❔ GPIO listening to button events (Raspbery Pi w breakout board)
Im using
GpioController
to control the GPIO pins on my Raspberry pi, i have a breakout board with some LEDs on it and a couple of buttons.
Controlling the leds has been pretty easy so far, as they are not something I have to actively listen to but I'm trying to make a method that will listen to all connected buttons (there are total of 6 of them) and do something based on when they are pressed (For testing im simply trying to print to console with their respective button number)
I've tried a couple of things, but Im having issues in their behavior (you press once, it keeps going off for eternity) and in general I think I'm doing it in a convoluted way.
Does anyone know the best method to listen for button presses (on the background) and then upon button press allow them to execute a piece of code?
the extent of what I have tried so far mainly revolves around opening all pins that are buttons
I have no idea if this is the right approach and any pointers would be greatly appreciated38 Replies
Oh and the _controller is just an instance of gpiocontroller
GpioController _controller = new GpioController();
Are you sure you've set the pull-ups/pull-downs on the button inputs as appropriate? Or do you have external pull-up/pull-down resistors?
They are setup yeah
Where? Are they external? Your code doesn't enable the internal pull-ups/pull-downs?
Its a breakout board that is wired to another one that has resistors on them
It functions properly in a python project it came with, not sure how they handle it
I'd also avoid calling
OpenPin
on every loop iteration
Also try specifying the PinMode
when you call OpenPin
?Good points
Is there a more elegant way to open all these pins in 1 go?
Also, what's the logic in
// Logic here to listen to them
? Maybe that has a problem
Doesn't look like it, from the docs
Although I'm not familiar with GpioController
itselfThey are are a bunch of tasks that refer to a corresponding method based on the pin number
This is pin 4 for example, mainly trying to get one button working properly before moving on to all
Which after pressing the button once results in this

Wait, that means the pin is constantly rising/falling, rather than just being stuck low?
It seems so, but only after the initial press
If i do rising, it will always be going off
Ill give it a quick try though with pinmode as input
Ah, d'oh
There's no code to re-read
waitResult
in there
So yeah, of course it keeps printing the waitResult
-- because it's Falling
when you enter that loop, and it's never re-assigned so it never becomes something other than Falling
ahh
Also don't use
Thread.Sleep
in async code. If you need to delay, use await Task.Delay(...)
Alright, ill give it a quick test, ill reassign
waitResult.EvenTypes
to rising and ive set the pin type to input
Yeah that stopped it from repeating
I think i should flip them actually, i only picked falling because it was spamming on risingWhat's the point of that loop anyway? Just print all edges?
Im not sure what you mean with print all edges
What are you trying to achieve with that loop?
But my basic goal is to have a press of 'button 1' result in 'Button 1 was pressed' to show up in console (I mean later there will be logic tied to what it needs to execute but thats not important for now)
And only do that once, for every time i press a button
It sort of works now i think, it just prints it out 4 times each time i press it assuming I want the 500ms task delay i put in
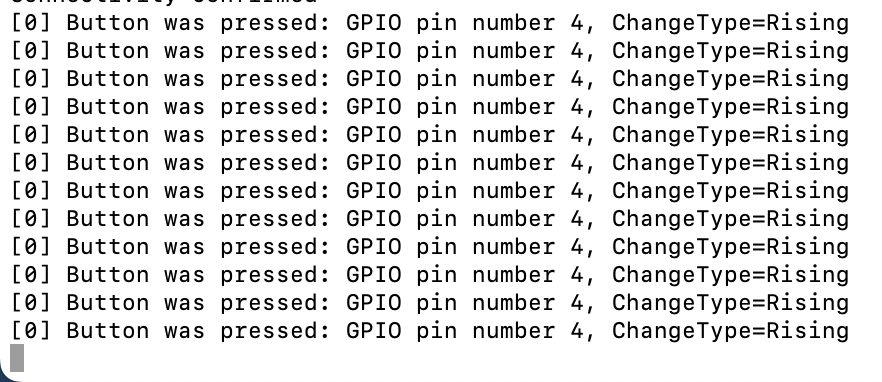
Ah, so:
?
I will say this current code is the result of just throwing shit at the wall and seeing what sticks, i think i overcomplicated it for no reason
Yeah
This is what i have now, made it a bit shorter since that Console.WriteLine doesnt need to be very detailed anyway
That
do...while
and waitResult.EventTypes = PinEventTypes.Rising;
just cancel each other out
It means you'll never loop around that loop more than once
And once you strip that out, it starts looking like my codethats true
Oh, unless it's TimedOut, but that won't happen anyway? And besides you've got the outer loop for that
Yeah good point
Im gonna try yours now, one slight edit because I just did the order wrong for Rising and Falling; it was spamming falling over and over until i would press and hold the button
Also it's racey: if you get an edge after
WaitForEventAsync
returns before you call it again, you'll miss that eventYeah, im not sure how to best approach that
ideally it wouldnt matter how quickly you spam the button
GpioController.RegisterCallbackForPinValueChangedEvent Method (Syst...
Adds a callback that will be invoked when pinNumber has an event of type eventType.
Could you give me pointers on how to implement that? I'm not really certain how to do it
if not thats fine too, I think this was in one of my open tabs but i couldnt figure it out or find a guide online
Looking at the docs,
Or whatever
PinEventTypes
you care about -- PinEventTypes.Rising | PinEventTypes.Falling
for bothAlright, thanks!
Ill give this a try, thanks for all the help Canton
Sweet! No worries
Also fun little problem, i noticed if i hover my finger like a half an inch / few mm above the button it is already activating lol
Hah
That strongly suggests that you don't have those pull-up resistors working right?
Yep, I think something got disconnected
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.