❔ What data structure to use?
Hello! I'm working on a chess game, in particular to a method that gets me the valid moves for that particular piece. I need to return a list of valid moves for that piece, but the problem is, i don't know what data structure to use to return those moves. The method takes in a row and a column, which in fact represent the position the piece is found at. I've been thinking about tuple but im not sure. I also thought about an array, however, i need to initialize to a size, but i can't know ahead of time how many valid moves a piece can have at a given time. Could anyone suggest something? Thanks a lot!
21 Replies
List<Square>
where square is struct that has row and columnUnder the normal circumstances, that would be the obvious approach. But i dont have a square class for this. I'm building the squares in the wpf project. So i have no
Square
class.Are you saying you cannot make custom type?
this is the "square" so to speak... but its in the wpf project, the ui has access to the game engine logic, not viceversa.
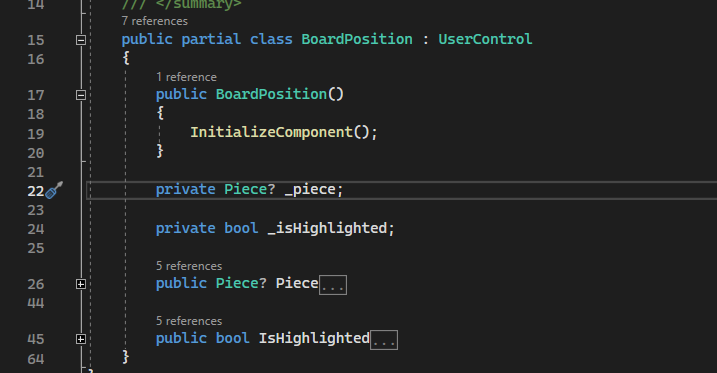
and this is where im creating the board, using the "square"
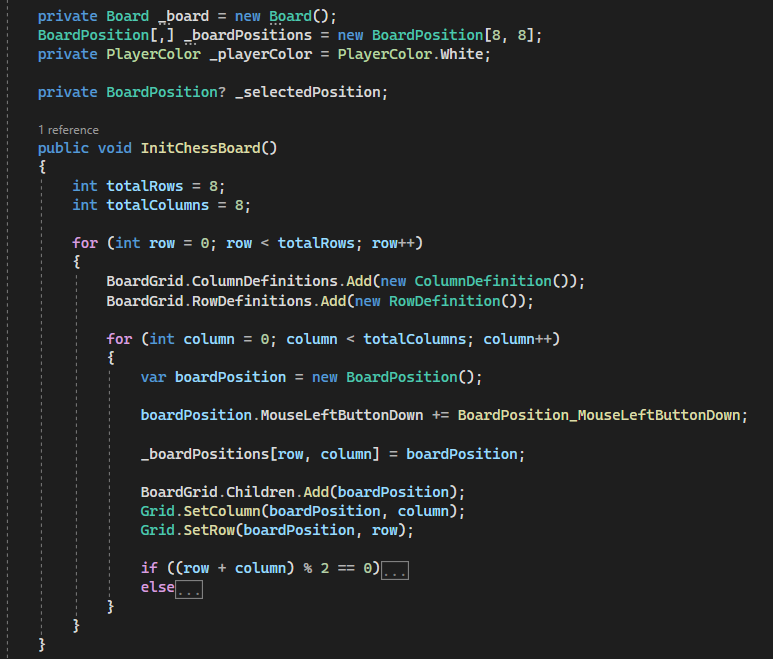
and this is what im attempting to do in the board class
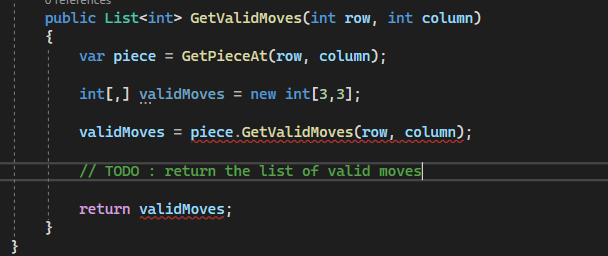
I'm saying make a struct in your game logic. As simple as:
I'm not sure i follow. I'm not exactly familiar with records, never used them before
record is just shorthand to reduce boilerplate code
Are you familiar with struct?
somewhat, yes.. but i haven't been in a situation where i needed them
You could use tuple for your case, but it would be more simple if you have custom struct like
Square
You can use it as easy as:
Okay i guess. Thats one approach.
Is there another i could use? I'd like to have some more options to choose from so i can try them out then decide.
Maybe return an array of rows and cols?
Being tired is not doing me any favors 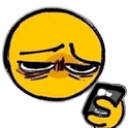
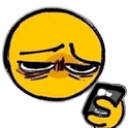
List<(int col, int row)>
is tuple way
Idk why you would use array for it, especially multidimensional one would be horrificbecause i wanna do this myself with minimal help from the outside. Doesnt have to be the most optimal approach right now. I just wanna do something that works. So if it works, it doesn't matter too much. At least not for now.
i mean isn't something like this usually just a
Figurine[,] _board
it is ^
Make sense for board, but not for list of moves
Wdym, where?
Here you are trying to use
int[3,3]
I guess? While the code looks WIPattempted something yes.. i was still confused about how to go about it
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.