How to properly redirect to different page using trpc?
export default function Post() {
const { id } = useRouter().query;
const postByIdQuery = trpc.post?.byId.useQuery({
userId: session?.user?.id,
id: String(id),
});
const { data: post } = postByIdQuery
if (!post) {
return <Error statusCode=404>
}
return (
<h1>{post.title}</h1>
<p>{post.desc}</p>
)
}
Previously i use the getServersideProps and if the api returns nothing then simply redirect
the above method does not render the 404 page for some reason75 Replies
Just wondering whether the above method is correct?
purely client side you can hook into the onSuccess/onError prop on ur query and redirect using the next router from useRouter
use the onError callback that useQuery has
That would work if they’re throwing an error but not if he’s returning nothing right
That’s why I mentioned both
oh yeah
just do if (!thing) { throw new TRPCError({ code: NOT_FOUND })
throwing a 404 is the server's responsibility
i mean you can redirect to the 404 page from the client arbitrarily, but seems bad
Yeah i agree
Any solid example can share, sorry im a newbie
@cje @CFKeef
Thanks guys
Error Handling | tRPC
Whenever an error occurs in a procedure, tRPC responds to the client with an object that includes an "error" property. This property contains all the information that you need to handle the error in the client.
I can post a direct solution but try using the docs first and if anything I can hold ur hand with questions you may have 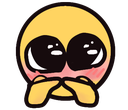
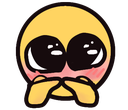
hahaha @CFKeef im a bit stuck right now
I don't think i can reroute example
because its a TS file i dont think i can use useRouter or something
The use router is for the client side
In your original code block you can pass in a object to the useQuery arguments
That let you adjust how your query functions
Use these docs for that
It’ll look something like this
.useQuery(input,{})
I’m on my phone right now but I can give you a nice formatted code block in a little
Something like the above?
Just wrap it in braces since it’s expecting an object
There’s also this nice prop in there “enabled” that will let the query only run when the condition is met which is good to know in general
Something like this right
The enabled thing isn’t necessary I’m just mentioning it for ur future
I’m just googling to see if trpc lets you redirect to see if you can do it serverside or if clientside is the only way
I tried to do serverside with getServerSideprops
its not working and found a github thread that the only way is clientside
You can type the error as TRPCError btw or see if it’s implicitly typed without the any
If so then ye that should work
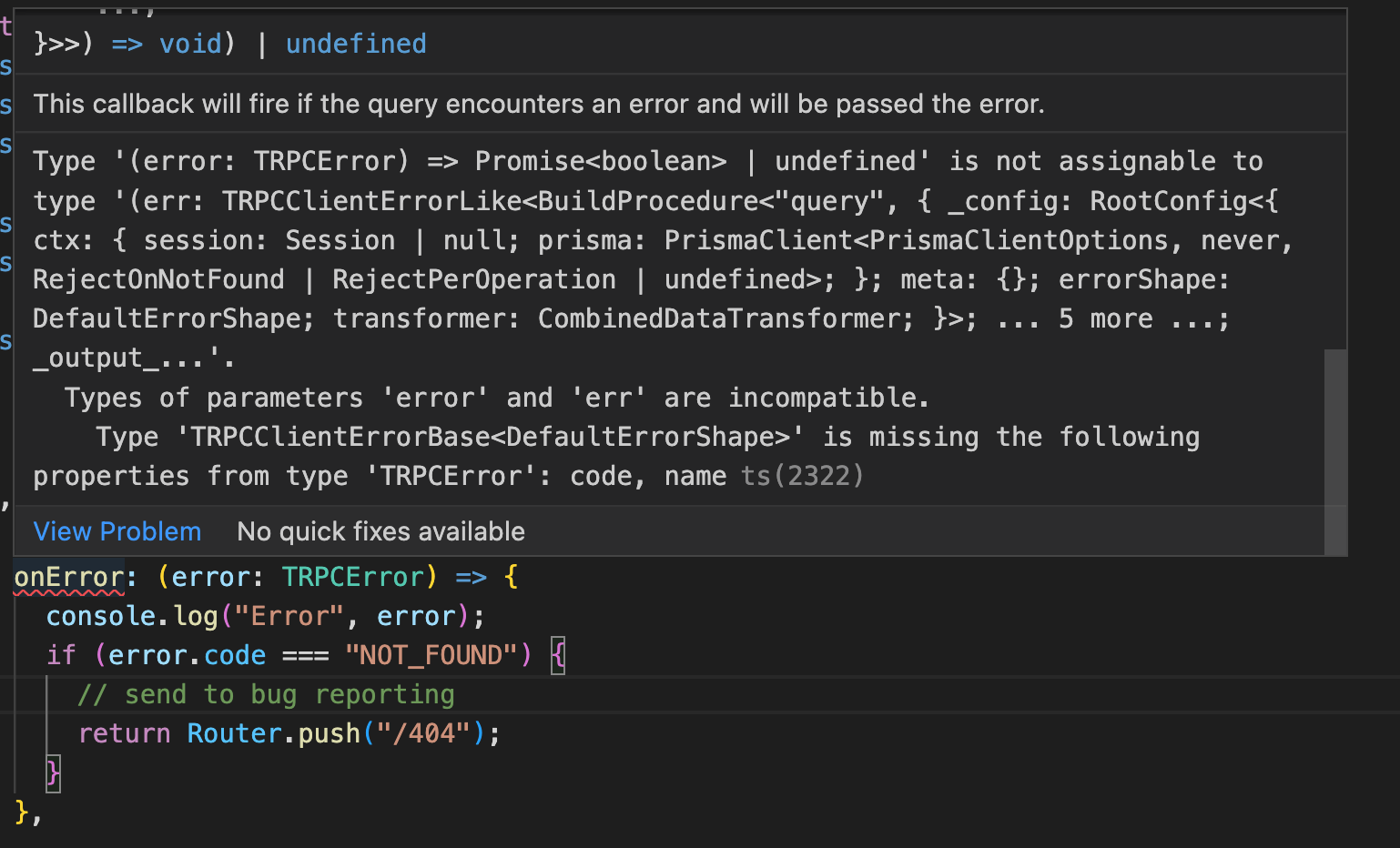
import { TRPCError } from "@trpc/server"
It might have a type already
The general rule for typescript is see if it’s implicitly typed already (meaning don’t need to assign it using : <type>) then explicitly type it
See if removing the type and hovering gives you a type
I avoid explicitly typing so I just assumed trpcerror would work since that’s the type on the server
sorry
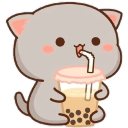
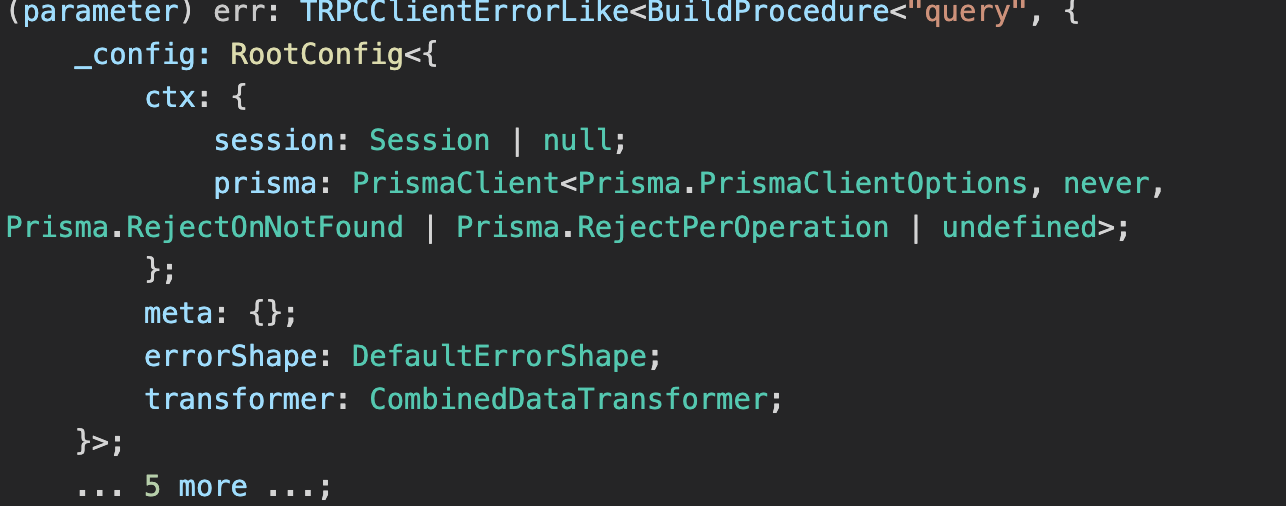
No worries man 🙂
Yeah so someone did the hard work for
it’s implicitly typed and ur good 2 go
Are you seeing any issues?
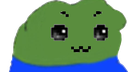
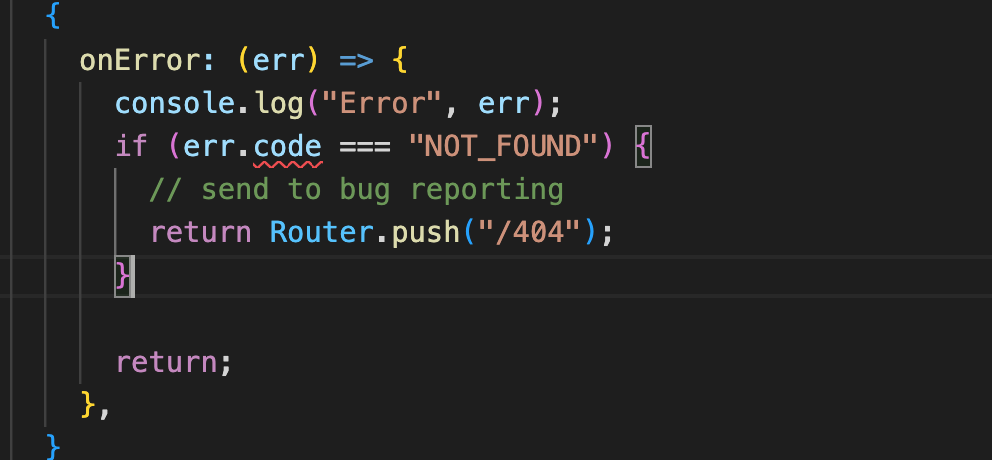
I tried to load the page but it does not redirect to 404 for some reason
although the error is obvious
Ur conditional is prob failing
Check the red squiggly
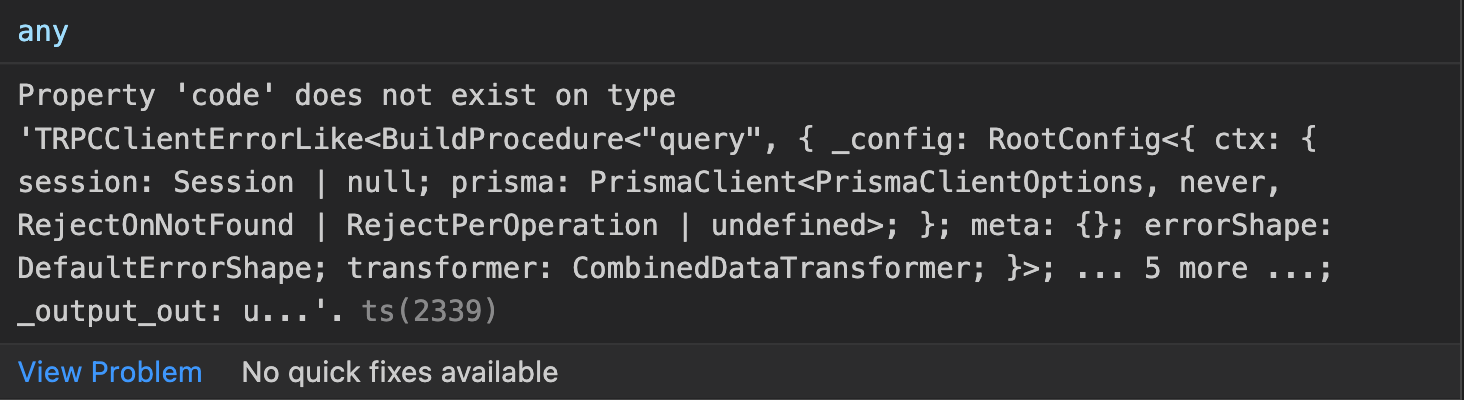
Yeah I’d say console.log it and rerun the page to see what’s available for you
You can also ctrl click a type to get to its type declaration but that’s a scary typescript world and you might be overwhelmed
its err.data.code
the onError approach has some issue, it will load the page and then only it will trigger the onError which will lead to 404
Yeah thats the downside of clientside
Oh my
Same with checking user session on client side, it'll have to render the page then redirect if they dont have one for example
I wish i can do trpc on getServerside
Ill see if i can dig something up since I'm back at my pc
but you really dont need to do this
Okay thanks man
im too lazy to rewrite my trpc code to api based
Just render the 404 content in this component when it errors like that
I use trpc strictly and dont use the nextapihandler
Atleast I haven't found the need to yet
Do you have any code example on github that i can learn from, i really need to push this project out haha
digging something up
okay cool thanks alot
https://github.com/t3-oss/create-t3-turbo/tree/main/packages/api heres how I structure my trpc generally
GitHub
create-t3-turbo/packages/api at main · t3-oss/create-t3-turbo
Clean and simple starter repo using the T3 Stack along with Expo React Native - create-t3-turbo/packages/api at main · t3-oss/create-t3-turbo
This is a monorepo tho so don't go digging too hard
https://github.com/t3-oss/create-t3-turbo/blob/main/packages/api/src/router/post.ts direct example of a query
GitHub
create-t3-turbo/post.ts at main · t3-oss/create-t3-turbo
Clean and simple starter repo using the T3 Stack along with Expo React Native - create-t3-turbo/post.ts at main · t3-oss/create-t3-turbo
heres some usage: https://github.com/t3-oss/create-t3-turbo/blob/main/apps/nextjs/src/pages/index.tsx
GitHub
create-t3-turbo/index.tsx at main · t3-oss/create-t3-turbo
Clean and simple starter repo using the T3 Stack along with Expo React Native - create-t3-turbo/index.tsx at main · t3-oss/create-t3-turbo
Yeah the api structure is identical to mine
im using the t3 boilerplate
first time using it, looks promising hence why i tried it out for this new project
haha
I enjoy using it a lot but there are some edge cases like this for example
So you might have to just render it on the page instead of trying to redirect to a catchall 404
i see render the 404 component itself
Yep instead of redirecting to a specific page
Something like this yeah
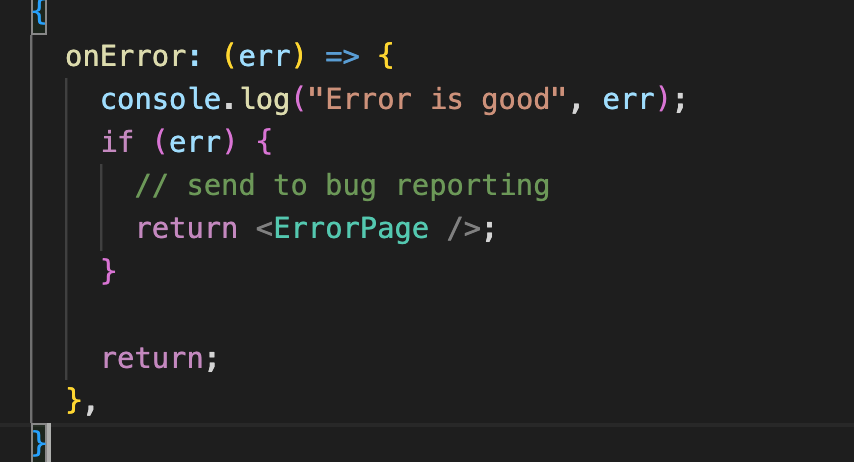
no the return from the qeury has a couple properties like isLoading,isError you want to tap into and then just conditionallyrender
ahh got it
I dont think that'll work but I treat my components more akin to a state machine where if its in this state (isError) return <p>somethign blew up</>
Same issue, it will load the page then only it will show a different component
but the url stays the same though
instead of /404
Yeah thats fine tho
oh okay
You don't need to redirect in these situations
tbh
you can but it seems like it is working against you. There are waysto clean up the search bar btw if you wanted to obfuscate this
but idk if routing is gonna get involved since I would treat this solution as enough
but the loading time to transition is slow, maybe i should i add isLoading as well/
Yeah but thats how async works
Prisma is also slow as shit when on a cold start so your performance should be fine when people are using the app
I see i think adding the isLoading did the trick
but i prefer the getServersideprops
hahaha
Theres a cost to that too 🙂
its all about trade offs honestly
thats engineering for u
if i have to add isLoading on some of the pages, im not sure though haha
Its tedious yeah
btw i notice the query will keep on running, whenever you click on the page
Thats the stale-while-revalidate (swr) pattern
that react query employs to ensure you are generally upto date
oh okay reading it right now - https://swr.vercel.app/
React Hooks for Data Fetching – SWR
SWR is a React Hooks library for data fetching. SWR first returns the data from cache (stale), then sends the fetch request (revalidate), and finally comes with the up-to-date data again.
Its not a vercel topic
React Conferences by GitNation
YouTube
React Query: It’s Time to Break up with your "Global State”! –Tanne...
Find the latest React.js talks & workshops at https://portal.gitnation.org
🗓 Talk recording from React Summit Remote Edition 2020
Website - https://remote.reactsummit.com/
See other React conferences by GitNation
React Summit – https://reactsummit.com
React Advanced London – https://reactadvanced.com
React Day Berlin – https://reactday.be...
good talk on this idea and REACT specifically
web.dev
Keeping things fresh with stale-while-revalidate
stale-while-revalidate helps developers balance between immediacy—loading cached content right away—and freshness—ensuring updates to the cached content are used in the future.
general
Thanks for sharing these 🙂
That vercel link gives you an eli5 explanation which works but if you want a deeper understanding those links will help
SWR the library is vercel's take on react query
Oh i see okay i'll watch it
i think now i need to add skeleton loader