✅ need help with a problem
i know what im supposed to do but im having trouble implementing it. i need to add the sum of a list except for the minimum value and do the same but this time except the maximum value
82 Replies
i could do
that would get me one of them
it has to be in the form
x y
ill give an example
1+2+3+4 = 10
2+3+4+5 = 14
answer: 10 14
im curious why this worked for 3/14 tests, but failed the rest
the error ^
Overflow means you went past the end of int. Probably added two very large ints. That went beyond the valid range of int
hmm
ill send some of the numbers being tested
@Deneri
this is 1 of the 14 inputs being tested against my algorithm
they are adding massive numbers. the question is, what do i do about that?
all the tests past if the numbers were much lower
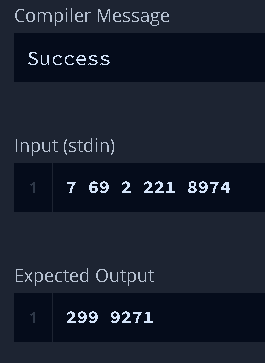
that passed for example
if the problem calls for me to add the numbers, but the numbers are too large... it kinda seems impossible from my pov. maybe there are some solutions to this though, but i cant think of any
Don't store the result in an int.
An int is too small. Store the result in something bigger.
alright
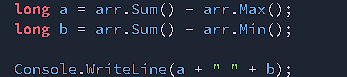
i stored it as a long
same tests pass
same ones fail
i guess the calculations are still using an integer
im stumped
Don't forget to change the type of the arr parameter to List<long>
yeah i tried that. it seems to break the whole program.
What's the expected method signature?
Okay, what's the current problem
With
long
there should be no overflowsthe results of both calculations are being stored in the variable
but the list is an
Oh I didn't notice it's a
int
You can modify main
or no?i dont believe im supposed to
i mean i can fix it
if i edit main
i could give that a go, just im not sure if i m supposed to do that
edited verison
@Kouhai it passed all the 14 tests
i couldve done that the whole time. i guess i was just under the impression that i wasnt supposed to edit main
If you don't want to edit main you can do this
im curious, what is |
doing?
It casts the int in arr to long before summing
does the "m" mean something specifically?
No, m is just the name I chose randomly
It's basically a variable name
oh
where was it initialized?
or i guess it was initizilized in that that scope
ive never seen that so im pretty confused on which each component is doing
That syntax is for lambda expressions in C#
If you don't know what lambda expressions are, they are basically an anonymous function that can be executed.
(If this is more confusing and makes it harder, reading the docs might help)
https://learn.microsoft.com/en-us/dotnet/csharp/language-reference/operators/lambda-expressions
Lambda expressions - Lambda expressions and anonymous functions
C# lambda expressions that are used to create anonymous functions and expression bodied members.
alright thank you 😄
But also you can just do
what does Cast do?
Cast<T>
as the name suggests casts an IEnumerable<T> to another IEnumerable<T>@Kouhai quick question, how can i check whether a number is odd or even?
nvm
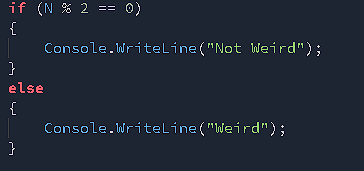
im curious why this worked
24 / 2 doesnt = 0
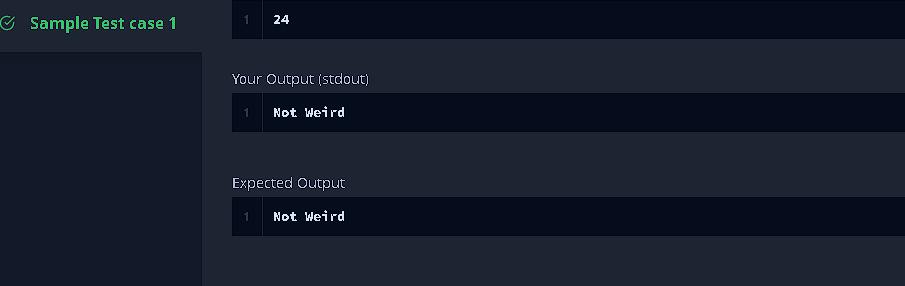
it failed 2/8 tests
the rest did fine
wait it isnt dividing it
%
thats what "/" would do
yeah
%
is modulo operatorbut im curious why 2 tests failed
but 6 passed
20 % 2 = 0
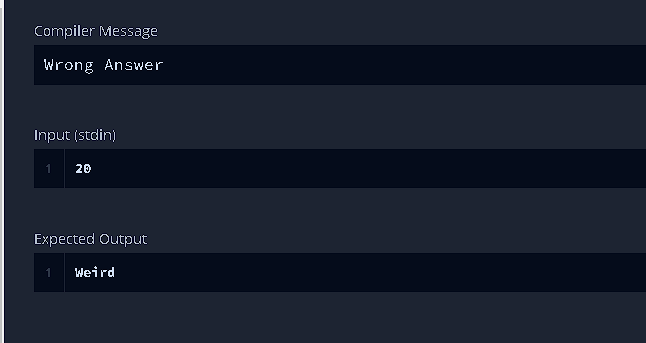
so why is it printing "Weird" here
20 % 2 will return 0, I'm not sure if another part of the code is causing that error
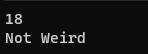
i ran the code in vsc
and it prints "Not Weird"
i dont think the problem lies with my code..
Yeah, if
Weird
should be printed for odd numbers, then the test case above is invalidim not sure how i can pass this problem then
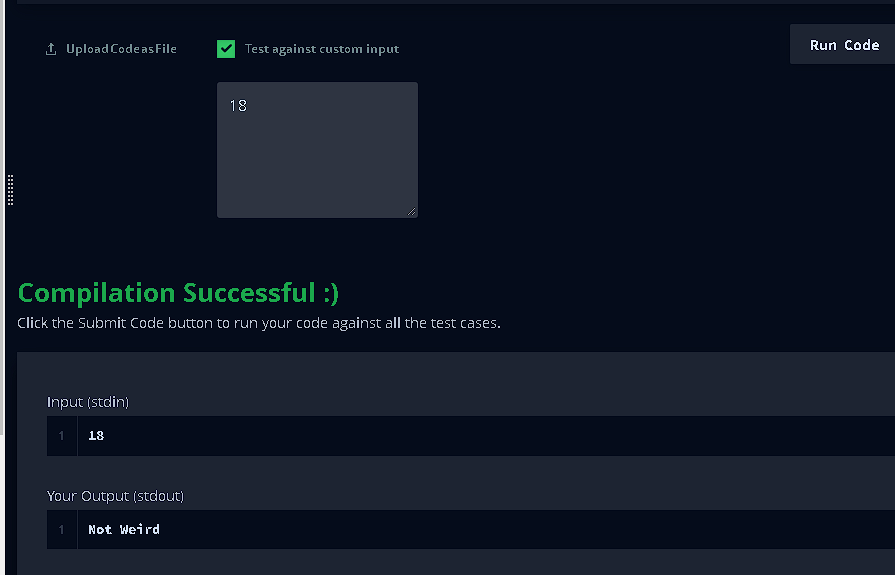
Can you show the description of the problem?
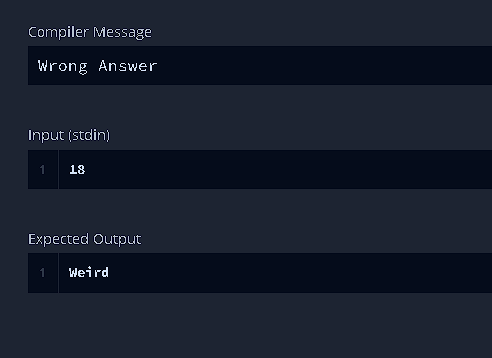
why is it giving me 2 answers?
it isnt giving me an error or anything if that's what you mean
No, I mean what are the requirements?
oh
Task
Given an integer, , perform the following conditional actions:
If n is odd, print Weird
If n is even and in the inclusive range of 2 to , 5 print Not Weird
If n is even and in the inclusive range of 6 to , 20 print Weird
If n is even and greater than 20, print Not Weird
Print Weird if the number is weird; otherwise, print Not Weird.
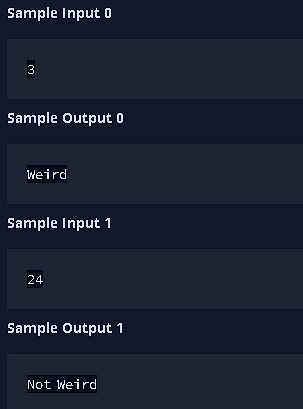
wait i think there is a little bit more i have to do
i didnt read this part
If n is odd, print Weird
If n is even and in the inclusive range of 2 to , 5 print Not Weird
If n is even and in the inclusive range of 6 to , 20 print Weird
If n is even and greater than 20, print Not Weird
ok i have to nest some if statements. i know how to do this now
alright i believe i fixed everything
nvm...
from reading that, isnt this supposed to print 18
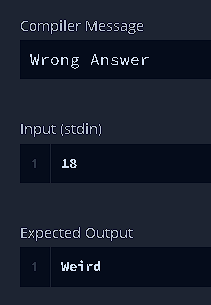
thats my updated code
How can a number smaller than 6
yet bigger than 20 ?
oh wait i know what i need to do
it should be ||
it's still failing 3/8 tests
im not really sure what to do
If n is even and in the inclusive range of 2 to , 5 print Not Weird
why is this wrong then?
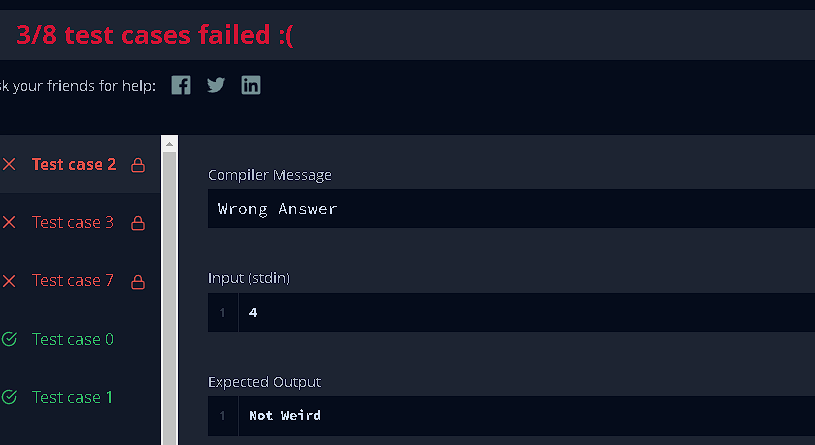
What's the problem exactly ?
I mean assignment
oh wait i saw
mb
wait i see a couple mistakes
i gtg for a little. i think i know how to solve it
What is the expected result of 0 ?
btw if number is expected in range (inclusive)
It should be n >= 2 && n <= 5
yeah i mixed around the the greater than and less than symbols
i havent seen a switched used like that
hmm
i thought it had to be
so result is a bool that checks if the number is % by 2 == 0
what does the underscore and "=>" mean
i have seen those symbols but i dont really understand what they do
It's lambda and switch expression
so even numbers supposed to be Output "Weird" right ?
sorry odd
yeah i think so
can you try this ?
If this doesnt work
This should work
i got past the problem
a few hours ago
i shouldve mentioned that
think this is more concise, at least to me
more concise
less readable
:&
what does the _ mean there
"default"
oh
last _ is default
what about the one that is in the middle
others are discarded parameter/variable
conciseness is basically a synonym for readability
Not really for "shortness"
Plus it doesn't have an "I don't know" path
i mean @Samarichitane version was definitely more readable but it doesnt really matter
thanks for the help
both of you 😄
He got shortened tho
yeah
nice to see all the different ways you guys took it apart
I'd agree that their version makes it more clear what's happening
You can close thread with /close @shawski
Think mine's just more efficient
alright
Closed!