Shifting a List(int) to become 1,2,3,4,5...
Hi, If I have a List like this:
2,3,4,5
And I would like for it to become:
1,2,3,4,4
This should be done by taking from the higher numbers in the list shifting them to the left so they come in order like above, how would I do that?54 Replies
is the second 4 in what you would like a typo?
this doesnt look like a shift but more like you are just decreasing everything by one
No, its not a typo
I need to reformat the List to be from 1->2->3 etc. but if they have the same value at the end it won't be a problem
The 5 in the original list, removes 1 from itself, and becomes the 1, and then there is 4 left in the end,
Idk if it makes sense
Can you share an actual example, which is correct?
Not sure what you mean?
That is the example
I don't understand, if its not a typo, and I wan't it, why would I remove it?
Scrap that, I can't read
I still don't understand how 2,3,4,5 became 1,2,3,4,4 though
2,3,4,5
:
2
stays the same
3
stays the same
4
stays the same
5
lends 1 to the beginning of the list, so it becomes 4
, and the begging now has a 1
1,2,3,4,4
So you want to take the last item in a list and subtract 1 from it? And you also want to add 1 to the start of the list?
Which of those two bits are you having trouble with?
Its situational
I wanna input
N
(number of items in the list)
N, N, N...
N amount of items in the list that needs to be sorted this wayCan you give a full example? I don't follow what
N, N, N...
means4
2,4,6,8
Another example ^^
N is just a whole numberOK, so that becomes
1,2,4,6,7
?
(Presumably not, because that's too simple, but you're not giving me enough information to have a better guess at what's going on...)That sohuld become
1,2,3,4,5,5
How did
2,4,6,8
become 1,2,3,4,5,5
?Thats the thing im trying to figure out
Uh...
what are the rules?
everything above 5 get added to beginning?
Its in danish, I can't explain it well
??translate en Dette er en eksempelsætning.
bot dead?
It wont work like that
Let me try and translate it, so it makes sense
Just to quickly clarify:
n
= whole number
i
= whole number
a
= whole number
Some beavers wanna build a new damn, but they wanna sort their sticks before starting to build, they have n
amount of stickpiles, the beavers have counted the piles and written it down in the order that the i
'th pile has a<i>
amount of sticks. The beaver engineer says it will be easier if they sort the piles so one pile has 1 stick, the next has 2, the next has 3 and so on. The beaver engineer needs help to know how many sticks that maximally can be used.
Example:
If the beavers have the following piles with sticks {2,5,4,3}
, the beavers can move them around and get the following piles: {1,2,3,4,4}
, in this example the answer would then be 1+2+3+4=10
, since these are the sticks that makes up the piles that the beaver engineer have asked for.
Input:
1:
4
2:
2 4 5 3
Output expected:
1:
10Right, so you want to sum all of the values in your input array, and then construct an array 1,2,3,4,... such that the sum of the second array is as large as it can be, while also being <= the sum of the input array
Yes
I have the following for now, but im not sure where to go from now:
This works for the 2,4,5,3 example, but not for the 2,4,6,8
I think you're making this more complex than it is
I might be lol
The question talks about "sort the piles" but I think all you need to do is to put all of the sticks in single big pile, and then draw sticks from the big pile to form a pile of 1 stick, then a pile of 2 sticks, etc
Until your big pile runs out of sticks
So I would just add all the sticks from the original togehter, and then just start adding them until it runs out
Yeah
Or it becomes longer than the original input
no it can get quite much longer than original input
eg 1 2 9
which would be
1 2 3 4 (2)
solution would be 10 again tho
if i understood that correctly
Is there an easy wat to sum all of the value of a list?
I mean the first input
.Sum()
, or just use a loop and sum += element
inside the looperror CS1061: 'List<int>' does not contain a definition for 'Sum' and no accessible extension method 'Sum' accepting a first argument of type 'List<int>' could be found (are you missing a using directive or an assembly reference?)
I'm using using System.Collections.Generic;
Nvm i needed Linq
Hm, it completes the first task, but it errors on someo f the othercode + input + actual output + expected output would be helpful
I don't know, its hidden
Thats the thing
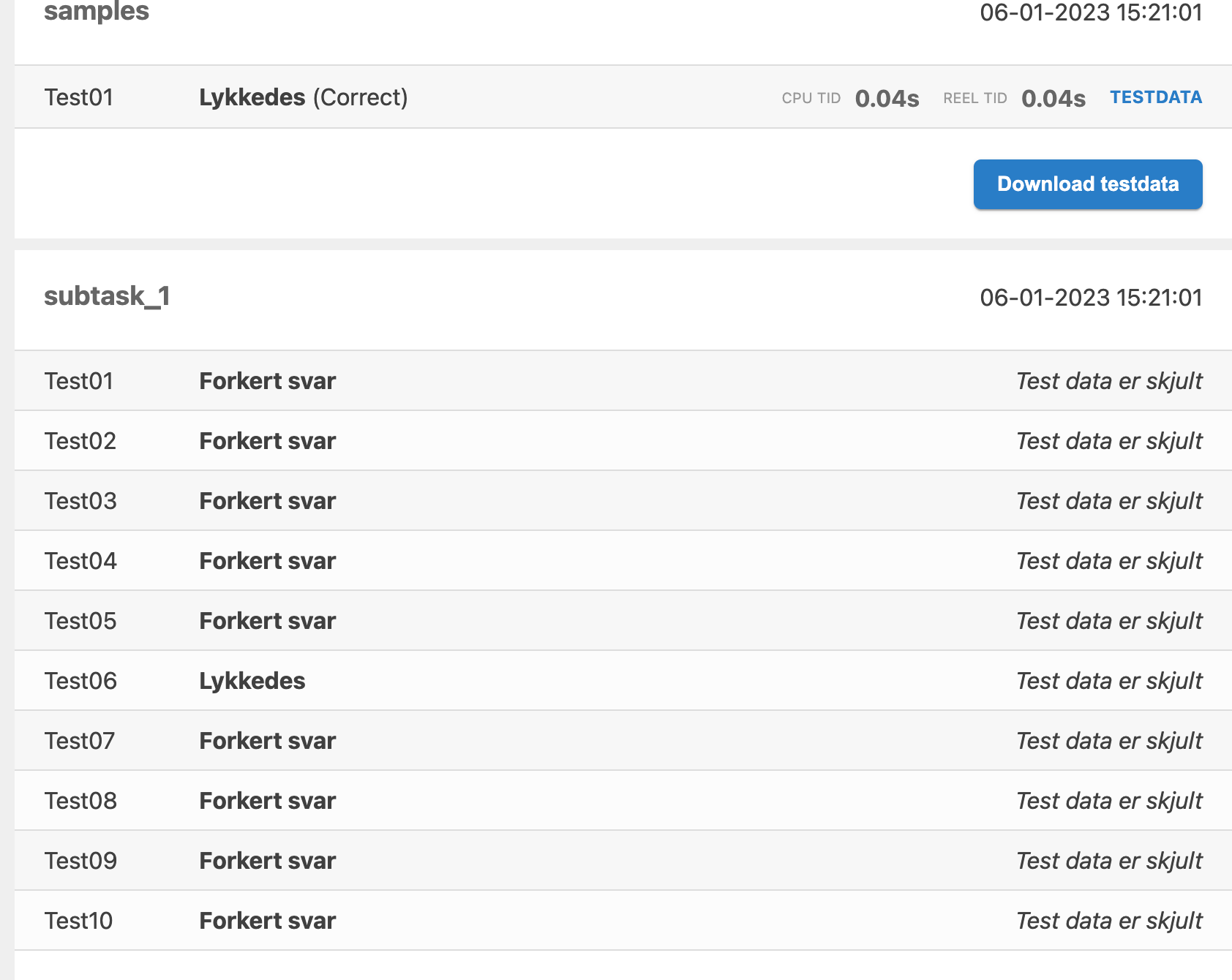
Forkert = wrong
Lykkedes = completed
Test data er skjult = Test data is hidden
The first one in the top is the example with 2 5 4 3
well show some code
All we know is that some code you've written, which we can't see, is wrong
for (int i = 0; i < BunkerAmount; i ++)
-- why stop at BunkerAmount
?Since it's what it does in the example
I think
Where in the example?
The question says to sort all of you sticks into piles, until you can't create any more piles
1,2,3,4,4
-> 1+2+3+4 = 10
- wherei sth elast 4?Not to stop when you reach some arbitrary number of piles
it cant fill the last stack so gets discarded
You don't have enough sticks to make a pile with 5 sticks in, so you stop
Oh
But what would I then stop at
if
i < ??
...when you can't create any more piles
when rest of stick smaller then size of pile should be
So if the next pile needs 5 sticks, and you have < 5 sticks in
TotalGreneAmount
, then you stopNot sure how I would do that
You're not sure how to compare two numbers?
No lol
What should i have in my while loop
I'm not going to tell you the answers
I think if you start
i
at 1
rather than 0
, you won't have all of those i+1
's, and that'll make things easierI figured it out, thanks! And completed all tasks
ty