✅ Code highlighting issue in Rider with Int128 and UInt128 types
Putting the new 128-bit integer classes to use which implement ISignedNumber and IUnsignedNumber. Both of those interfaces implement INumberBase and that implements IAdditionOperators. Here is the code of both UInt128 and Int128 with their operator + implementations:
How come Rider keeps telling me these types are incapable of any sort of operation? The code runs fine but is there a way to fix this inconvenience? Or at the least, disable the check here?
I have already changed all projects to target .NET 7.0 and the solution's .NET SDK is 7.0. I also invalidated the caches
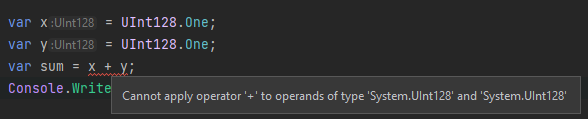
5 Replies
known bug, is expected to be fixed in the next version
Rider is a little slow to support new .NET versions
also it's apparently a regression exclusive to 2022.3.1 so reverting to 2022.3 might fix it if you want to do that.
https://youtrack.jetbrains.com/issue/RIDER-87010/Cannot-apply-operator-to-operands-of-type-System.UInt128-and-int
Just curious, what's your use case for 128 bit integers?
Many of the numbers in my program are larger than longs but no larger than long longs so I'm just avoiding the use of BigInteger
But just playing around with new toys that came in .NET 7 really
Those...are some big numbers 🙂
For real 😂