Help refactor please? I might have missed something big here [Answered]
is there maybe a better way of writing this?
The idea here is that I have a list of an object, of which one of its properties (or fields) is
Methods
and I've got a second list of strings, which are the Tags
I got to find the matching strings between them (with fuzzy preferably)
here's an example list of the two:
Basically I have to create a method that calculates the matching "tags" so to speak between the two lists.
Oh, additional note: I made a class MatchingTags that looks like this (just because I need both properties in the rest of the program)
60 Replies
Unknown Userā¢3y ago
Message Not Public
Sign In & Join Server To View
yea I'm thinking Linq too š
Unknown Userā¢3y ago
Message Not Public
Sign In & Join Server To View
I'm thinking I still follow š
but I have to make a conditional for the tags and methods too:
string.Equals(tag, method, StringComparison.CurrentCultureIgnoreCase) || tag.ToLower().Contains(method.ToLower()) || method.ToLower().Contains(tag.ToLower())
for the Matching
property, and I have to select the method that is actually in the Tags
list
š
hence I get confused
afterward I'm calculating a percentage based on this list too
but that was easyUnknown Userā¢3y ago
Message Not Public
Sign In & Join Server To View
the contains is supposed to be my "fuzzy" comparison
Unknown Userā¢3y ago
Message Not Public
Sign In & Join Server To View
dude š
I think you lost me. I'm still trying to figure out how to get both tags and methods matching up with the linq you sent
Unknown Userā¢3y ago
Message Not Public
Sign In & Join Server To View
whelp, hang on. Lemme try and figure out what you made there
IndexOf
returns an int... you know this right?
also this part:
is not valid C# (according to Rider)
it gives me this:
Unknown Userā¢3y ago
Message Not Public
Sign In & Join Server To View
but if this
IndexOf >= 0
doesnt' that pretty much always make it true?
I guess I don't know how IndexOf
works then š
I'll read up on it loltebeco#0205
REPL Result: Success
Result: int
Compile: 452.686ms | Execution: 25.759ms | React with ā to remove this embed.
tebeco#0205
REPL Result: Success
Result: int
Compile: 536.815ms | Execution: 25.470ms | React with ā to remove this embed.
fair enough
tebeco#0205
REPL Result: Success
Result: int
Compile: 456.006ms | Execution: 19.065ms | React with ā to remove this embed.
tebeco#0205
REPL Result: Success
Result: int
Compile: 525.744ms | Execution: 38.151ms | React with ā to remove this embed.
wait, how is the last one no match? while it ignores case?
Unknown Userā¢3y ago
Message Not Public
Sign In & Join Server To View
what if the tag says
Function Azure
and the method says Azure Function
does that still make it true then?Unknown Userā¢3y ago
Message Not Public
Sign In & Join Server To View
Oh, in that case
how did this command work?
Unknown Userā¢3y ago
Message Not Public
Sign In & Join Server To View
So that would be a false, but I need that to be a true
because it's basically the same, but written different.
Unknown Userā¢3y ago
Message Not Public
Sign In & Join Server To View
Also, this is still not entirely valid because of the error I shown before (plus you forgot the
Select( tag => new XXX() ...
part (the tag)Unknown Userā¢3y ago
Message Not Public
Sign In & Join Server To View
no, the tag part
Unknown Userā¢3y ago
Message Not Public
Sign In & Join Server To View
okay, hang on. Let me grab a working list of both
Unknown Userā¢3y ago
Message Not Public
Sign In & Join Server To View
Assignment.Tags
(Don't know why it added the spaces in front, but this is from the Database, next up is my "Methods" list)
Unknown Userā¢3y ago
Message Not Public
Sign In & Join Server To View
what I meant you forgot is this >
Select( tag => new() ....
you had this: Select(new() ...
it could not resolve tag.IndexOf(...
Unknown Userā¢3y ago
Message Not Public
Sign In & Join Server To View
What's with the
false ||
lolExperience.Methods (from multiple Experiences)
lol is okay
Unknown Userā¢3y ago
Message Not Public
Sign In & Join Server To View
Eugh
Wow i hate that
Unknown Userā¢3y ago
Message Not Public
Sign In & Join Server To View
I'm still getting this error:
at the last
SelectMany
Unknown Userā¢3y ago
Message Not Public
Sign In & Join Server To View
If your code is too long, you can post to https://paste.mod.gg/ and copy the link into chat for others to see your shared code!
I'll try to, give me a minute š
Unknown Userā¢3y ago
Message Not Public
Sign In & Join Server To View
paste.mod.gg doesn't need to be single file
You can have up to 5 tabs
I'm getting you the paste.mod
tebeco#0205
REPL Result: Success
Console Output
More...
Compile: 774.203ms | Execution: 138.220ms | React with ā to remove this embed.
Unknown Userā¢3y ago
Message Not Public
Sign In & Join Server To View
Unknown Userā¢3y ago
Message Not Public
Sign In & Join Server To View
what I'm getting now is a much too long list of items though š
the list seems to keep on going, adding the same tags five or six times
Unknown Userā¢3y ago
Message Not Public
Sign In & Join Server To View
Ooh, no, I figured it out š
it lists every tag the user has in his Experiences items š
I think it actually works now š
your help with Linq was awesome, thanks for that
it was this bit that messed up the rest afterward
but this is the fixed version, and it lists everything awesomely
Just the percentage bit needs to be fixed
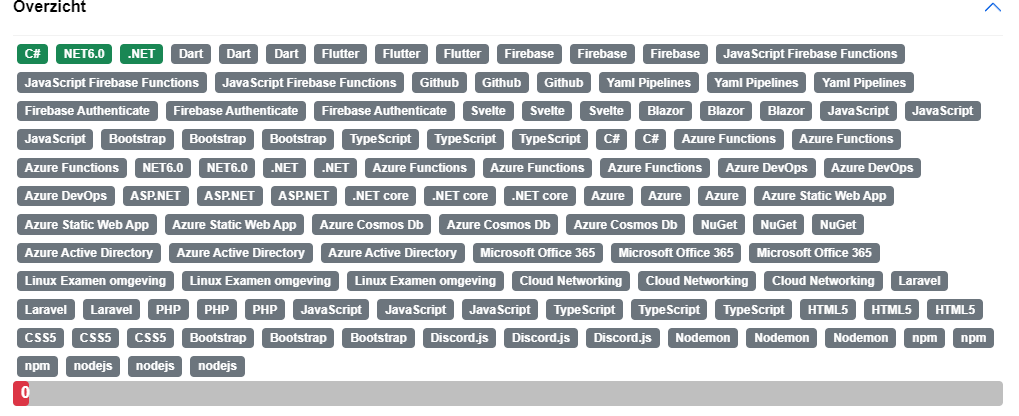
Unknown Userā¢3y ago
Message Not Public
Sign In & Join Server To View
Don't even get me started on why the LIst š
my colleage has been messing things up, and I'm here now to fix it lol. So let's not go there in this topic shall we?
Unknown Userā¢3y ago
Message Not Public
Sign In & Join Server To View
Thanks!
ā
This post has been marked as answered!