❔ ✅ AES - Padding is invalid and cannot be removed
I am attempting to quickly encrypt a set of files using AES, but I'm getting the above error when decrypting.
I'm not sure if it's encryption or decryption that's wrong, but I've tried and googled everything I can think of, but nothing seems to works.
Can anyone tell me what's wrong with this code:
(also tried reading one-byte at a time, but with the same results)
17 Replies
did you just share your hardcoded encryption key?
That's the IV I think
oh okay
I don't aes
@Arch Leaders what are you trying to make with this? from our perspective it sure looks like ransomware
Although you should probably use
aes.IV
and not something hardcoded
Anyway...Just personal encryption of files and some practice for a security model I'm working on elsewhere.
Didn't really understand it, so I just put 16 random bytes there xD
Here's the entire project if it helps with that concern with my super secret key totally visible this time
I can also plop it on github if need be, just want to solve this weird issue.
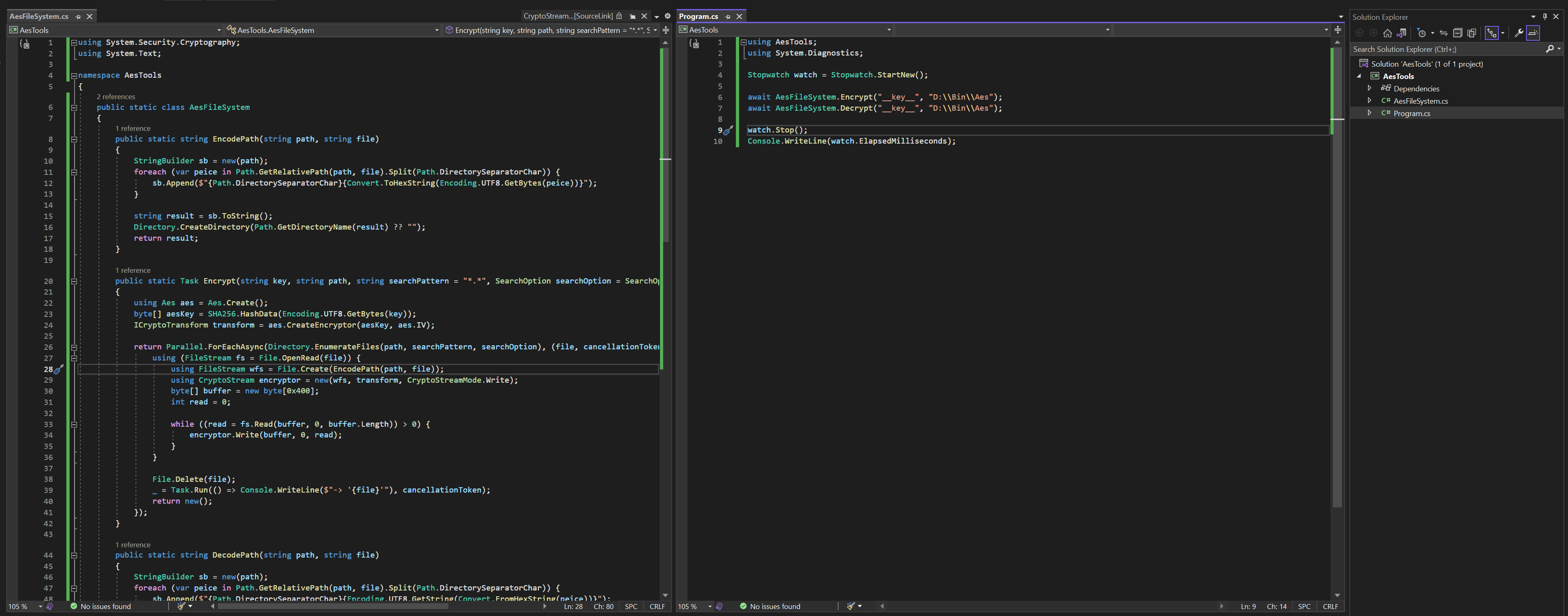
@Arch Leaders you are hitting that error because the code is not thread safe. You can't share the ICryptoTransform across multiple threads concurrently. Give each parallel task it's own instance of ICryptoTransform and it should fix it.
Also just as an aside, if you want to do this more "correctly" the right thing to do is to store the IV along with the ciphertext and then calculate an HMAC on the combined ciphertext + IV and then store that as well.
Then validate the HMAC on decrypt.
Ah splendid, thanks!!
Ah good to know thanks, I'll look into that 👍
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
lol
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
Because AES and RSA are not even remotely the same thing
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
You would never use RSA for encrypting arbitrary files so I doubt it's relevant for the OP scenario
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.