❔ How do i count how many different vowels are in a string?
The only method i've come up with is to count how many vowels are in a string not accounting for duplicates.
This is the code so far:
char[] balse = { 'a', 'A', 'e', 'E', 'i', 'I', 'o', 'O', 'u', 'U' };
Any help is appreciated
43 Replies
yeah seems good
the best way would be to use
Array.IndexOf
or a binary search maybeWouldn't my code count duplicates too? i only need to know the amount of unique vowels
oh you don't, alright
well probably just mark a vowel whener you find one
with another
bool[]
or BitArray
or just by marking bits on an intWell you can use linq but I don't know if it's worth for you to learn it rn
or a
HashSet<char>
, or a List<char>
there are a bunch of ways, the int with marking bits is probably the fastestCould you type an example? haven't really dealt with text editing that much
so like when you find a vowel at position N, set the bit at position N in that int. If it's already set, don't increment the count
with an int
with a bit array
same with
bool[]
it's a general thing, it has nothing to do with text editing in particularJust tried it out and it works, thanks a lot ;D
np
note that the first one is undefined behavior if the length of
balse
is more than 32
be sure to use bit operations carefullyAnother question then, say i have 3 lines of text, and i want to move the 2nd line all the way to the top, how should i approach this ?
split by new line characters, swap the needed lines, join the text back
but if you're doing text editing software, you probably should store it split by linea internally
that would make sense either way
i know how to split text into lines words etc, how do i move it to the top though
I think
all the lines asides from the one i want to move up should stay in the same position
the same way you swap values of two variables, swap the values of two positions in the array
oh hold on
you mean you want to move the rest below them
say these are the lines
1 yes
2yup
3 maybe
4 no
this should be the output:
3 maybe
1 yes
2yup
4 no
1
2
3
4
3
4
1
2
let's say these are the lines
right?
pretty much instead of moving 2 i need to move 1 only
yeah with a single line moving it's a bit simpler than moving a chunk
its a weird task with a bit of limitations, but to put it simply, find the longest word in a text file, move that line that has the longest word to the top
the idea is to remember the line you want to move, then move all the lines before it one up, then write it into the first postion
okay so treat the lines of the text as an array and then simply make space for a line at the start with double for ?
or is it easier in c# ?
double for?
two 'for' cycles
you only need a single for
to move the lines one up
you could also just use a list
it does a bit more work than moving stuff manually tho
this moves twice as many items on average
would lines.ReadAllLines(filename); work to fill up the list?
ah no, three times as much
oh wait no
but just move manually, it's like 4 lines of code
LINQ:
Aight, thanks a lot for the help :D
just do a
ToHashSet()
and keep it a set
whats the point of converting it to a string if you've already got a sethmm true
(distinct makes a set internally)
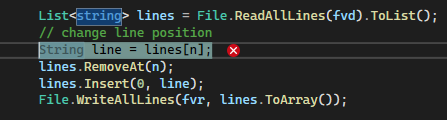
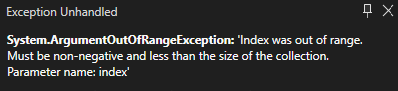
n is 5, my text file has 7 lines
error is pretty clear, no?
i mean evidently it does not
ye nvm mixed up the names of the text files...
string inputString;
inputString = Console.ReadLine();
int vowelCount = 0;
foreach (char c in inputString)
{
if (c == 'a' c == 'e' c == 'i' c == 'o' c == 'u')
{
vowelCount++;
}
}
Console.WriteLine(vowelCount);
@softmek It's neither concise nor fast solution and it counts duplicates too
You can immediately get the count without storing the score in the string
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.