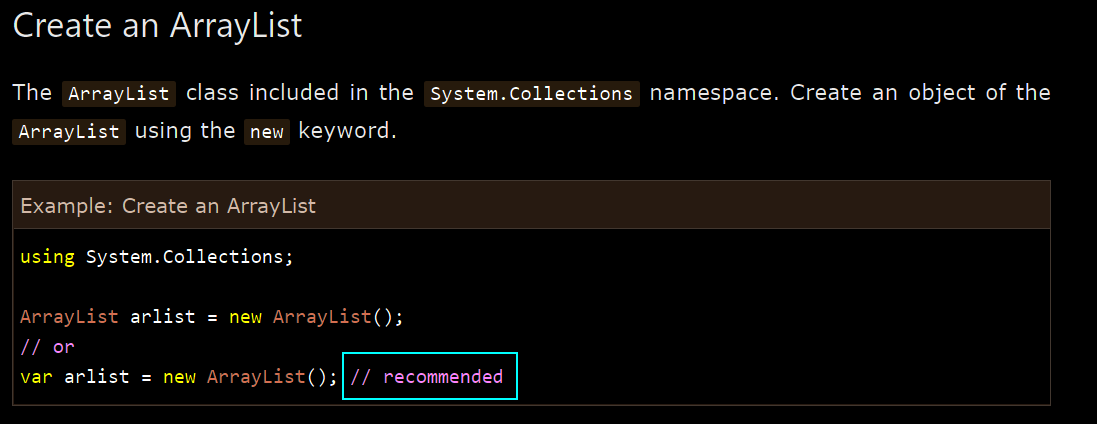
is there a way to select which parameter I want to get and print?
in this way it print both since the called function return 2 parameters

Solved using this
Last question, I think, I wanted to proceed with ArrayList first as an update of my version, before go with your solution that's better.
I noticed that if I never go to floor -1, the ArrayList will stay empty, and the program will crash.
I could resolve it in 2 ways, at least I thought just those 2:
1) putting a random value into it for avoid making it empty (sounds like dirty xD)
2) using Try Catch statement for handle this exception
Do you suggest to use one of those solutions, or you have a third one?
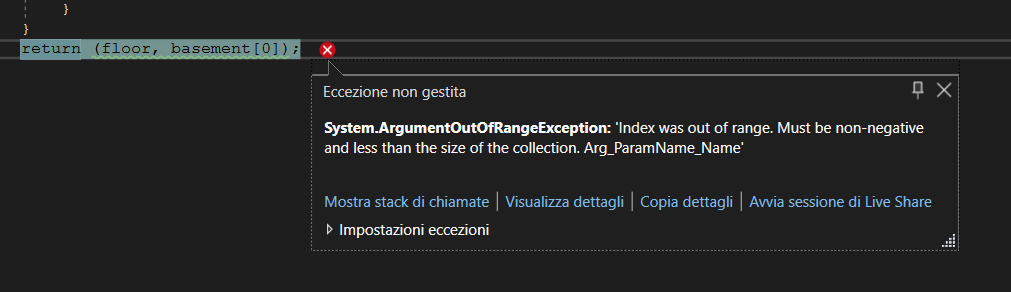
don't use arraylist
it's a relic of literal decades ago
yeah I know that in this case is a bad choice.
is also discontinued?
it's a bad choice in every case
I mean old and not used anymore
it's not generic, therefore not type safe
which collection should I use when I need to use something like
ArrayList
?I tried this just for make practice, and I'm not understanding why it execute this instruction twice

you either use an array, or a list
ok
I can't use
instead of
cause the function is setted to return two
short
values, so I suppose that if I want to print never
I should put an if statement to the calling instruction like if second parameter == 0 print that santa never accessed the basement else print the value
or is there some cool trick I don't know?null
it seems I can't put those instruction in a ternary operator

$tryparse
The TryParse pattern is considered best practice of parsing data from a string:
- a TryParse method returns
true
or false
to inform you if it succeeded or not, so you can use it directly in a condition,
- since C# 7 you can declare a variable that will be used as an out
argument inline in an argument list,
- it forces you to check if the out
argument contains valid data afterwards,
Avoid: Convert.ToInt32 — it's a bad choice for parsing an int
. It exists only for backwards compatibility reasons and should be considered last resort. (Note: Convert does contain useful conversion methods: To/FromBase64String
, To/FromHexString
, ToString(X value, int toBase)
, ToX(string? value, int fromBase)
)
Avoid: int.Parse — you have to use a try
/catch
statement to handle invalid input, which is a less clean solution.
Use int.TryParse https://docs.microsoft.com/en-us/dotnet/api/system.int32.tryparse?view=net-5.0#System_Int32_TryParse_System_String_System_Int32__ Int32.TryParse Method (System)
Converts the string representation of a number to its 32-bit signed integer equivalent. A return value indicates whether the operation succeeded.
read the docs on tuple types
much much better, I had to make this before xD
wtf
yeah it came from this
and I was unable to make the comparation with == with an object, so I had to convert it to Int32 first
with
null
isn't necessaryin the meanwhile the compiler keep throwing null warnings xD, which is the severity of those warniings?
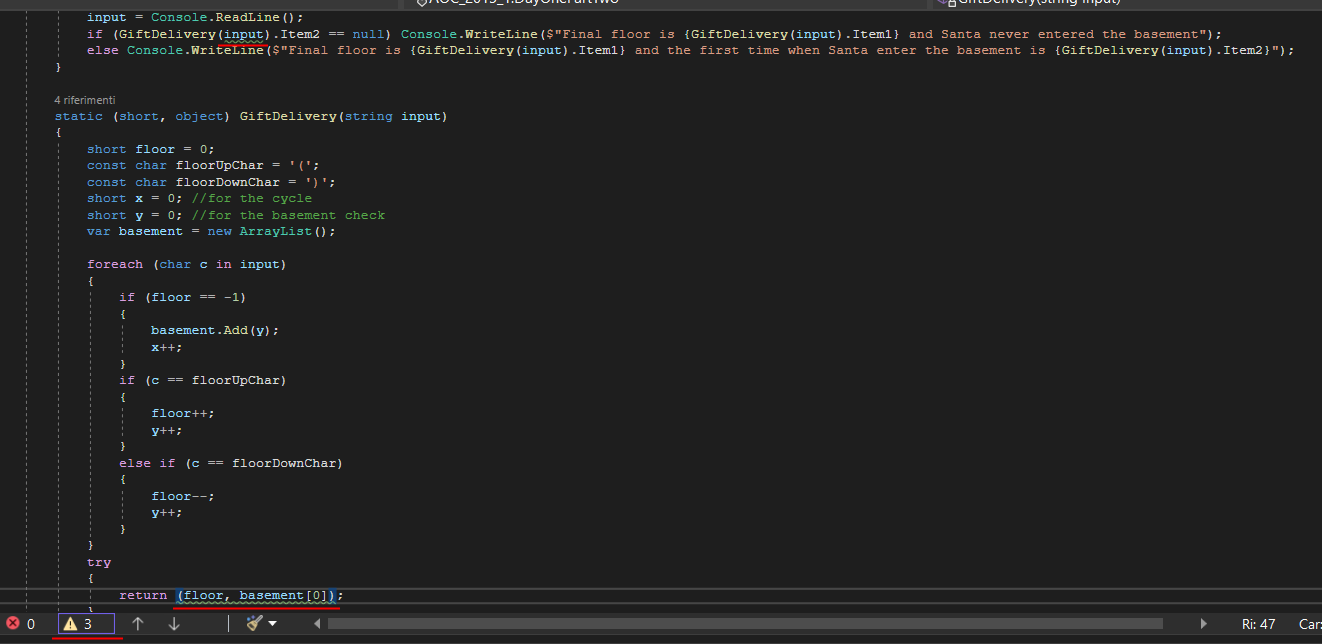
I "solved" all null related warnings by putting a
!
at the end of some parameters, but idk if I made a good thing or is maybe leave them as before and ignore warnings.
the app seems working normal xDobject? are you serious
null forgiving? lol
you've got to be kidding
learn nullability or disable it bro
don't hack it like that
I putted object instead of short due to the ArrayList
ArrayList??? wtf are you doing?
it's not the year 2000
List<string>
is what you want
ArrayList
ignores the tyoe systemI used it to intercept at which position the floor had value -1 for the first time
I started with an array, then I replaced it with an arraylist for improve the code, but now I'm assuming it's quite old
what about this?
null forgiving is what I made putting the
!
everywhere ?or List<whatever>
yes
that's the null forgiving operator
oh, I thought I was solving problems with it, so it's better I remove them ignoring the warnings
bruh
either learn how nullability works
or disable the nullability entirely
ok
like already mentioned in the code review the other day, nullability warnings are there for you to handle them
not to ignore them
what about in the var declarations?
I declared a var using
putting the
?
at the end, I seen expert coders making this for remove the warning
all code below the if block will now know that
input
is not null
because it can't be
because you already handled the case where it is nulloh ok now I und, seems to be an easy concept
the compiler just warn me that I didn't handled the nullability
Can I retain this valid for C#?
Standing to this description, the List doesn't allow to add data dynamically, that's why I used ArrayList before on my program.
I made some searches of other collections, but I didn't found anything that allow me do create a dynamic array.
Stack and Queue could works for this job maybe.
Btw I'll continue with my exercises for learn new things, and I think that I'll going to try learn Interfaces soon.
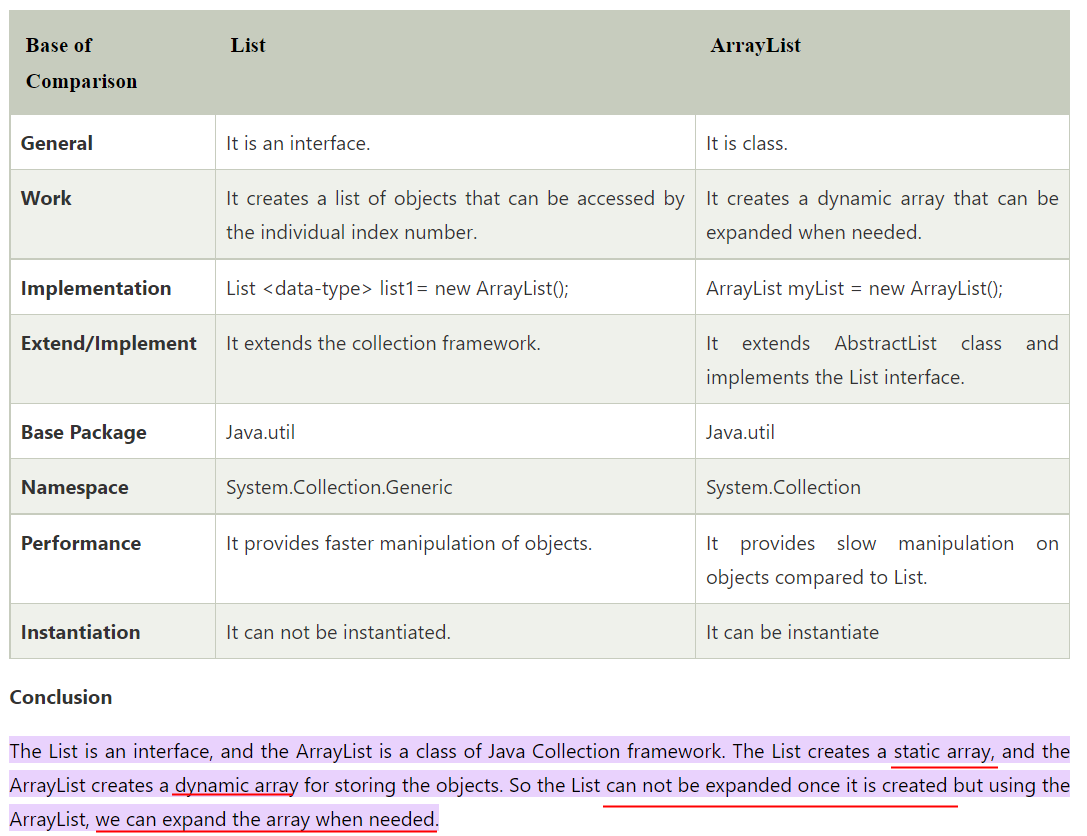
this is bogus
what the fuck
this is Java!
ik
so what are you doing reading this!
is the only comparison table I found on the web, for this I asked if is valid also for C# 🥲
i mean you have to specify that it's talking about java in the first place
yeah my bad
no, this is not at all true for c#
Ero#1111
REPL Result: Success
Result: List<int>
Compile: 443.184ms | Execution: 25.501ms | React with ❌ to remove this embed.
works really good^^
in this case I suppose that I should put a random number instead of null, since it's a valid value for numbers types
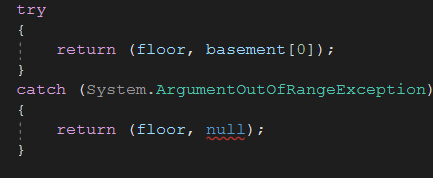
You shouldn't use try catch first of all
I put it for handle the empty case, the program crash normally
So handle the empty case
Check if the list is empty or not
why is better to use an if statement instead of a try catch?
Way more performant, way more readable, way less prone to other issues
oh, ok, I thought it was more accurate for the purpose
what makes you say that?
how much more accurate than
if (basement.Count != 0)
can you get?I thought having the exact name of the exception and using a dedicated statement would be better
normally I would have declared the list using the second sample.
is the first one a sort of abbreviation for creating new instances?

yes
it should be almost done.
for some reason I'm trying to figure out, this get executed three times
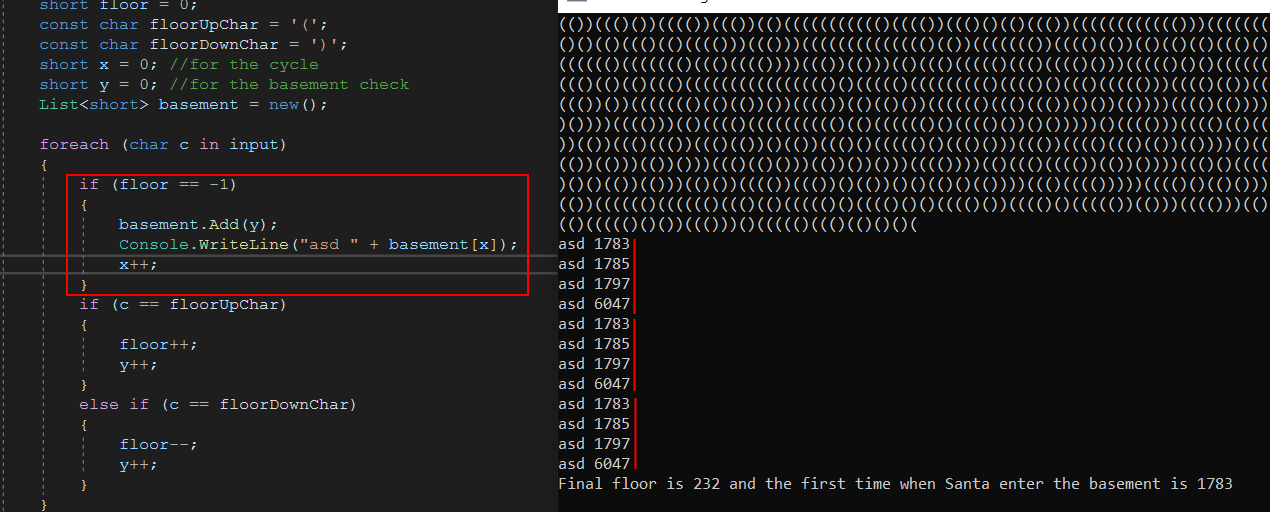
I'm looking for var values and for some problem seems related to the whole foreach cycle that restart after all
c in input
are iteratedI am going to throw up
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.