β while loop help(im new ish so its probs obvious)
'''cs
namespace HippityHop
{
internal class Program
{
static void Main(string[] args)
{
Console.WriteLine("** HIPPITY HOP **");
Console.Write("Please enter an integer between 1 and 100:");
// WRITE YOUR CODE BELOW THIS COMMENT
string input = Console.ReadLine();
int num = Convert.ToInt32(input);
while (num <= 100 || num >= 1)
{
Console.Write("Please enter an integer between 1 and 100:");
num++;
return;
}
for ( num = 1; num == num; num++ )
{
if(num % 3 ==0)
{
Console.WriteLine("Hip");
}
if (num % 5 == 0)
{
Console.WriteLine("Hop");
}
if (num % 5 == 0 && num % 3 == 0)
{
Console.WriteLine("Hop");
}
}
}
}
}
'''
60 Replies
basically i do my input and then after it does show the "please enter integer", but it wont let me type or show anything else and it does the press any key to close command prompt
i tried few things (thats why theres return and num++) but im sure i use return somehow idk lol
You have an ||
or
in there.
Your condition is ALWAYS trueUse backtick (`), not apostrophe (') for markdown $code highlighting
To post C# code type the following:
```cs
// code here
```
Get an example by typing
$codegif
in chat
If your code is too long, post it to: https://paste.mod.gg/so i use &&
Wait a sec, I think I might have misread
Please hold π
i think i might be something else in my code
i feel like the OR should work
The condition isn't always true
It just does the exact opposite
Of what you want it to do
i have to make it so it keeps prompting while input isnt between 1 and 100
thats what that is
That's not what that is
I think its both @Ero π any number is either smaller than 100 or greater than 1
Ah, right
The code inside the while is also nonsensical
the for loop is weird and looks like its also endless
Absolutely
num == num is a strange condition to use @SAFE
You're overwriting the user's input
oh yes i was working on that too
So the problem as I see it
Once the user enters a valid integer, use a for loop to loop through the values between 1 and the
number that the user entered
You take the input, you check if its EITHER smalelr or equal to 100
thats what that is; or am trying to do
OR larger or equal to 1
which will always be true for any number
oooh
you increment it by 1, print somethign and then end the program
trueee
okok
im trying to make it not end
So don't use return
if you want to keep asking for a valid input, a while loop is a perfectly reasonable choice
Not just that, it's your best choice
you probably just want to repeate the ReadLine instead of the num++
And drop the return, because that just returns from your Main, thus ending program execution π
ooh okok
that helped
didnt end
You could (perhaps should) use int.TryParse instead
it returns a boolean depending on if the conversion was succesful, rather than just crashing when it doesnt work
@SAFE Is it doing what you want or just not quitting early? π
had to go do something just earlier thats why i answer now; it isnt quitting anymore but i havent quite figured out how to get what i want working
i think i just need to change what i need in the brackets in while ( ) and for ( )
like this lol
You could get valid input like this for example
Ok, then what did you want again, let me double check π
So you had the general syntax for a for-loop correct, but using the input as the iterator variable is probably not what you want.
Insteead do something like
You don't want to be modifying the original input by incrementing, like you are doing. Because then you immediately lose track of what it was and to which number you want to loop. That's why your original condition could never have worked.
<= cannot be applied to int and string
static void Main(string[] args)
{
Console.WriteLine("** HIPPITY HOP **");
Console.Write("Please enter an integer between 1 and 100:");
// WRITE YOUR CODE BELOW THIS COMMENT
string input = Console.ReadLine();
int num; while (!int.TryParse(Console.ReadLine(), out num) num < 1 num > 100) { Console.WriteLine("Please choose a valid number between 1 and 100"); } { Console.Write("Please enter an integer between 1 and 100:"); Console.ReadLine(); } for (int i = 1; i <= input; i++) { if (i % 3 == 0) { Console.WriteLine("Hip"); Console.ReadLine(); } if (i % 5 == 0) { Console.WriteLine("Hop"); Console.ReadLine(); } if (i % 5 == 0 && num % 3 == 0) { Console.WriteLine("Hippity Hop"); Console.ReadLine(); } } } } }
int num; while (!int.TryParse(Console.ReadLine(), out num) num < 1 num > 100) { Console.WriteLine("Please choose a valid number between 1 and 100"); } { Console.Write("Please enter an integer between 1 and 100:"); Console.ReadLine(); } for (int i = 1; i <= input; i++) { if (i % 3 == 0) { Console.WriteLine("Hip"); Console.ReadLine(); } if (i % 5 == 0) { Console.WriteLine("Hop"); Console.ReadLine(); } if (i % 5 == 0 && num % 3 == 0) { Console.WriteLine("Hippity Hop"); Console.ReadLine(); } } } } }
Well, you were a bit too literal there π
You copied my example, you have no variable called input however. I just picked the name randomly to illustrate the point
You want to compare the iterator variable against your parsed input, num
ooh i was confused cause i had already soemthing named input
Happens, you also should remove the stuff between the extra brackets after the while loop.
You are just taking extra input unnecessarily π
Also, do you want execution to pause after every print in your for-loop? It should give you the right output now, so you can just let it run in one go.
just all at once after i type
All good then?
close
im still trying things
Well I'll be off, if anything else comes up, I'm sure you can find a helping hand around here somewhere.
i think i did get it actually
just now
yeah this place seems great for help
so i almost got it, its just that it is not showing the non-multiples. its only shows the multiples(hip, hop, hippity hop)
In bed but still awake π
You are only testing for the multiples and discarding all others.
You could append a final
else
and just print i in that case.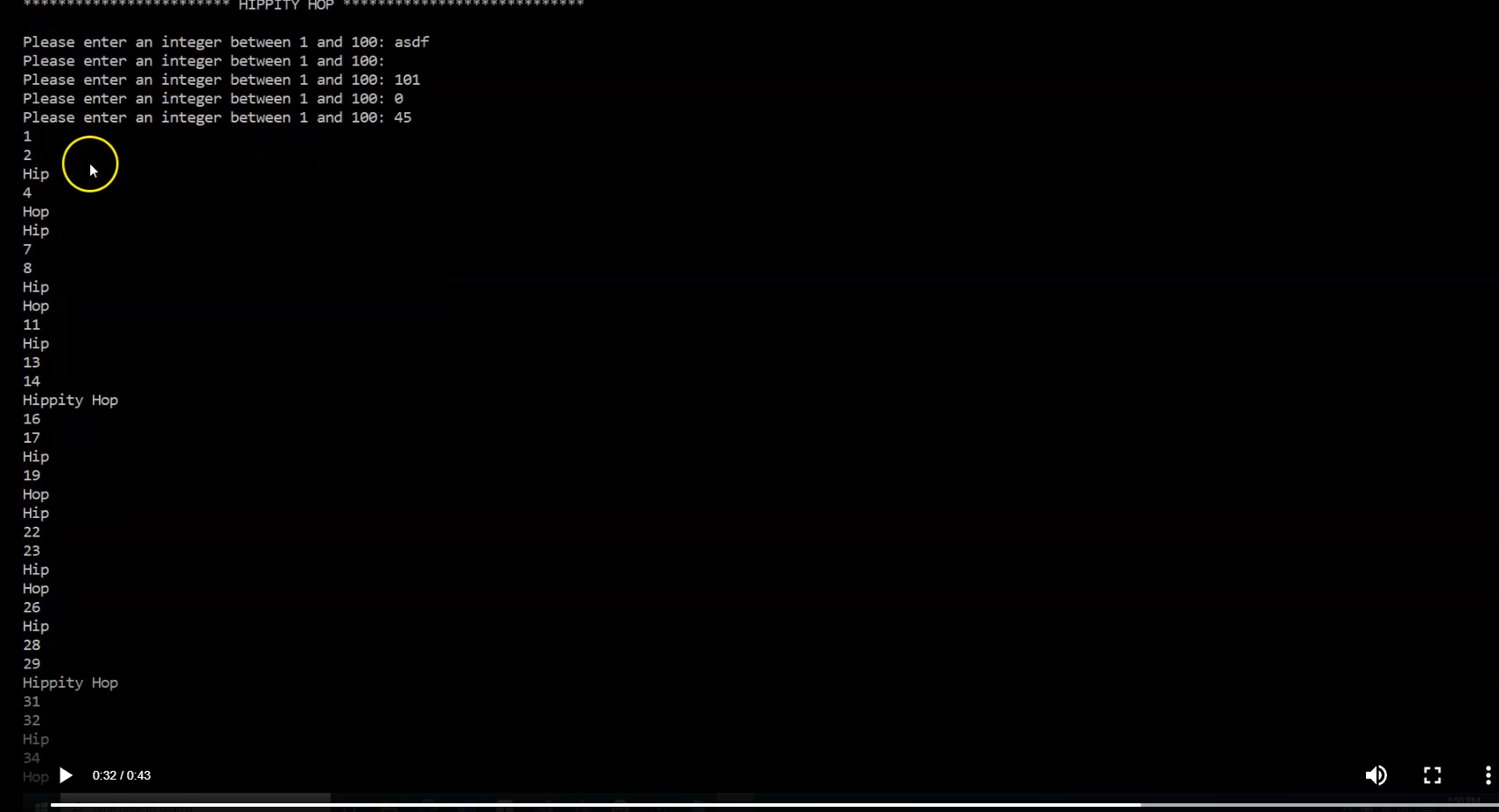
it should like this for 45 but it shows 33 lines which are only hip/hop/hippity hop
and also theres defo not 33 multiples lol
an else makes sense
The screenshot is what you want or what you have?
what it should look for for 45
You should als make your last two ifs into else ifs.
The way it is now, 15 for example would make all 3 true, thus 3 prints
this is what it is for me
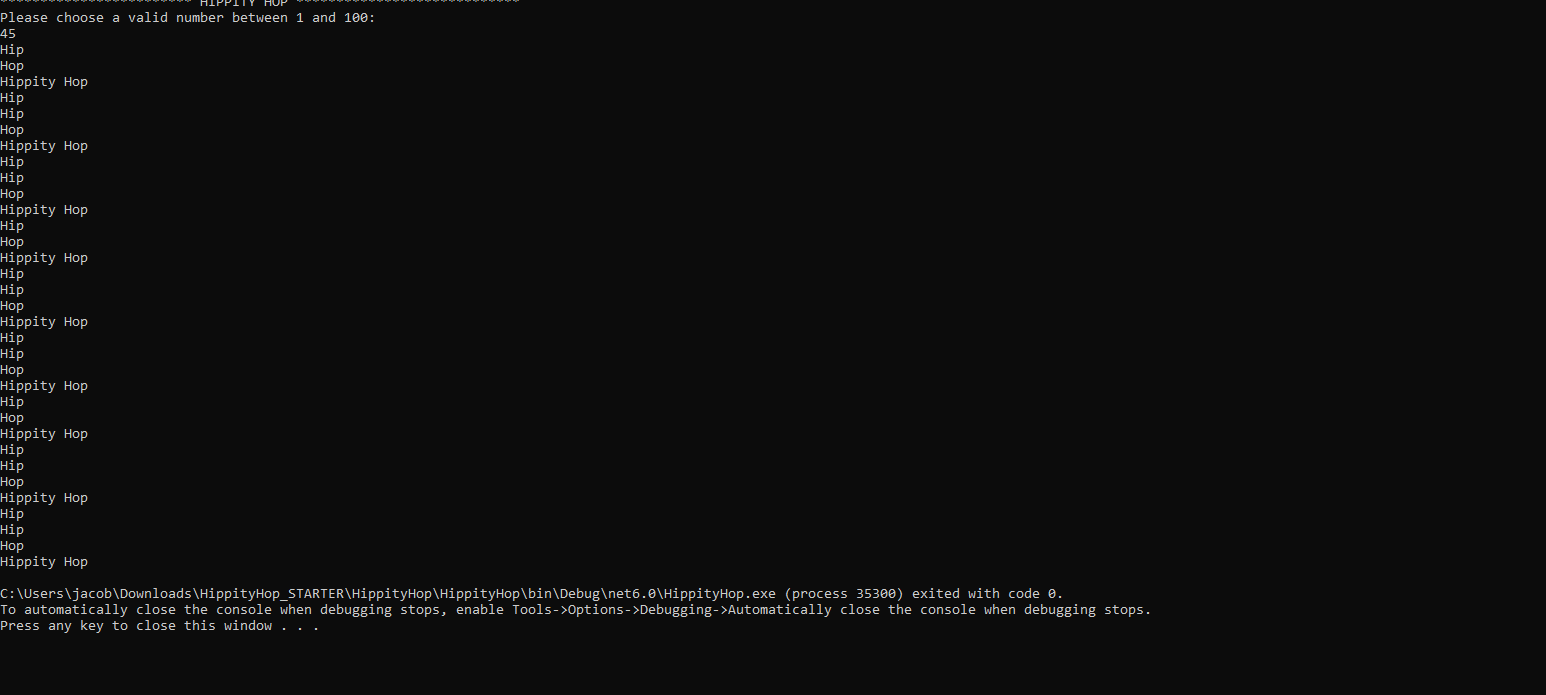
Yes, only hips and hops because of no final else to catch non-multiples.
And too many because some numbers fulfill multiple conditions.
yeah im confused about that part
so i think i should put two else ifs instead of if
two else ifs under first if
Unknown Userβ’2y ago
Message Not Public
Sign In & Join Server To View
Actually, you should test for mod 3 AND mod 5 first of all.
Chain the other two with else ifs so only one of the three happens for any given number.
Add a final else clause for any numbers that dont match any of the three.
how would i do
trying to figure out
cant think of what would be good to put in it
i++ maybe ill try
hmm
feel like its something with i++ since each line increments by 1 till the input number
got it !! console.writeline i worked
so theres no hops at all for 45.. when in the example when he types 45 he gets all three
@Stalli help please if u want ? : )
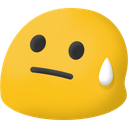
Unknown Userβ’2y ago
Message Not Public
Sign In & Join Server To View
ooh ive heard of that
ill look at that
so my assignment is fizzbuzz in disguise
basically
Got it working?
yes itβs all good i think; i submitted it earlier
i got one more little assignment due for next week and then final test on the 15th
please use the
/close
command to mark this thread as answered πClosed!