❔ Unity3D C-Sharp Create class instance from Type
Hello need a little help trying to create an instance of a type at run time but once I try to create the instance it gets returned null, even after verifying that the time I'm looking for is correct but the System.Activator is not working for me I event tried to use the Assembly
35 Replies
The function that does the conversion
original Class
The method that calls the function
Activator.CreateInstance
does not return null
(unless the type is Nullable<Something>
) so something about how you are debugging things is not right.
I'm not going to read through all that code but if you're saying that Activator.CreateInstance
is returning null then I have my doubts about how you're testing thisi event stepped through the code and everything is correct up to the section of the fist codebase right after
var classes = new List<object>();
everything after that failseverything after that failsYou need to be more specific about what that means Program crashes? You get an exception? You get a null when you don't expect a null?
while stepping as soon as i get to the section that says Activator.createInstance it return null like i said not really sure i'm getting a
MissingMethodException: Constructor on type 'OkashiKami.Terragen.Modifiers.SmoothModifier' not found.
exception but all my classes have a default constructor in them so i really don't understandUm
and i expect when i rand the Activator.CI to return an object of the type that was provided but instead i get a null value when after inspecting the elements with breakpoints all is good but not that part
Well if its throwing an exception then its not returning anything, including null, its throwing an exception
That said...idk, it looks like you have a public default ctor
So that doesnt make sense
yes true one thing i did notice is that the exception doesn't get fired unless i put it in a try catch otherwise it doesn't do anything
You must have something weird going on because activator.createinstance has never had any problems afaik
do you thing it's because those classes inherits a base class in my case Modifier.cs
No
There is no overload of CreateInstance that takes 2 params with a second BindingFlags parameter
So idk what overload that is calling
But very likely not the right one
Remove the binding flags parameter
It's probably looking for a ctor that takes a BindingFlags parameter to pass that into
Because it thinks youre trying to specify that as a ctor argument
This is probably the overload its calling: https://learn.microsoft.com/en-us/dotnet/api/system.activator.createinstance?view=net-7.0#system-activator-createinstance(system-type-system-object())
Activator.CreateInstance Method (System)
Creates an instance of the specified type using the constructor that best matches the specified parameters.
so you know how on msdn they have
CreateInstance(String, String)
Creates an instance of the type whose name is specified, using the named assembly and parameterless constructor.
for some reason that one is not available to meForget that
You have a type
All you need to do is call CreateInstance(type)
Theres no reason for you to be using strings
this is my Activator class looks like
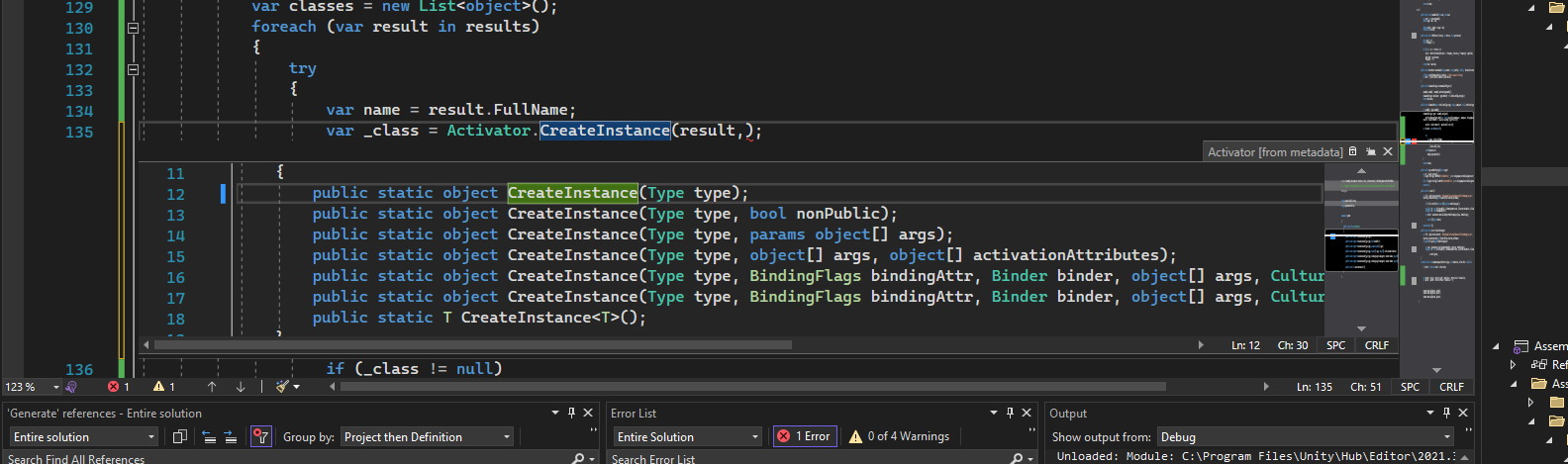
Yes, and what I said stands
let me try with just type
here me stepping through the code
i even show elements as we are stepping
It's not null
If it was then it wouldnt be added to the list
That
'null'
value is something differentIt's showing you properties on the object that are set:
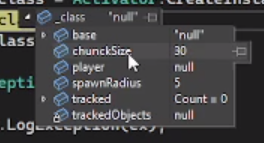
yeah it seams like it's creating and instance of the base class not the actual class of the type but what it inherits from
The
'null'
is what ToString() is returning
Which is probably coming from some base class
I dont see a ToString() override on your classes so its probably coming from UnityObject
Sorry UnityEngine.Object

ah probably
Override
ToString()
on Modifier and return GetType().FullName
And you'll see it'll show the class name
Instead of 'null'
The activation of the types is working fineyour right ugh dam are you serious rn
🙂
Gotta love debugging rabbit holes 😛
We all get stuck in them sometimes
i would of never guess thank you so much for your help very much appreciate it dam much love
I've always wondered about that. 👍
The nullable type annotation never made sense.
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.