❔ My code cant write to a certain path
Basically i have a script to download a file from github into a specific folder and when i click the button that runs the code it tells me it doesnt have access to the path.
Code.
Using framework 4.8, c# visual form project
Any help is appreciated <3
11 Replies
Is
test
the folder or the filename? If it's the folder, you should add the filename on WriteAllBytes' path variable. If it's actually the filename despite the lacking extension, check if there's not a folder of the same name.Test is the name of the folder that i want to download the github file into
Then you need to add an actual filename to
filePath
. You could use Path.GetFileName
on the fileUrl and paste it onto the filePath if you want it to have the same name.how would i do that?
im a beginner
but the actual file name is Test
Is it the file name or the folder? You just said it's both.
The name test is the name of the folder
the file name im getting from github is VEH_PDSVK0B_AT.BIN
Right. So you get that filename by using Path.GetFileName(), and then past that onto the end of your location.
WriteAllBytes need a full path plus the filename. It doesn't just guess what the file is named.
So what would the code look like then? its hard for me to understand without examples sorry 😅
I'm not sure what the rules are here about spoonfeeding, but I'm not going to write your code for you, sorry. What you need was explained in my second and fourth message.
oh
it worked
thank you
for your time and hassle 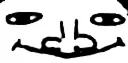
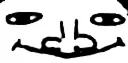
Looks like nothing has happened here. I will mark this as stale and this post will be archived until there is new activity.