❔ (Very beginner)
Guys, I've got a class TvProgram(The program it self), and a class TvWeek(the programs in the week), the class TvWeek consists of a maximum of 100 programs, now I need to make a method that adds a program to the week and adds the amount of the current amount of programs in that week by 1. How do I do that?
46 Replies
Use a list instead
We didn't learn lists yet
I need to do it somehow without
Well arrays are fixed size
Yes
It doesn't need to go beyond 100
Ok. So what's wrong with what you have?
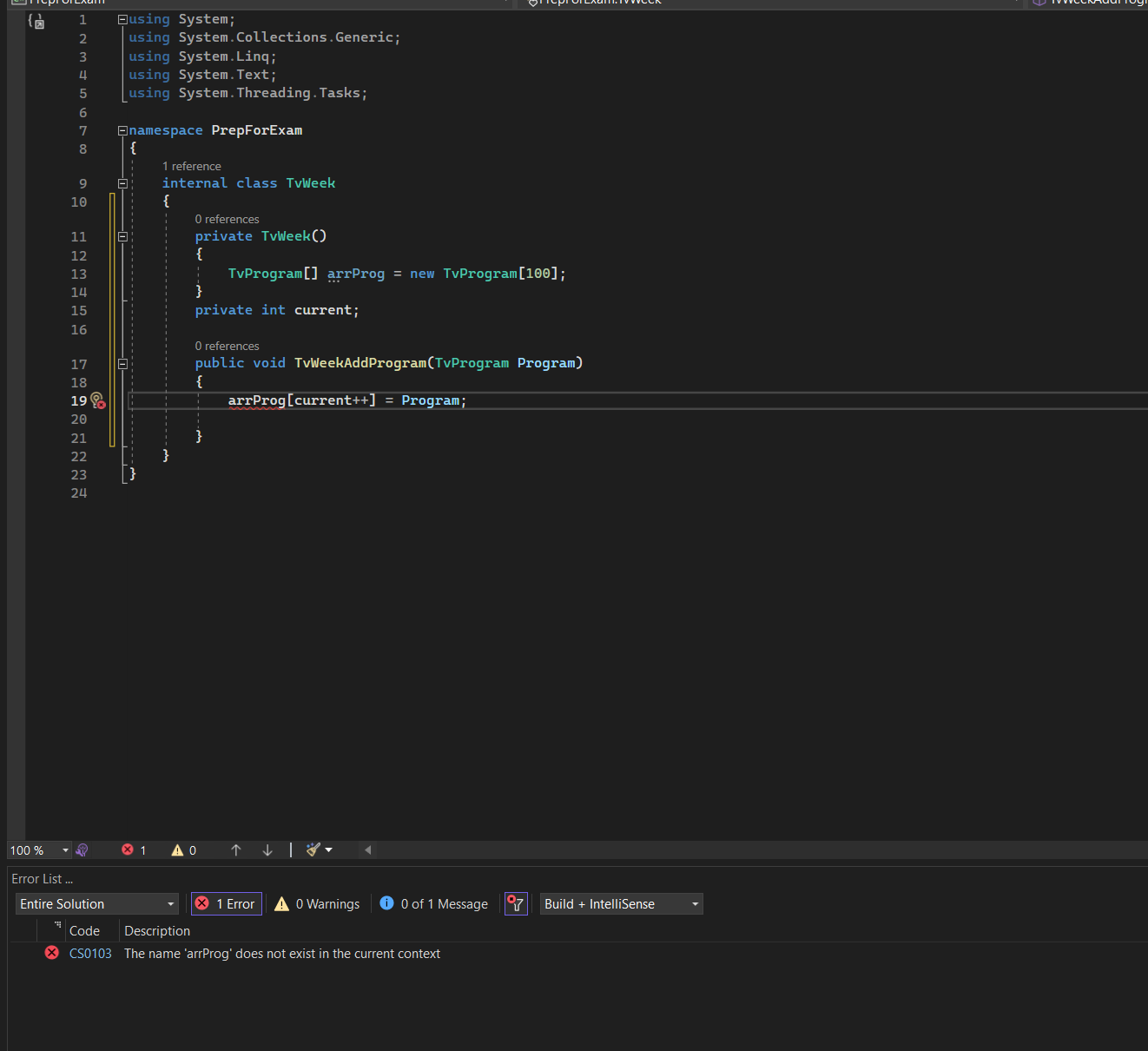
it doesn't see the arrProg from the TvWeek
Paste text not images please
Compiler Error CS0103 - The name 'identifier' does not exist in the current context
Why do you think that is? Where did you define it?
I defined it in the class
Nope
Kinda, but nope
xd, if I define it in another method it won't see it in another?
Even tho it's in the same class?
Correct
mhmm, so It's only possible to do this question by making a new array in the new method?
No
Because it feels kinda strange
Look how you defined current
Yes
I don't get it
What's the difference between that and your array?
Good question
You can't see any difference?
I can see a difference
I defined the TvWeek
and didn't the " current"
You did
I didn't encounter questions like this with arrays before
So I have hard time understand what's wrong with how I defined the TvWeek
It's nothing to do with arrays
It's basic c# syntax
Where is current defined?
in " internal class TvWeek "
Right. In the class body
Notice the compiler is not complaining about the use of current in your method
ohh
$code
To post C# code type the following:
```cs
// code here
```
Get an example by typing
$codegif
in chat
If your code is too long, post it to: https://paste.mod.gg/
but they say, that TvWeek has two parameters - array in a length of 100 TvPrograms and current in int
Is that considered a parameter of the class TvWeek?
Parameters are inputs to methods (including constructors)
So that's not a parameter
You know how can I make it a parameter and use it in the class?
Look up constructors
Yes
You pass in arguments, then assign the value to class fields
I do this, and then I send array of 100 programs?
Yes
And save the value to a field
Can you please explain that?
Ie you still need arrProg
Oh, so define this array as arrProg in the constructor?
No
Probably best if you look up constructors
I will check it, but I did work with constructors, just not arrays
Again, your questions are not any difficulty to do with arrays
You right
Ohhh, I see now
I had to write it that way, thank you for your time!
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.