❔ How do I pull information out of a function with alot of information?
I'm getting an error in the below statement SPECIFICALLY at keySet with the error "the name 'keySet' does not exist in the current context"
foreach (var iteration in keySet)
{
}
break;
I'm storing the keySet inside of another public function, I'm not sure how to get the information out of that function and into this foreach statement. Heres an example of the other the other function
public void Form1_Load(object sender, EventArgs e)
{
List<keyRing> keySet = new List<keyRing>(); //creating the list of key rings
//creating ALL key rings manually entering information
keySet.Add(new keyRing
{
keyRingIdentifier = "L1.6",
numberOfKeys = 2,
KeyLocation = "storage Building",
keyIdentifier = new[] { "146.23", "72.32" },
keyDescription = new[] { "locker room", "control room" },
});
44 Replies
Do you know where you want to keep the state in this case, should it be part of your class instance, or should it be something you want to pass to the other function as a parameter?
It sounds like this would be something you initialize once and then store in your class for further usage?
If yes, then you should have a List<keyRing> as a field in your class
And store it there
I think I tried that and it gave me another error
let me give it another shot
Basically as it stands, keySet would be immediately out of scope once Form1_Load completes, and it will be cleaned up
The reference is lost
gotch ya
do you think I should store this part within the keySet.cs? I'm getting a ton of errors doing that
I can't tell a lot about how your work looks like, but it sounds like you want to have this stored as part of your class state
Which errors do you get?
(At least since you have it in your form load, that makes me think it is something you initialize once and want to reuse later)
sec
Sounds like you know what's wrong already 👍
I wish I did
Ah
was trying to see if I could copy errors without typing them out
Okay, so it looks like you extracted the keyset, but now you broke the method declaration and your method body is now open
I think what you want might be:
the name 'keySet.Add' does not exist in the current context
internal class keyRing
{
...
List<keyRing> keySet = new List<keyRing>(); public void Form1_Load(object sender, EventArgs e) { //creating ALL key rings manually entering information keySet.Add(...) } } Does that make sense?
List<keyRing> keySet = new List<keyRing>(); public void Form1_Load(object sender, EventArgs e) { //creating ALL key rings manually entering information keySet.Add(...) } } Does that make sense?
just move the public void Form1 into the class file?
Ah, keySet is a different class from your Form
I see
In that case you can just make it a property like the other props in the class
I'm not sure what you mean, but I went ahead and copied the entire function
public void Form1_Load(object sender, EventArgs e){ ... }
and the error inside of the class file is gone
but the error in the main operator is still broken
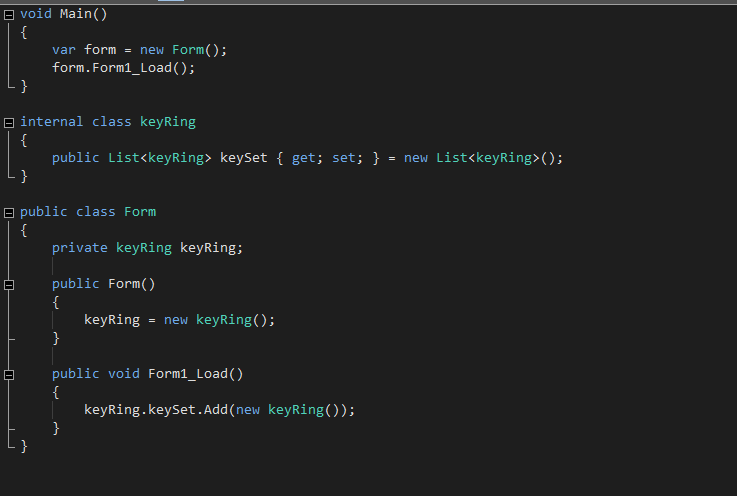
I quickly wrote up an example
If that brings the point across
Ignore the void Main() above, I'm just simulating your situation
should my internal class only have a single line of code?
I can honestly completely circumvent this problem by copying all of the information within
public void Form1_Load(object sender, EventArgs e)
and placing it within my button function
but I just don't understand why I can't get this information outside of other cleaner functions
I don't want to clutter a button
Oh, I was just doing a quick example with the part you are having problems with, so I just skipped the other properties - That wasn't supposed to mean anything
You can for sure
Did my sample help?
not really, I'm still learning so I think I'm a complete novice
Okay, I see. So let me roll up a bit.
Basically you need to have your keySet stored inside a class as a property or field so it does not immediately get out of scope like it does in your initial picture, since you stored it within a method. I'm not sure what your Form1 refers to, but technically you could place it inside there. Another option would be to create a dedicated class, let's say maybe . I think putting it inside keyRing.cs might not be the best idea, since that class seems to contain information about how a keyRing looks like, but it should not necessarily store state itself.
GeeksforGeeks
Scope of Variables in C# - GeeksforGeeks
A Computer Science portal for geeks. It contains well written, well thought and well explained computer science and programming articles, quizzes and practice/competitive programming/company interview Questions.
I think this article could help you understand the issue you are facing
I ended up putting that list within the original namespace
and it fixed everything
Yep, exactly 👍
Wouldn't this make it a global variable?
I have always been told global stuff is bad stuff
Depends on the visibility scope, by default, if not specified otherwise (as in your case), the class member is private, so it is only visible to methods within Form1
If it would be public, everyone could access it
Global state has it's uses, but generally encapsulation is a good pattern to work with, yes
Rule of thumb should be to only expose something if you have a good reason to do so and otherwise start with the least visibility
ty for your help
Yw 👍
Same exact error I think, I really don't understand scope
You are trying to access the property on the List<keySet>
You are already half way there
You're iterating the list in the foreach (var iteration in keySet)
Now you just need to access the iteration itself, which is a keySet instead of a List<keySet>
keyLocation doesn't seem to exist even though it does within the class keyRing
I think you also got your casing wrong
Here it starts uppercase
You're right it was uppercase, I went ahead and changed it to keyLocation
but still same problem
Did you also change it to iteration.keyLocation.Text?
wow
thank you again
I made a program similar to this earlier but I didn't make it look this clean
I've been going off of that but most of my words on that other program are the same
I guess if you learned a bit about scoping that already helps putting things into perspective a lot
its similar anyways
Ty again for your help, I hope I remember this stuff in the future
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.