Per-Page Layouts in T3
Hey guys I was attempting to implement per page layouts on the t3 starter template as shown here:
https://nextjs.org/docs/basic-features/layouts#with-typescript
I kept running into Typescript issues. Any way I could go about this in a way I could make Typescript happy?
There is a squiggly line on
MyApp
and the getLayout
function argument in the return.
Feel free to suggest a better approach for this.
Basic Features: Layouts | Next.js
Learn how to share components and state between Next.js pages with Layouts.
14 Replies
Unknown User•3y ago
Message Not Public
Sign In & Join Server To View
The one above is for the MyApp declaration squiggly Line
the one on the return
getLayout
call argument is this
Unknown User•3y ago
Message Not Public
Sign In & Join Server To View
got it working like this
I added a default
MainLayout
for all the routes that can be overridden by every Page Component with the getLayout
like so:
Ahaaaaaa thanks Man works for me too ...
Awesome, today I was struggling with the same lol
Dope stuff ..... Thank you sooo much for the work around
sure, if you are curious about the full implementation here it's, good luck 👍 https://github.com/esponges/t3-ecommerce
Unknown User•3y ago
Message Not Public
Sign In & Join Server To View
hmm I have checked and
session
is taking Session | null
as types, the compiler took some secs to update it btw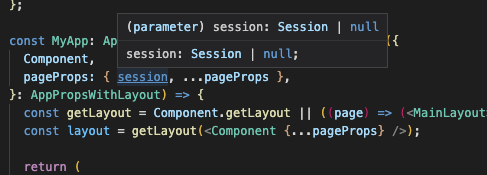
Unknown User•3y ago
Message Not Public
Sign In & Join Server To View
This seems to work, no guarantee
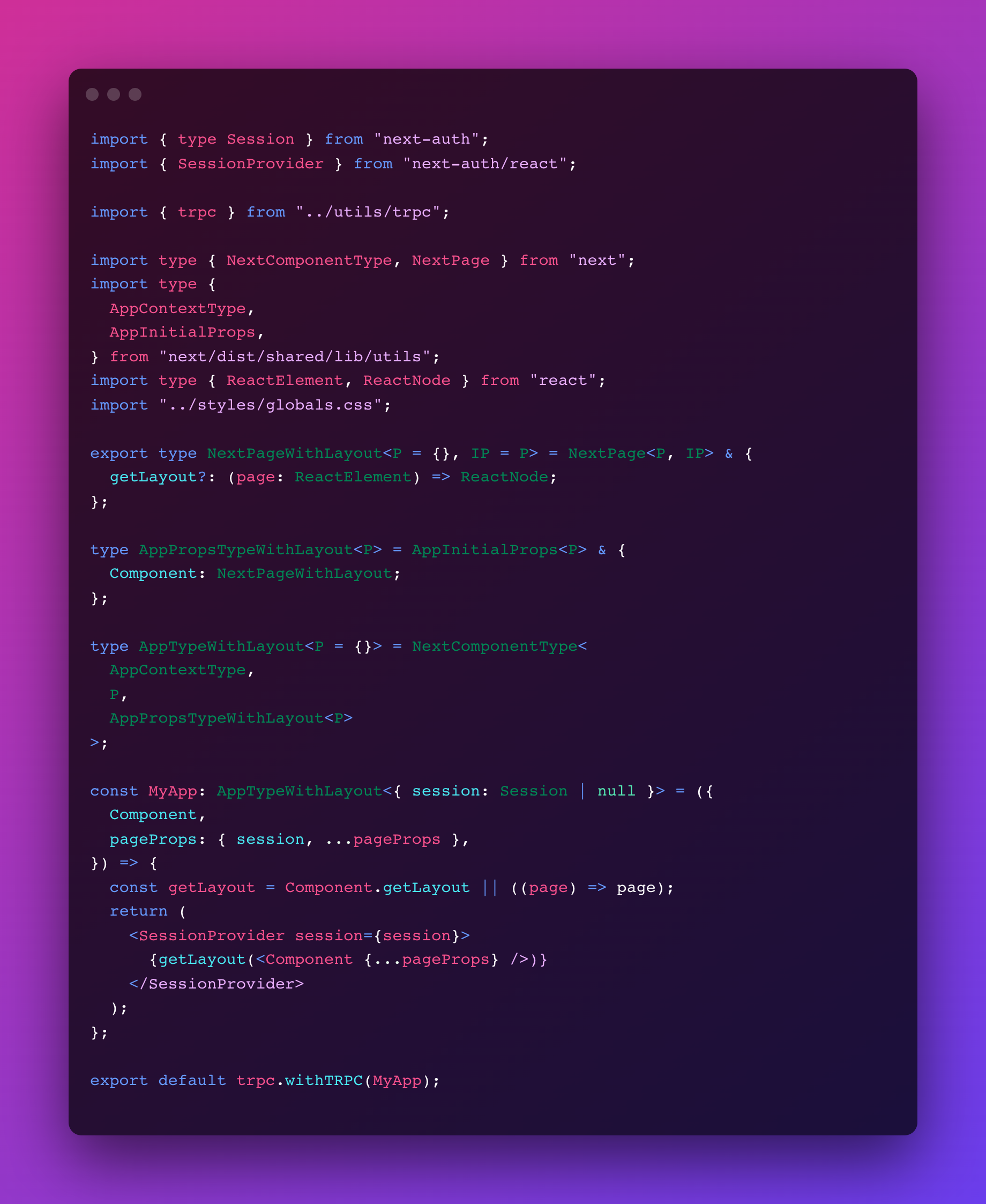
Unknown User•3y ago
Message Not Public
Sign In & Join Server To View
@Mik3y-F What's the benefits of using this .getLayout approach compare to wrapping each page content with some layout component?