tRPC prisma query type checking.
I'm wondering what the best practice is for type checking a prisma query in a tRPC function. I've been using input validation with zod for passing parameters into the functions but am not sure how to check types for a query which i then want to perform logic onto.
bookingsPerDate: protectedProcedure.query(async ({ ctx }) => {
const bookings = await ctx.prisma.bookings.findMany({});
for (let i = 0; i < bookings.length; i++) {
checkIn = bookings[i].checkIn;
checkOut = bookings[i].checkOut;
/* More Logic */
}
console.log(bookings);
}),
32 Replies
There really isn't a need to type check the response from a query since its comes from a "secure" source. Type checking user input is useful because the user is stupid but your database especially prisma should already be returning the correct type
I see you being shy 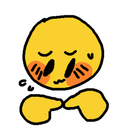
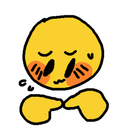
@CFKeef Thanks, that makes sense. TS is still complaining and saying that my bookings object may be undefined.
its possible to do it just serves no purpose
Is your prisma generated?
What does that mean?
So prisma needsto compile the typings for you
run yarn prisma generate
just ran prisma generate
now do ctrl-p restart ts server in your vscode
sometimes ts is lagging behind and you can force the sync
did that, still get the same error...
Could be your tsconfig
My best guess is that its because the findMany can return empty lists?
it should have a union type normally
usually Model | undefined or something like that
this is the type im seeing
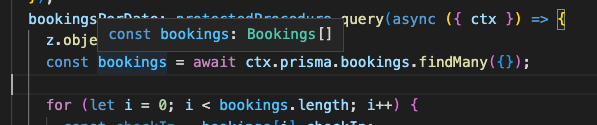
Yeah thats correct
which is my prisma model
Sorry for findOnes its gonna be a union type
A empty array is still technically valid for your bookings[]
Your typing looks correct
This is what i'm seeing
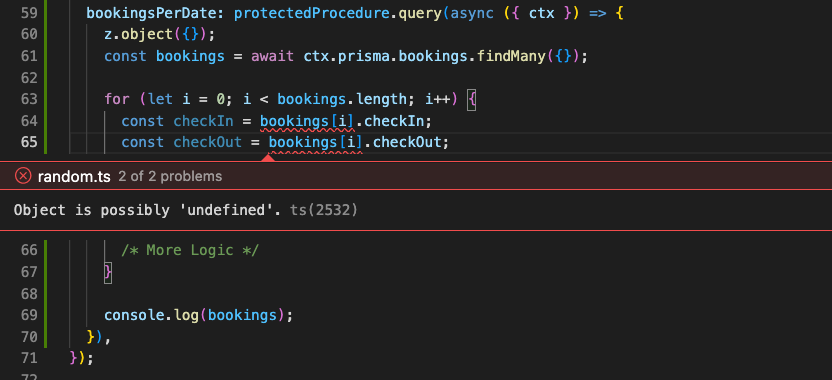
You should just store it in a variable first to avoid having to index for each usage
You can also just bookings.map and that should give you no issues
I think its a ts rule to have to check for existence iwth indexing but this is just typescript so theres alot of ways to skin this cat
thanks for the help, fairly new to TS and the T3 stack
np np
Try it with .map and it should be good to go 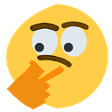
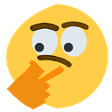
No errors this time 🙂
Strange issue...
It’s gonna be the ts rule probably
But if you are transforming data and want to stick to the newer js apis .map is the way to go
bookings[i] is possibly undefined because you're accessing an arbitrary array index and typescript isn't smart enough to know that it's perfectly reasonable in this case
map and foreach should work
but depends on exact tsconfig setup etc
Yeah I never really use a for loop now a days ty @cje
It’s not able to get the contextual information that a for loop should be able to provide but doesn’t
i ran into this a few times during advent of code where sometimes for loops make the most sense
The for item in/of loop probably should work
you can use non null assertions to "solve" this but feels bad
The for item in array loop doesn’t work?
But maybe that loop isn’t applicable to the use case you had
Hypothetically - how would one do this?
That makes sense
Non null asssertions can be if(!item){…}
Inside those braces item is null
If you stick a return in that brace it’ll type the item as the right typing for code after it
In your case you should store the indexing into a variable then just do a simple if(!booking) continue
For the cases where it exists you’ll have the right typings available for you as long as you shove that work code after the check
But you REALLLY SHOULD just use .map/.forEach depending on if transforming data or just doing something with it
Unless you have to for loop
I’m on my phone rn and lazy so I didn’t do code blocks but I’ll clean it up once I get to my computer
I implemented the .map method - was just curious and wanted to know what was meant with the non null assertions as a learning excercise
Yeah that’s a good reason to
Sometimes people get stuck in their ways so I was just reiterating my point to hopefully convince that someone
haha, yeah people are often pretty bad at learning and accepting new methods