112 Replies
So I am stuck with array.sort, I need to sort the array from a text file and then find the median. But I don't think I'm doing the sorting correct...
Array.Sort
sorts an array, you can find the median by getting the middle value.but how would i find median with the array sorting?
Let's take a simple example
We have this set
[1, 2, 3, 4, 5]
what's the median value for that set?3
Great, if we take this set
[2, 3, 4, 5]
What's the median here as well?technically, both 3 and 4?
sorry kinda learning disabled
You're correct, so when the set has an even number of values we take both these numbers add them together and then divide them by two!
So it's (3+4)/2
3.5
Awesome, in your case the number of values in the set (array) is odd, right?
43
so yes
but how would i do a sort with a text 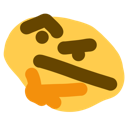
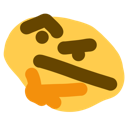
Great, let's break done the code you've written
Initialize the array
Read file and fill the array
The
numbers
array is most likely not sorted, we can however call Array.Sort(numbers)
Which will sort the array in place!is my Array.Sort in the right place??
What do you think?
I think it should be under int total = 0 imo
But the array won't be
filled
yetWhat would be in the array at that point?
so would i put it in after the input file declaration?
Your algorithm should be:
Read all of the data into the array.
Sort the array.
Compute the median.
Where to put the sort should follow from that.
alright, got it
but im still confused on how to find the median 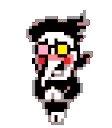
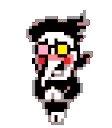
I think @Kouhai already gave a great explanation of how to do that. What part is confusing you?
im also supposed to display what the median is
would i just do Console.WriteLine(Array.Sort(numbers));?
What do you intend that to do?
First, notice that
Array.Sort
returns void
.show the median
So you just asked if you should do:
Console.WriteLine(void);
... oh
Remember, when you call a method you can mentally replace that call with the result that it returns.
im sorry, i'm still a bit confused
like im really slow
as mtreit said
Array.Sort
returns void
, that means it returns nothing
it doesn't make sense to call Console.WriteLine
with nothing, right?That’s right
Okay great, basically
Array.Sort
sorts an array in place, what does in place mean? it means it mutates the array passed to it instead of returning a newly sorted array!But how would I show the result of the sorting?
You only need the median right?
So you don't have to show the whole array!
yep!
how would i show the median then?
Let's ask another question first.
How to show the first element in an array?
uhhh
i wouldnt do a console writeline, right?
Console.WriteLine
as the name suggests just writes something to the console (terminal) and moves the caret to a new line (i.e the next time your write something to the console it'll be on an a new line)
So you can use Console.WriteLine
More importantly how would you go about accessing the first element of the array?numbers[index]?
what's index in this case?
the 43 numbers
But that means the first element is at index 43
oh
im really trying
but i really am having trouble understanding, im sorry
No worries!
Let's take an example
The value
9
is at what index?2
Close, but not quite right
Array's in c# are 0 indexed, meaning the first element starts at index 0
With that info,
9
is at what element now?OHHHH 1
Awesome, what about the index of the last element?
What is it?
Uh
3?
Spot on!
Let's try another array
Where are the first and last elements @Jules ?
0, 1 and 4, 5?
Yup that's exactly right!
So let's say you want to get the
middle
element, what would be it's index?2, 3?
That's indeed correct, now isn't that value the median for our set?
Yes?
Okay, so to get the median of an array with 5 elements, we need to access the 2nd element
Let's assume we have an array of 43 elements
What would be it's median's index?
uhhhh lemme do the math
uhhh i got 22/23
How did you calculate it?
i uh
wrote down 0-43 and crossed like 43, 0, 42, 1
You wrote the numbers from 0 to 43 and then crossed 0, 1, 42, 43?
no
i crossed from 43, 0, 42, and 1
i did 0 to 43 tho
Okay, let's first try a smaller array first, we have an array with 9 elements
Where is the median?
That means your array has 10 elements no?
(4+5)/2 = 4.5?
... OH
Would it be 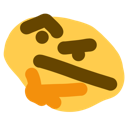
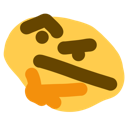
Yup the index would be 4!
Now let's go back to our 43 element array
What's the median's index now?
would it it be 22?
Why would it be 22?
How is it calculated?
uhhhhhh
gimme min
ima redo it
wait
is it 21?
Yes. But why is it 21? Can you come up with a formula?
is it this?
cause im looking over videos as well but im confused
Before code or anything
Mathematically how would you calculate it?
let's say you have 13981 elements, it would be REALLY tedious to do it by hand and cross entries
OHHH thats what youre
i dont know, i only know to cross
which is kinda embarrassing\

😅
Okay, if the set's element count is odd, we can easily calculate it like this
[n+1]/2
where n is the count, but that assumes a set starts at index 1, which isn't true for C#, so how would you fit it with a 0 indexed array?[n+0]/2?
Well, why not give it a try with 43?
[n+43]/2??
Give this one more read
tryna figure it out im sorryu
[43+0]/2?
Is this right?
That's indeed right, that expression could be simplified right?
yeah?
How would you go about simplifying it?
43/2??
Yes correct, not going back to your very first question
How would you apply this information with your program logic to get the median of the array?
You mean the code?
yes!
?
I meant your original code at the start of the thread
Where did a multi-dimensional array suddenly come from in this discussion? 👀
OHHHH
Videos
What do you mean exactly?
My instructors videos… aren’t the greatest :/
I asked her about this a few hours ago before this thread
Well, you just pasted code that seems to be from a completely unrelated problem to the one being discussed here.
All she sent me was this video that had this code on it
That’s why I’m kinda lost
I think I would forget what she sent you for the moment and focus on what @Kouhai has taught you. You might be overcomplicating things.
Probably am overcomplicating things
really sorry
I’m guessing… would it be Array.Sort(numbers[43/2]);?
Or am I wrong..?
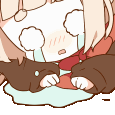
What is
numbers[43/2]
going to return?
Do you know what "indexing into an array" means?It errors
I'm sure it does
You effectively did this:
Does that make any sense? You can't sort a single integer value.
Sort should only be done once on the entire array. Once the array is sorted you can compute the median index and look up the value at that index. That's what your code needs to do:
Create the array
Sort the array
Compute the median index
Fetch the value at that index
Return that value
(Sticking with the simpler case that there are an odd number of values in the array)
Like I understand what you guys are telling me but it’s really hard for me to understand with my code ;__;
Translate the comments into code.
If you are not sure how to do that for one of those comments, ask.
I know you know how to do the first two.
fetching the value, im not sure how to do that
You "index into" the array.
Like this:
If you don't know how to index into an array I might suggest you go back through a basic C# tutorial. We usually recommend $helloworld
Written interactive course https://learn.microsoft.com/en-us/users/dotnet/collections/yz26f8y64n7k07
Videos https://dotnet.microsoft.com/learn/videos
mtreit#6470
REPL Result: Success
Console Output
Compile: 672.460ms | Execution: 43.349ms | React with ❌ to remove this embed.
like.. this?
The fact that you have a variable named
secondString
when your code doesn't use strings at all implies you are just copy pasting code and hoping it will work. Stop doing that and think carefully about what you are doing. Use variable names that make sense for the problem you are working on.
Why did you write this line of code?
You should replace your Console.WriteLine with this:
i did have return
And then figure out how to make the rest of your code create a variable called median with the correct value.
but would it not actually put it in the console like console.writeline?
If you want to print out the result, do that in the code that calls the GetMedian function.
oh
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.
Closed!