63 Replies
why do i have to pass by reference array that I am going to change?
arrays are reference type value, what is the point of passing them by reference?
to make
baz = quux;
work
you mean will print 1 and 2 because data itself was modified?
yes
(last comment)
but why isnt it modified
by
data
i mean the local variable in Main()
was modified, because it is a reference (like a pointer) to itshouldnt method without reference point to the same memory as array is in
in
Example1
array
is just a copy of the reference stored in data
ooooohhhh
yeah, but with
baz = quux
u basically let it point to other memorybut
how does it make difference
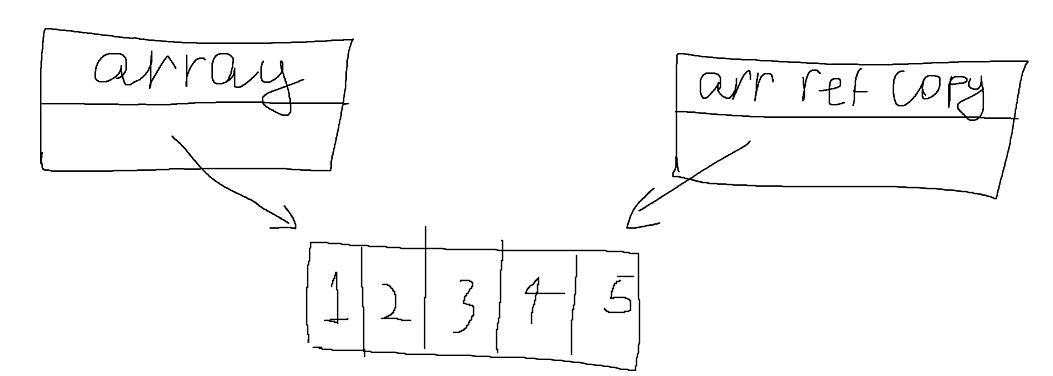
imagine third box as copy of reference that was created by method
hmm, lets take it with
int
instead of int[]
:
but int is value type, not reference type
this one will change
but a reference itself is something similar to a value type
when
Example1
will be called, another stack frame will be created, which introduces the parameter x
,
then the value of value
will be copied to x
(in this case 0
),
then the method will be executed and x
will be 1
,
then the method will be left and the stack frame will be discarded (x
and its modification is lost)yes
when
Example2
will be called its a bit different:
a stack frame will be created, which introduces a pointer to an int
will be introduced (thats more or less x
)
then the pointer of value
(from Main()
) will be copied to that x
the the method will execute and the memory x
is pointing to will be modified (thats value
on the stack frame of Main()
)
the method will be left, the stack frame is gone and thus the copy of the pointer of value
, but its modification not, because its on the stack frame of Main()
in c it would look like
if thats more understandable for uyeah, i totally understand this
but its for the value type
so now comming back for reference types:
ref int[]
isn't a reference to the array itselfint[] value;
doesnt store the array, but a pointer to a memory block on the heapit's a reference to the local variable in your method
if u would just modify the elements of the array, it wouldnt matter if its a
int[]
or ref int[]
parameter, because u just modify the memory on the heap.
but as u want to assign it to a new memory block baz = quux;
it makes a difference if u just modify the stack frame's local variable
or the pointer/reference itselfoohhhh
missed the fact that quux is new memory block
;p
so, is ur question answered? if yes, please $close the thread
Use the
/close
command to mark a forum thread as answeredwait a sec, thinking it over
wait fucking what
so its like
referencing to a reference?
yes
that's exactly what it is
a
ref int[]
is effectively a int**
+ a lengthWhenever you see a reference type variable in code, you can think of it as a pointer
So string s is a pointer to character data
that why i took the
int
example first, to verify the general understanding ;pint[] is a pointer to int data
wow
Adding a ref modifier is adding a pointer to the signature
So ref int[] is effectively int**
But safer
so i took reference, and dereferenced it to a new chank of memory that i have created?
In which example?
yes
Right, so bar has an effectively int** parameter
wow wowowowowoowowow
and all of the arrays, classes are all same as just pointer to smth?
like int*?
all reference types are
damn
c# is cool
effectively they work like pointers, but because this is managed code its a bit different because the memory manager could move the memory blocks around, thus pointers would be invalid if they would contain "real" addresses
they do contain the real addresses
thank you everyone, thats a big help!
!close
Closed!
yeah but there is more to it because of the memory management i mean, or am i wrong here?
basically what i meant is, that the address can change without every touching that one reference
yeah, the GC can move things around
btw
can i somehow pass the copy of array itself?
copy the array, and pass the copy ;p
Not in one easy action
is this ok?
isnt it just for example
new int[] { 1, 2, 3 }.ToArray()
?Sure.
fastest way is just
array.AsSpan().ToArray()
I thinkProbably
but all of them work fine
Well, it should be pretty close in perf to what was written above, but it's certainly easier to type
ok, thank in advance
just doing .ToArray() on the array gets you the LINQ method, which has a fast path for lists and the like where length is known, but also has to do a type check and multiple vcalls to get there
so ¯\_(ツ)_/¯
well, for optimization just throw a benchmark on all, but i guess the span version would be the fastest
I meant in comparison to the foreach
yeah I know, was just comparing to caps answer