❔ Swagger Open API not showing Request examples in the UI
I've written an Azure Function with Open API in C#, but when I open up the Swagger/UI to test my Azure Function, the "Request Body" example only shows types instead of example values..
Here's the function:
public static class MyFunction
{
[FunctionName("MyFunction")]
[OpenApiOperation(operationId: "Run", tags: new[]{"Run"})]
[OpenApiSecurity("function_key", SecuritySchemeType.ApiKey, Name = "code", In = OpenApiSecurityLocationType.Header)]
[OpenApiRequestBody(
contentType: "application/json; charset=utf-8",
bodyType: typeof(MyFunctionRequest),
Description = "Sample Description",
Required = true,
Example = typeof(MyFunctionRequestExample) )]
[OpenApiResponseWithBody(
statusCode: HttpStatusCode.OK,
bodyType: typeof(MyFunctionResponse),
Description = "The Ok Response",
Example = typeof(MyFunctionResponseExample) )]
public static async Task<IActionResult> RunAsync([HttpTrigger(AuthorizationLevel.Anonymous, "post", Route = null] HttpRequest req, ILogger log)
{
// Function logic
}
}
public static class MyFunction
{
[FunctionName("MyFunction")]
[OpenApiOperation(operationId: "Run", tags: new[]{"Run"})]
[OpenApiSecurity("function_key", SecuritySchemeType.ApiKey, Name = "code", In = OpenApiSecurityLocationType.Header)]
[OpenApiRequestBody(
contentType: "application/json; charset=utf-8",
bodyType: typeof(MyFunctionRequest),
Description = "Sample Description",
Required = true,
Example = typeof(MyFunctionRequestExample) )]
[OpenApiResponseWithBody(
statusCode: HttpStatusCode.OK,
bodyType: typeof(MyFunctionResponse),
Description = "The Ok Response",
Example = typeof(MyFunctionResponseExample) )]
public static async Task<IActionResult> RunAsync([HttpTrigger(AuthorizationLevel.Anonymous, "post", Route = null] HttpRequest req, ILogger log)
{
// Function logic
}
}
3 Replies
and here's my Request class:
and response class:
public class MyFunctionRequest
{
[OpenApiProperty] public string FirstProperty {get;set;}
[OpenApiProperty] public List<string> SecondProperty {get;set;}
}
public class MyFunctionRequestExample : OpenApiExample<MyFunctionRequest>
{
public override IOpenApiExample<MyFunctionRequest> Build(NamingStrategy namingStrategy = null)
{
Examples.Add(OpenApiExampleResolver.Resolve("MyFunctionRequestExample", new MyFunctionRequest
{
FirstProperty = "Some Prop Value",
SecondProperty = new() { "Other", "Prop", "Values" }
}));
return this;
}
}
public class MyFunctionRequest
{
[OpenApiProperty] public string FirstProperty {get;set;}
[OpenApiProperty] public List<string> SecondProperty {get;set;}
}
public class MyFunctionRequestExample : OpenApiExample<MyFunctionRequest>
{
public override IOpenApiExample<MyFunctionRequest> Build(NamingStrategy namingStrategy = null)
{
Examples.Add(OpenApiExampleResolver.Resolve("MyFunctionRequestExample", new MyFunctionRequest
{
FirstProperty = "Some Prop Value",
SecondProperty = new() { "Other", "Prop", "Values" }
}));
return this;
}
}
public class MyFunctionResponse
{
[OpenApiProperty] public string Result {get;set;}
}
public class MyFunctionResponseExample : OpenApiExample<MyFunctionResponse>
{
public override IOpenApiExample<MyFunctionResponse> Build(NamingStrategy namingStrategy = null)
{
Examples.Add(OpenApiExampleResolver.Resolve("MyFunctionResponseExample", new MyFunctionResponse
{
Result = "Some return value"
}));
return this;
}
}
public class MyFunctionResponse
{
[OpenApiProperty] public string Result {get;set;}
}
public class MyFunctionResponseExample : OpenApiExample<MyFunctionResponse>
{
public override IOpenApiExample<MyFunctionResponse> Build(NamingStrategy namingStrategy = null)
{
Examples.Add(OpenApiExampleResolver.Resolve("MyFunctionResponseExample", new MyFunctionResponse
{
Result = "Some return value"
}));
return this;
}
}
This image shows the actual "example" that OpenApi renders
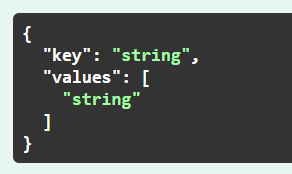
Looks like nothing has happened here. I will mark this as stale and this post will be archived until there is new activity.