Typeof question
why this is coming out as an object?
let person = ["cheese", "hot"];
console.log(typeof person === "object"); <---- true
console.log(this is any array? ${typeof person === "array"}
); <---- false``5 Replies
Because everything in JavaScript is an object :p
Also, if you use three backticks (```) it'll give you code blocks (see #How To Ask Good Questions for more on codeblocks).
More specifically, anything that's not considered a primitive data type is a
typeof
object.
The following are primitive data types (those that are passed by value and not by reference)
• string
• number
• bigint
• boolean
• undefined
• symbol
• null
If you want to know what exact type something is, you can use this snippet:
The Object.prototype.toString.call([data])
will give you [Object [Type]]
and the slice grabs just the type and makes it lowercase.
@interactive-harp or if want to just check if it is an array or not you can use
Array.isArray(person)
thanks, another question
this is my array:
name: 'Mr. White', plea: 'wouldShe', diary: 'diary' ] 2 length is 2, shouldn't it be 5?
let person = ["cheese", "hot"];
let plea = "wouldShe";
person.name = "Mr. White";
person.plea = plea;
person["diary"] = "diary";
console.log(person);
console.log(person.length)
This is the output:
[
'cheese',
'hot',name: 'Mr. White', plea: 'wouldShe', diary: 'diary' ] 2 length is 2, shouldn't it be 5?
The actual output is:
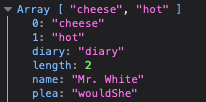
The length is 2 because that's how many items are in the array
Remember that everything in JS is an object, so your array now also has some object keys/values in addition the the 2 items in the array (and that's all that
.length
measures, the number of items in the array)
The items prefixed with a number are the array indexes. This is digging into the weeds a bit, but when they say everything is an object everything is an object. An array isn't actually an array, it's an object with a length
property and each item in the "array" is a property on the "array" object with a key of the "array" index
Also, please use code blocks instead of inlining the formatting like that
Start you code blocks with this:
```javascript
// code goes here
```
And you can replace javascript
with almost any programming language to use appropriate text highlighting (on desktop). Like HTML, CSS, Go, Python, etc.