✅ What is better, in this case, Replace() or Substring()
In Unity it seems that text input comes with a 'Zero Width Space Character' at the end, and that messes with the following logic since a word with 5 characters has 6 characters and this ZWS character ain't accepted everywhere.
Both of this solutions work fine to remove the character, but I am here asking if there is good reasons to use one or another? or even other way maybe even in unity settings to avoid that behavior.
or
69 Replies
Sal#0955
REPL Result: Success
Result: string
Compile: 601.693ms | Execution: 42.546ms | React with ❌ to remove this embed.
Sal#0955
REPL Result: Success
Result: string
Compile: 625.734ms | Execution: 42.323ms | React with ❌ to remove this embed.
did you check if this character is removed by
.Trim()
?
that's designed to remove leading and trailing whitespaceSal#0955
REPL Result: Success
Result: string
Compile: 473.508ms | Execution: 40.504ms | React with ❌ to remove this embed.
I didnt know for sure but
IsNullOrWhiteSpace()
didnt recognise the space when the field was "empty"It's hard to believe strings behave any differently in Unity
they dont, do they?
If they didn't, why would there be a zero width space?
This seems like a very big bug, if true
Sal#0955
REPL Result: Success
Result: string
Compile: 578.966ms | Execution: 46.675ms | React with ❌ to remove this embed.
sounds like more of a potential issue with whatever UI control is being used
it behaves the same way here
Yeah but you're manually adding that to your string. Are you adding a zero width space to your input in unity?
I would argue you are right but they might have their reasons..
I don't think they do, that's a massive flaw
no I am just simulating here...
well I was firstly trying to react if the input box had some value before doing any logic and nothing worked ,
value is null
, value == ""
, string.IsEmptyOrWhiteSpace(value)
until some guys here helped me our and we find the character by parsing the "empty" string to a charWhat're you using as the UI control for the input? You should report this as a bug
the default input box
Unity Forum
TextMesh Pro - [Solved] Getting 'ZERO WIDTH SPACE'
Getting 'ZERO WIDTH SPACE' character at the end of text from Input field
[ATTACH]
Is it ok !?
Does this have anything to do with it?
Also i don't think TMPro is the "default"
gonna see, but i have no other choice than TMPro
it is in the latest version
they removed the old renderer
i think
either way you cant use it
I thought it was like an external library
they aquired it a while ago
hey, so i tried using the child "Text" game object and it returns the same char
The post says not to use the child
first i was using the (input).text, now i tried the Text.text
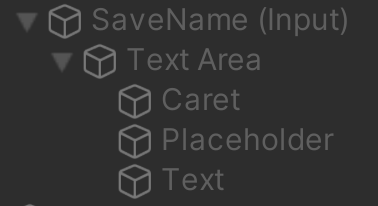
i think those are the only options
I don't know. You're not really showing us much
you dont need much more, what do you want more?
this is the whole tree of the input field, and i am telling you when i get the input text on both gameobjects they both have the char attached to the end
nameHolder
was SaveName (Input)
at first now I tried Text
I think this is absolutely allCan you show what fields exist in the class?
sorry why?
there you go
I mean don't, and just use substring
What do i care
That doesn't look like a TMP_InputField to me
lol if you don't care why you came try to help. I have no intentions to lie. my question was what would be best choice for handling the behavior now we are getting into strange territory
The best choice to handle the behavior is to not have the behavior happen
For that, we need the entire context of what you're doing
So we can tell what not to do
i lay out the entire context.....
Mhm
brand new
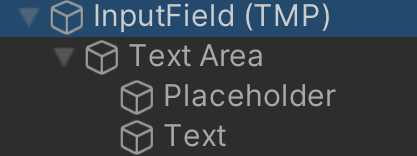
maybe its changed since you used it..
I have never used Unity
then I repeat myself but i am just fetching the value from the input field.
so why did you complain hat it doesnt look like TMP?
the post mentions a
TMP_InputField.text
. you're clearly not getting the text of a TMP_InputField

2018 is not that old
i highly doubt it changed that much, if at all
in unity's case
unity aren't really known to
innovate
and i could call my variable WTV then use WTV.text
calling it a different thing doesn't give it a different type
enough so that they are using TMP as their ui solution now..
@Ero you dont use unity why are you fixing with that?
the post dont even show the type just the name...
[SerializeField] TextMeshProUGUI nameHolder;
not a TMP_InputFieldLOL its new... now you have 3 options...
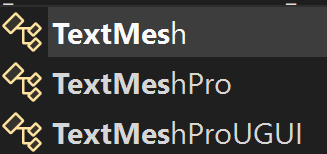
and so far i figured i have to use the UGUI
it's clearly like a base class
there's not even any documentation on this
tf
but i can tell you that if i use the non UGUI i cant assign it in the inspector
idk man, i think i'd use something called an
InputField
for something like an input fieldTMP_InputField
is the input fieldcrazy
yes it does exist such thing x)
and i just tried it works without the char
🤯
maybe don't be like that the next time you ask people to spend time out of their day to help you
i can also recommend google
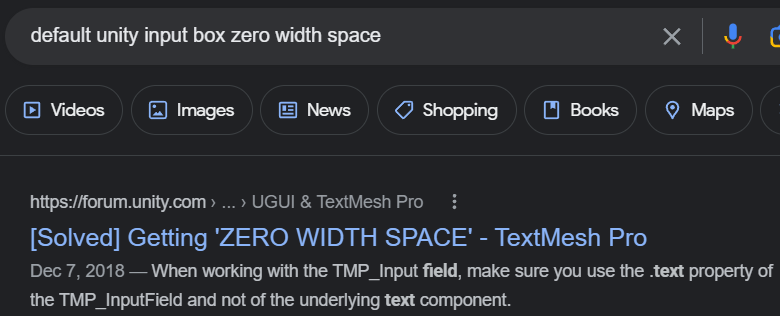
this is literally what i searched to find that post
lol you dont even have to go to the page
i think you are a bit of an arrogant, thanks for the time, but good will doesnt come with strings atached, this is certainly the best solution, but you dont use the software, and you expect that because a 4y old link says something i got to instantly understand that was the type itself that was wrong, i did a lot of learning (not enought, never enough) and sorry if i didnt realize it at first , and doubted your advice. but i tried to follow along. and you just slap like i didnt help the conversation.
he wasnt being arrogant
you couldve tried his suggestion
even if you didnt think it would work
i didnt understand
when i understand i did
then say you dont understand
nothing wrong with that
programming is programming. you don't really need to understand the software to know that a link like that will definitely have valuable information
googling well is just part of coding
whatever problem you have, at least a dozen people have posted about
thats all true, thanks guys
Closed!