❔ Can anyone help me out with a calculation project?
using System;
namespace UppgiftVäxel
{
class Program
{
static void Main(string[] args)
{
Console.WriteLine("Welcome to the app where we calculate " +
"the change for one/ several goods when buying and pay for it");
Console.WriteLine("Enter the paid: ");
int paid = Convert.ToInt32(Console.ReadLine());
Console.WriteLine("Enter the cost money: ");
float cost = Convert.ToSingle(Console.ReadLine());
float change = paid - cost;
int num1000Bills = (int)change / 1000;
change %= 1000;
int num500Bills = (int)change / 500;
change %= 500;
int num100Bills = (int)change / 100;
change %= 100;
int num50Bills = (int)change / 50;
change %= 50;
int num20Bills = (int)change / 20;
change %= 20;
}
}
}
42 Replies
Hey man, sorry for the delay, I was tired last night and missed including the assignment requirement.
I received an assignment from my teacher that I need to build this project.
Write a program that calculates the change you get back when you have paid for your goods. When printing, it is indicated which notes and coins the buyer gets back. The input should be the price to be paid and the amount the buyer has paid. The program must print the number of 1000, 500, 100, 50 and 20 bills and the number of 10, 5 and 1 Swedish cron coins you receive as change.
Divide the task into smaller parts.
1. The program opens with a welcome message and informs that it will calculate change for one/several goods that users buy and pay for.
2. declare the variables needed to count gear.
3. Ask the user to enter the price of the item and read that value
4. Ask the user to enter paid money
5. With a simple rise, calculate the gear back
Don't forget to check the entered values first.
6. Calculate how many notes and coins users should receive.
Use division and modulus for this.
Växel means exchange
Yeah "växel" should be translated to "change" in this case.
Anyway one thing I would point out is that you are asked to divide the task into smaller parts. How have you done that?
No "exchange" means "växla", the verb.
I forgot to include this too
We assume that 50 öre is still used. The exchange rate is rounded to the nearest 50 öre. If the number of öre is < 25, the change is rounded down to 0, if it is between 25 and 75, the change is rounded to 50 öre, and if it is >75, the change is rounded up to a krone.
I hope you got the idea
Some form of bankers rounding then.
Kind of
Then another problem with your solution is how the user should enter "öre"/pennies.
They could be paying 1.5 crowns for something also.
Yes that's what I am saying, it is one part of the problem.
using System;
namespace UppgiftVäxel
{
class Program
{
static void Main(string[] args)
{
Console.WriteLine("Welcome to the app where we calculate " +
"the change for one/ several goods when buying and pay for it");
Console.WriteLine("Enter the paid: ");
int paid = Convert.ToInt32(Console.ReadLine());
Console.WriteLine("Enter the cost money: ");
float cost = Convert.ToSingle(Console.ReadLine());
float change = paid - cost;
int num1000Bills = (int)change / 1000;
change %= 1000;
int num500Bills = (int)change / 500;
change %= 500;
int num100Bills = (int)change / 100;
change %= 100;
int num50Bills = (int)change / 50;
change %= 50;
int num20Bills = (int)change / 20;
change %= 20;
}
}
}
No actually, I missed it.
You mean this the number of 10, 5 and 1 Swedish cron coins you receive as change.
I will point out again that the assignment asks you to divide the task into smaller parts.
Well! How do we do that then.
Good question! <a:raeClap_GIF:594205606705889281> What parts do you think there are to the task?
I think it's hard and complicated task for a beginner like me 🤷🏻♂️
Good question! I have to think about it 🤔
It is, I'm not sure how to solve it myself and I'm not a beginner. That is why you should divide it into smaller parts!
I applied recently for a course and this is first assignment that I got, very strange.
How long have you been in coding with C#?
Professionally, just a year and a half. A couple of more years in my spare time. Sorta.
Ok! Great. You've been in this field for a while then.
Sure, but I still don't know anything!
that is mostly because I'm dumb! 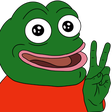

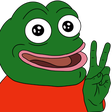
Really! Almost like me hahaha
I can help you with this assignment though, if you want.
I set at my MacBook for hours, but still not learning a lot and not progressing in coding.
Great
Well, it is hard to learn anything. Mostly because you have to learn to accept failure.
Yeah! I totally agree.
Good, good and you are willing to do that? Failing I mean?
If you do, this assignment should be no trouble to actually solve!
The hard part is dividing it into smaller parts. If you can do that the other things should fall into place.
Of course. I don't like to give up att all. Regardless the complexity of coding, I will thrive until I learn this skill.
Yeah! I totally agree.
That is the spirit! <a:raeClap_GIF:594205606705889281>
We start off with the first divided part.
Yep
You can start of anywhere really. Are you familiar with how functions/methods works?
These should be the divided steps, right?
Divide the task into smaller parts.
1. The program opens with a welcome message and informs that it will calculate change for one/several goods that users buy and pay for.
2. declare the variables needed to count gear.
3. Ask the user to enter the price of the item and read that value
4. Ask the user to enter paid money
5. With a simple rise, calculate the gear back
Don't forget to check the entered values first.
6. Calculate how many notes and coins users should receive.
Use division and modulus for this.
AlmostI believe what they want you to do when they ask you to divide the task into smaller part is to use functions to divide the program.
Ok
What kind of function would that be?
By the way, what is the Swedish for number 5?
A function that you make yourself.
So for instance, you could make a function that calculates how much change there is.
So instead of
you would have
Well 5 in Swedish is fem, but if you mean as coins it's 5 crowns.
I meant, what is the Swedish for point 5, "5. With a simple rise, calculate the gear back
Don't forget to check the entered values first"? I'm Swedish too.
I'm not really sure what this means?
Do you think a call would be a good method to build this project?
I do not understand what "With a simple rise, calculate the gear back" means, so I wondered if you could provided the untranslated text?
Ok
If by "call" mean a "function call", then yes. I think that that is what your assignment is implying that you should do.
I could do that
Usually functions are one of the first things you start learning.
They are key to most programming languages.
Skriv ett program som beräknar växeln man får tillbaka när man har betalat för sina varor. Vid utskriften anges vilka sedlar och mynt köparen får tillbaka. Inmatningen ska vara priset som ska betalas och beloppet köparen har betalat. Programmet ska skriva ut antal 1000-, 500-, 100-, 50- och 20- sedlar och antal 10-, 5-, 1- kronorsmynt man får som växel.
Vi utgår från att 50 öre fortfarande används. Växeln avrundas till närmsta 50 öre. Om antalet öre är < 25 avrundas växeln neråt till 0, om det ligger mellan 25 och 75 avrundas växeln till 50 öre och om det är >75 avrundas växeln upp till en krona.
Tips! Använd modulo-operatorn (%) som ger resten vid heltalsdivision.
OBS! Skriv din dokumentation och utvärdering av din lösning som kommentarer i källkodsfilen eller om det behövs, i ett separat textdokument (som du i så fall skickar med i samma zip-fil).
Ok! Yeah! You're right. But what I meant by "call" I meant calling you & talking on here in the server 🤗
Oh, I see, sure you can call me.
Great
How do I call you? I don't see any option!
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.