✅ Not able to locate web api controllers in a class library
Hello,
I'm having some issues with trying to load web api controllers from a class library. I did try explicitly adding the assembly with
AddControllers().AddApplicationPart
, but no luck there. I'm thinking it has to do with the pattern I'm using for registering services in separate assemblies.32 Replies
This is what my main project startup looks like:
This is the extension pattern I'm using to piggy back off the startup of the main project inside of the class library
Here's the output I get when making a request:
minimal reproduction repo if anyone takes a look: https://github.com/RyanCallahan312/multiple-projects-with-web-api-controllers
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
I understand the issues with magic string constants, but I don't think thats relevant to the question. The repo this issue is actually happening uses options configs. I just didn't want to copy over all the configuration
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
unless im completely misunderstanding and thats preventing controllers from being discovered
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
thats why i included the repo because i realized it was too much for just copy and pasting files
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
its in the same assembly as the extention
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
No i did not. what does
...
do?Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
oh then yes I did specify the controller class
same result and same assembly
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
i think i accidentally added that when adding
<PackageReference Include="Swashbuckle.AspNetCore" Version="6.5.0" />
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
yep thats pretty much what I have in the private repo.
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
The motivating factor for me right now is transitioning away from a ruby world that has bit me in the ass with convoluted "god objects" or "god models" too many times
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
appsettings.json:
src/Foo/FooOptions.cs:
src/Foo/FooServiceCollectionExtensions.cs:
Program.cs / Startup.cs:
Bar.cs:
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
I updated the project but still same behavior. Still a bit new to anything outside of basic one project crud apps so how would FrameworkReference not being defined break things?
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
tebeco#0205
<FrameworkReference Include="Microsoft.AspNetCore.App" />
Quoted by
<@!689473681302224947> from #Which dll do I need to load for Microsoft.AspNetCore.Builder WebApplication Class to be loaded? (click here)
React with ❌ to remove this embed.
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
Adding the framework reference didn't fix it. I'm trying to find a way to list out all registered controllers to see for sure they're not registered
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
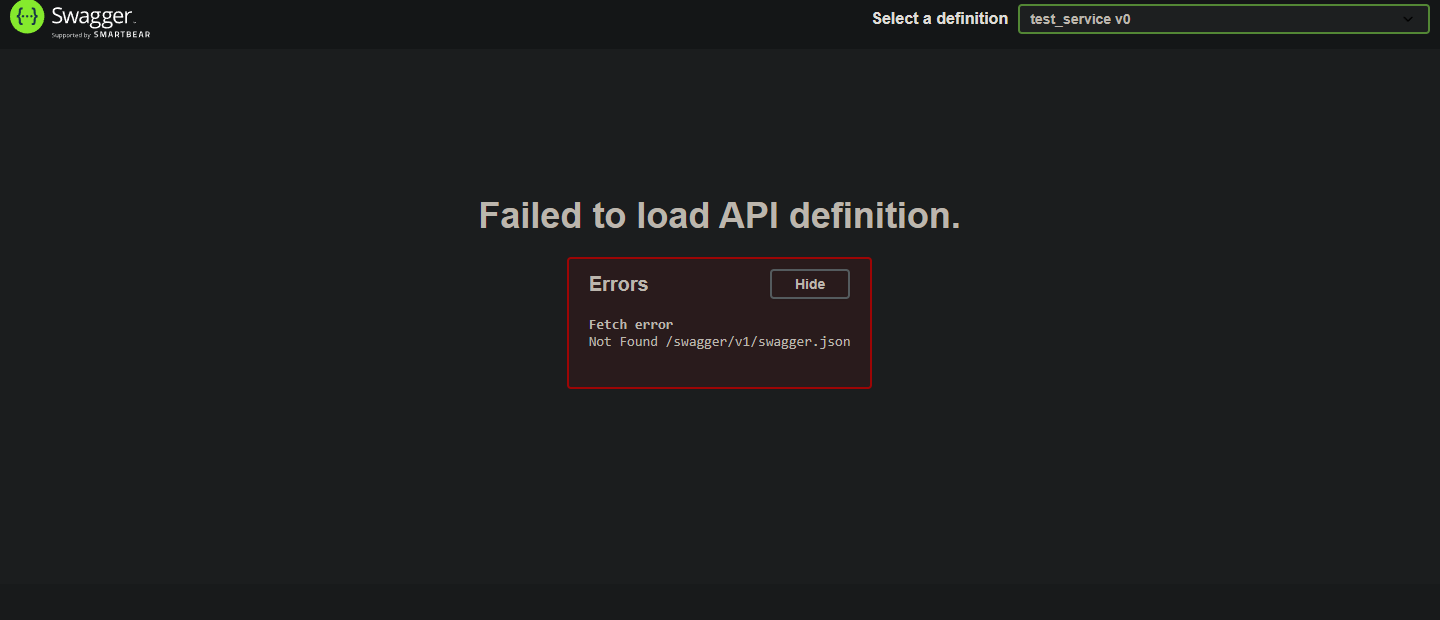
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
tebeco#0205
TLDR:
if you open a term and
dotnet new webapi -n PleaseDeleteMeLater
you'll have an example to re-use and delete laterQuoted by
<@!689473681302224947> from #Not able to locate web api controllers in a class library (click here)
React with ❌ to remove this embed.
so that worked...
I added a project reference to the LibApi and it found the controller without going into the extension method
Solved. I think it was
<FrameworkReference Include="Microsoft.AspNetCore.App" />
but the secondary issue of me not including the rest port in the appsettings.json didn't show it working once that was fixed. Guess thats what I get for laziness and not copying over the option configs. ty @TeBeConsulting