❔ Removing JSON data from JSON File
Hey,
I am attempting to remove JSON data from a text file if required (I'm using it as a local database)
This was my attempt:
Obviously, this doesn't work and I receive this error
I know this is because I am directly modifying the collection by removing the pending order - so I am hopefully looking for a better way of doing this. Thanks a lot.
I know this is because I am directly modifying the collection by removing the pending order - so I am hopefully looking for a better way of doing this. Thanks a lot.

11 Replies
Note: I am using Newtonsoft.Json
any reason why you're using newtonsoft?
System.Text.Json is a lot faster (especially in .net 7)
My whole codebase uses it and it was all I was familiar with.
Am using .NET 6
yeah stj is still faster in net6
I will likely look to convert in the future - but for now it's probably too much to change over
Have quite a lot of JSON processing in this project
Appreciate this, I will test this after dinner and report back here!
Hey, so this is exactly how i've implemented this.
Upon running the function, it seems to run successfully however the JSON file still retains the data.
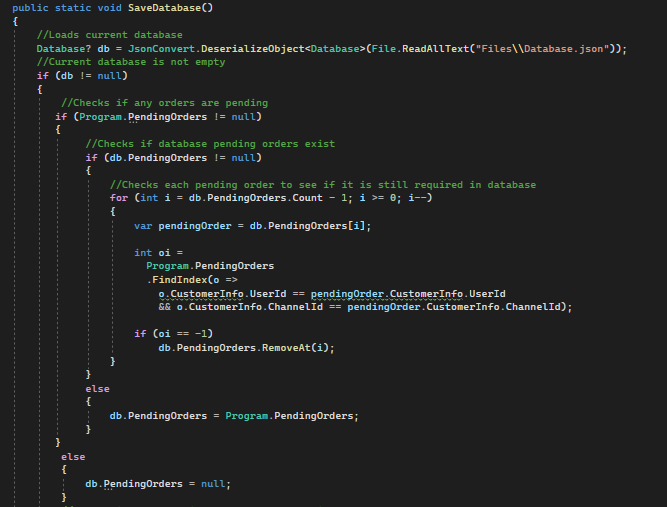

This is right at the end of the void
Resolved! Was fixed by changing the db.PendingOrders = null to a new List
Thanks for your help
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.