Building a design system?
Hi Guys,
I'm taking first steps to build a design system... would love your advice before i go too deep.
I'm using tailwind-styled-components: https://github.com/MathiasGilson/tailwind-styled-component
For my first component, i created a Button, and used it, like so:
src/designsys/Button.tsx
I then use it in my google login button:
It's simple and working nicely. Does anyone have any comments on how to do this better? Thanks!GitHub
GitHub - MathiasGilson/Tailwind-Styled-Component: Create Tailwind C...
Create Tailwind CSS React components like styled components with class names on multiple lines and conditional class rendering - GitHub - MathiasGilson/Tailwind-Styled-Component: Create Tailwind CS...
88 Replies
i think cva is better for creating design systems with tailwind css. The other way is using a design system focus library like vanilla extract or stitches. https://github.com/joe-bell/cva
GitHub
GitHub - joe-bell/cva: Class Variance Authority
Class Variance Authority. Contribute to joe-bell/cva development by creating an account on GitHub.
Why did you decide to go with styled-components?
Not sure I'd recommend that, especially since you're just starting out and can choose something else that's more performant (i.e. vanilla extract like mentioned above).
In Spacedrive we use that same styled components approach using a custom, more lightweight version of the
tw
function, but only for basic elements that don't need multiple variants. For more complex styles with multiple options class variance authority is definitely your friend.ooo a lot to think about guys thanks
I started with styled components cause I've used it for years
For example our Tabs ui is just a restyled version of Radix UI, but our Dropdown ui is done with CVA and is more complex
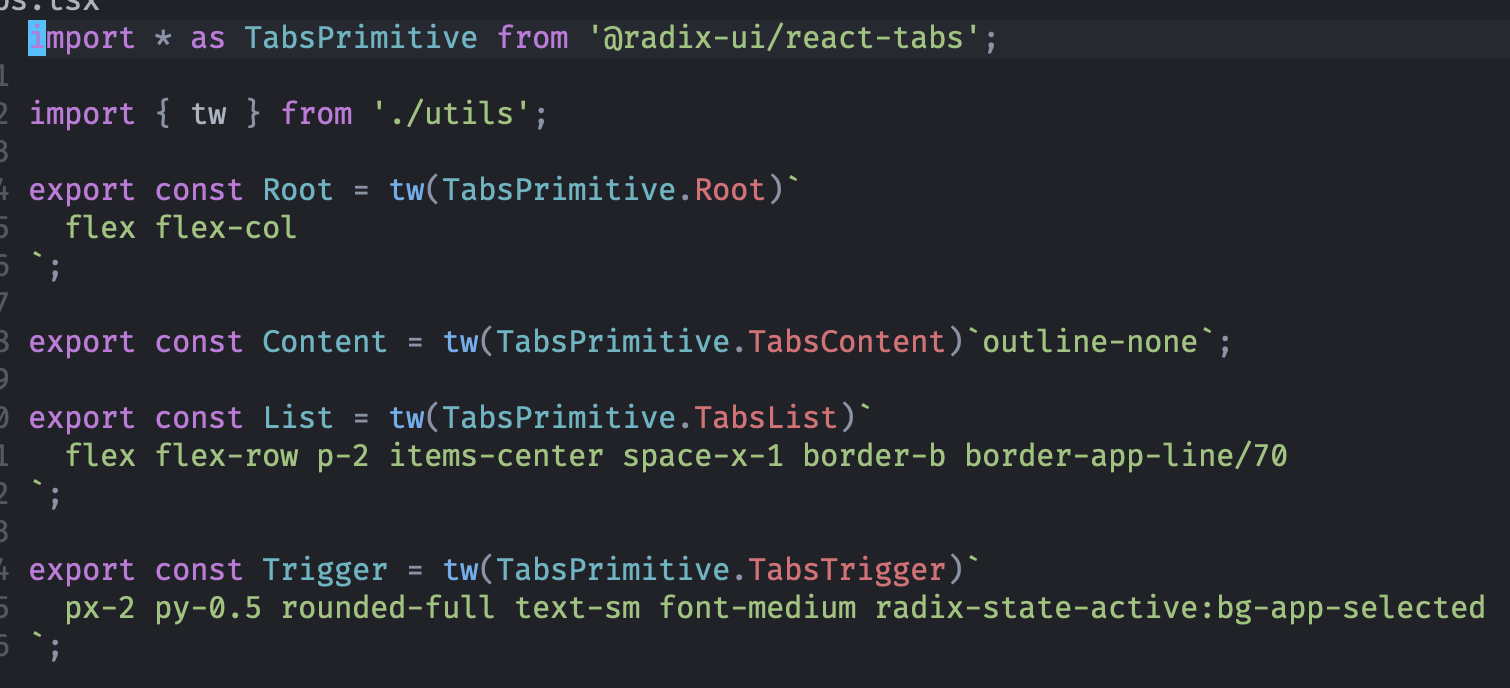
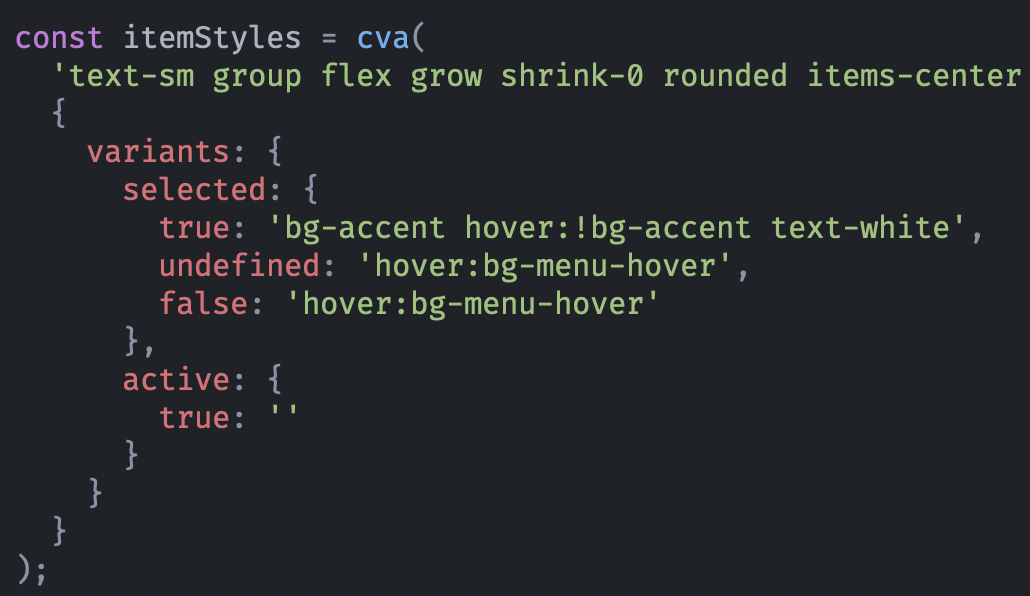
im also familiar with passing variables in and building themes
with cva, can you pass props in and make themes @Brendonovich ?
https://vanilla-extract.style/ looks like you clearly can
vanilla-extract
vanilla-extract — Zero-runtime Stylesheets-in-TypeScript.
Zero-runtime Stylesheets-in-TypeScript.
CVA just gives you a function that returns styles and provides Typescript types that match your variants. You pass your props to that function and then apply the styles to your elements.
vanilla extract is CVA but for actual css and not tailwind
is more than that
Here's out Input component, notice how we pass props to
inputStyles
and the return value is used as class names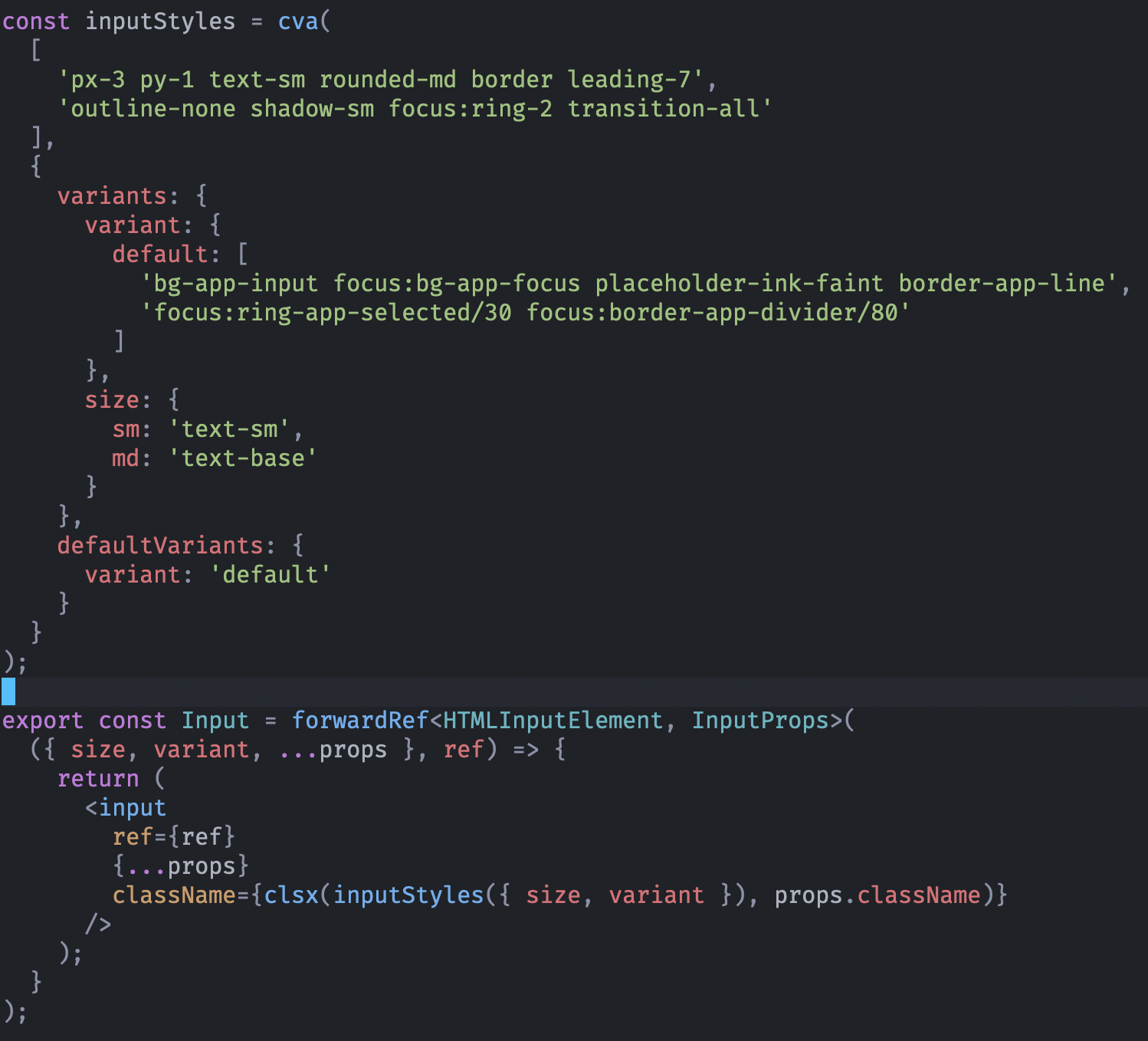
and is for css modules, not pure css
this is so clean
ehh same thing
though it handles themeing too right?

this looks a little scary
lol
it's just building a string
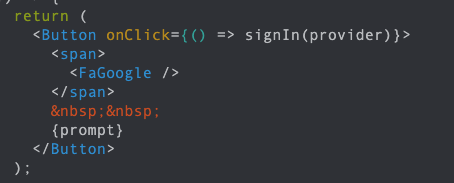
Oh dw about
clsx
and props.className
inputStyles
just returns a stringso builds these class strings and typechecks on tailwind

it typechecks the input and gives a string as output yeah
?
and StyledComponent replaces the element call
hmm?
i created <Button> with styled components. with cva I would use regular dom components and do
<div className={button()} />
(or some syntax like that)Yep
Got it
(i think)
As you expand your design system you'll likely need to switch to cva for stuff like buttons, since they by design need some level of customisation
or i would create <BlueButton> and <GreenButton>
which would get messy
I wouldn't recommend that ^ just for colors
you'll have an explosion of buttons
just as an example
yeah
ye just cva it, you'll have typesafety with what variants you can pick and you'll have a great time
btw the typesafety comes from cva's
VariantProps
helper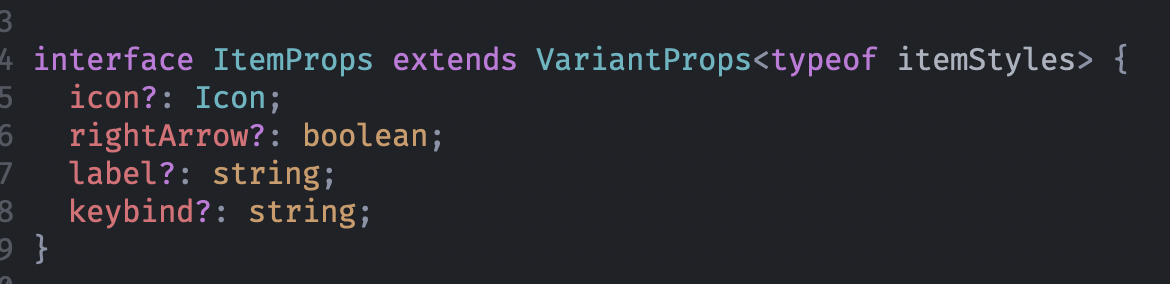
This example adds on some additional, non-styling related props to those from cva
interesting
the CVA syntax still feels daunting... but the explosion of buttons sounds worse
and the typesafety is nice
trust me, you'll come to love it
but i like calling <Button>
if u want to pick a button color it'll be like
<Button color="red" size="lg"/>
btw thank you for this guidance
or instead of color you can drive it via
variant
which handles things like colors
behind the scenesVercel
YouTube
Tru Narla: Building a design system in Next.js with Tailwind
Learn how to set up a design system in a Next.js application, and how to build an accessible and easily customizable user interface with Tru Narla, Software Engineer at Discord.
Design at the moment of inspiration: https://vercel.fyi/WEIb8yH
Speaker: https://twitter.com/trunarla
ye we use the variant approach
was just gonna grab that video haha
yeah great video
If i want to pick a button color with styled <Button> I would do <Button className="bg-red-500"> and make a mess...
thanks guys gonna watch that video
ehh it's not so bad, having the ability to override is always nice
just don't do it too often
can have the best of both worlds
yeah i dont think its so bad
i will say that what this approach doesn't address is themeing
im doing it already in my second component
Your
Button
can take variant
prop and also spread ...delegated
or w/e you wanna call other props to overrideive done styled compoinent themeing before with the styled theming HOC
depends on how strict you wanna be I guess... normally a design system I don't think would allow for such a freeform escape hatch like
...delegated
, but it dependswatching that video
yeah styled does everything at runtime i think so they can just swap out colors and such, we have a custom themeing system built on css variables
good stuff to think about
instead of using regular tailwind colors we use predefined ones that can be altered with variables
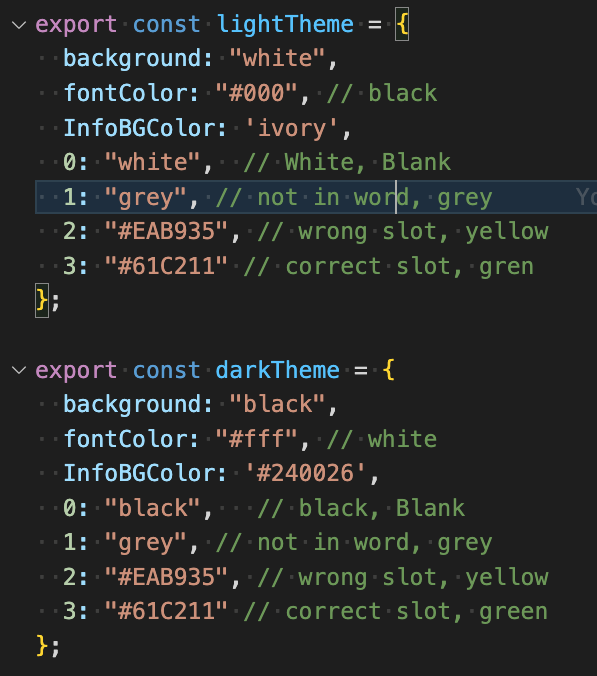
from a simple project i did
classic styled components
WordCheater - The Wordle Solver, and much more
WordCheater helps you find 5 letter words!
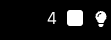
click the little sware
square
>yeah styled does everything at runtime
when does cva do it?
build time?
(this is my first time using tailwind btw)
yeah tailwind classes generate css at build time
to remedy that we've added colors to our tailwind config that are derived from css variables
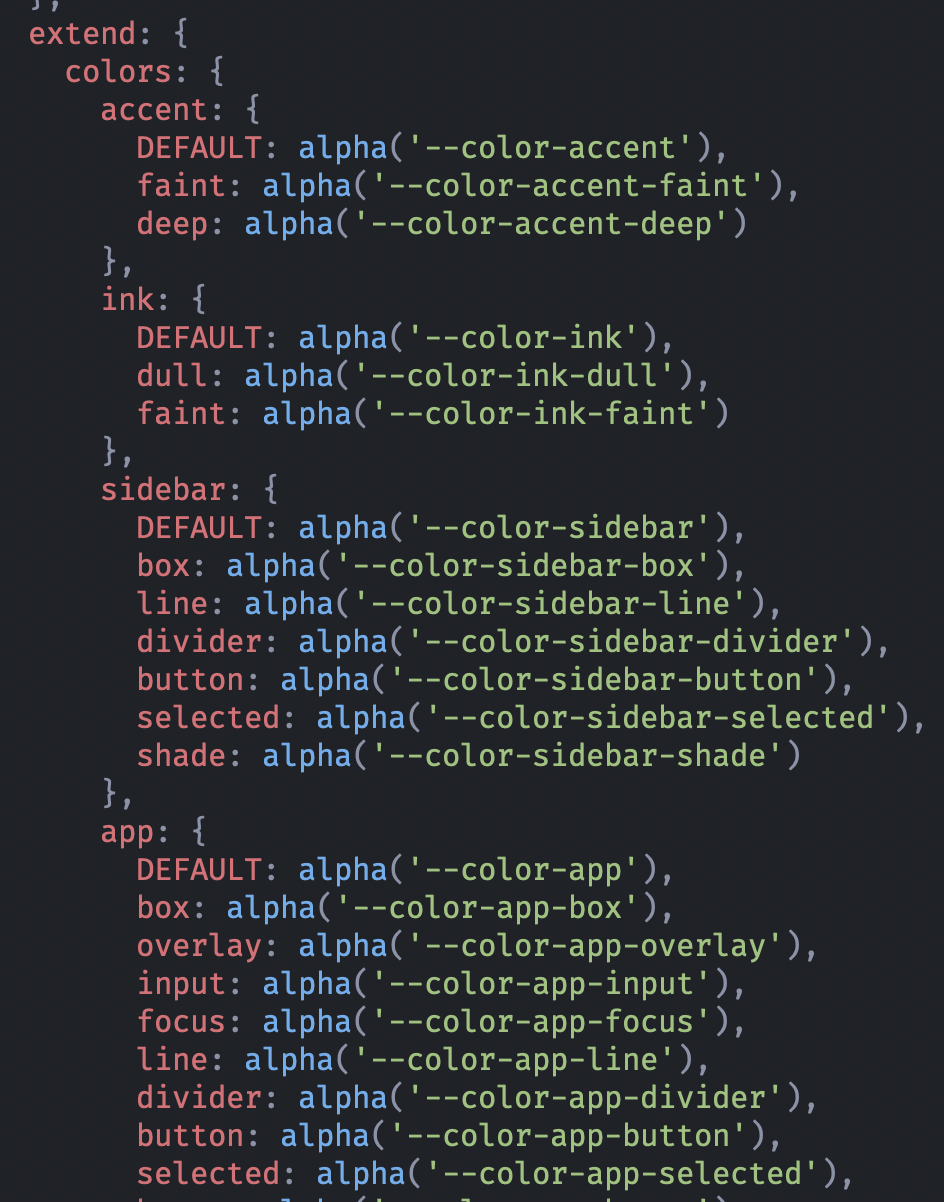
how do you call those colors?
i think i have to watch a couple videos on tailwind 🙂
just like regular tailwind colors
bg-blue-500
can be bg-app-button
className="ink-dull"?
text-gray-200
can be text-ink-faint
oh i see
they can extend the type of enity that's getting selected
yeah u can fully customise tailwind stuff
cool @Brendonovich thank you. You dont know wht you dont know... lot to know here
happy to help haha
gonna deep dive
Ohh coming back to this - is Styld components slow for some reason? i think its compiled by swc?
It does all of its style shit at runtime
https://www.useanvil.com/blog/engineering/react-styled-components-best-practices/ here's an example from a quick google search
Anvil
Measuring React styled-components performance & best practices
Learn how to measure the performance of your React styled-components and implement best practices to keep your code concise and efficient.
for small apps or w/e it's probably not gonna matter too much
but if I were starting a new project today I would not choose a runtime CSS-in-JS solution
idk if your particular tailwind variety of styled-components does something differently
I would probably go for vanilla extract like someone mentioned higher up in the thread
since there's no runtime cost to my knowledge
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
That looks much neater
thank you HerrSchade.
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
Oh I didn’t know that haha
Random question: In that second picture, why do you have that undefined selected variant? Wouldn’t that be solved with a
defaultVariant
?yeah it would, i didn't make those styles tho so it's been done that way haha
will refactor a bunch of that stuff at some point
could also use clazzx
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
hi @Kharann i dont recall having a problem with the color preview

this one right?
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
sorry what is the question
?