for next-auth how to include a different model to a User Object
What i want
so that i can attach the user. teacher in the
The goal is to use that teacher object to verify certain things.
Thanks
39 Replies
1:
2:
Oh thanks for the answer, I think i need to be more specific next time
The above code solves the problem which it will include the teacher object but if you take a look at my model
I added a relation to a teacher model
So even i added the teacher object to the session, it will undefined
You need to fetch it from db in your createContext I believe?
Where do i do that @julius
Thanks for helping
Im assuming its here
but it didnt attach it to the
@julius
The useSession uses the object from the session callback you changed previously. But imo I wouldn’t attach to much stuff to the useSession hook
Tried doing it in the callback session also but i dont want to call prisma there haha
Just do a user.me procedure or smth
And you can attach the teacher there
The session object is stored in cookies (I think?) and you don’t want too big object there
Sorry i dont understand the user.me procedure part . Do you have any examples that you can share if you dont mind
Gimme sec at my pc soon
Okay julius take ur time, thanks for helping
This is under trpc router right?
yea i placed it just in the approuter now but if you have a user router that's prob better
consider that the createContext function is called on every request so you want it to be as minimal as possible to not block unnecessary
Okay understood, the thing is i need the teacher object to present in the session globally so that i can use it whenever i can like
in Navbar etc...
so example
Unknown User•3y ago
Message Not Public
Sign In & Join Server To View
Thanks for sharing! but the problem is about relational data, seems like even if you extend the session it wont be included because i believe we need to @Jan Gryga
include : {
teacher: true
}
then only we can access the teacher object, so im still looking aroundUnknown User•3y ago
Message Not Public
Sign In & Join Server To View
You can override the prisma adapter
Small snippet
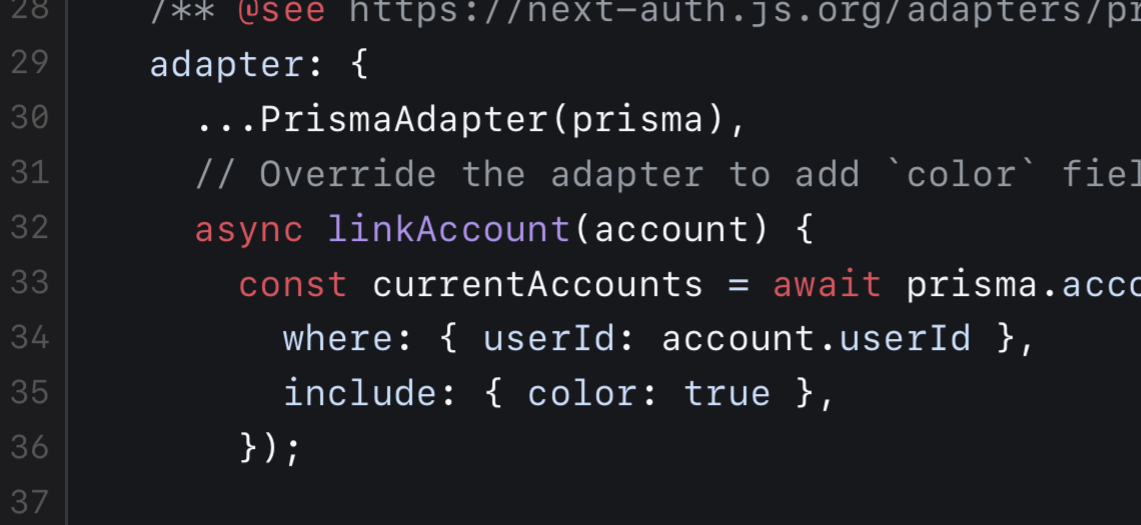
Or include the teacherId in session (should be possible since it’s a primitive?) and fetch the rest in tRPC context
i tried to do the latter where i include the teacherId in session but in my case i have to call prisma there which is not ideal.
but will look into overriding the adapter i think thats what i need
but is there like an official guide to override the adapter? example should i call
linkAccount
somewhere in the code base?
I could not find the official guideUnknown User•3y ago
Message Not Public
Sign In & Join Server To View
no, and it's probably not linkAccount you want to override. what i do is i check the source of the adapter and modify the function slightly. you don't need to call it somewhere - nextauth will do that automatically
https://github.com/nextauthjs/next-auth/blob/main/packages/adapter-prisma/src/index.ts
i'd imagine it's the getUser you want to override
@cje should we include a snippet like this in the docs?
i think it would be helpful
fyi: didn't @ you cause you usually don't like that
ive never seen/used this pattern before, my instinctive response is to keep the session object as small as possible even if it means more queries, but if a lot of people want to do this sort of thing then it might be helpful to put this in the docs
we already have a bunch of other snippets so sure
Unknown User•3y ago
Message Not Public
Sign In & Join Server To View
this isn't about the session only though. it's more if you want additional fields on your account/user etc
oh i don't mind haha
yeah its tough, most of the time you will have to write that code yourself
unfortunately we don't have a better library for auth in nextjs
next-auth is great for "oh i need auth in my project" but it's flaws come to be seen as soon as you want to so something custom and a bit complicated
I think doing this approach will get unexpected behavior
@julius
Why?
You have to do module augmentation too
So the types match
under the node_modules itself?
types/next-auth.d.ts
something like this:
Thanks man for helping!
Unfortunately the teacher attribute is not there and im not sure why
I think getUser is not getting triggered
most likely when i login its calling this function
@julius
https://github.com/nextauthjs/next-auth/blob/8387c78e3fef13350d8a8c6102caeeb05c70a650/packages/adapter-prisma/src/index.ts#L24 the principle is the same, i dont know exactly which method you need to override, maybe this one
include the teacher in that query:
or do this if you feel more comfortable with that. it will trigger 1 extra db call but that's not the end of the world
hahaha you are right
Thanks btw for helping 🙇♂️
@julius is there any chance i can donate to you? the least i could do to contribute
im on github sponsors but don't worry about it. happy to help https://github.com/sponsors/juliusmarminge