Levenshtein distance method throwing `IndexOutOfRangeException` [Answered]
I have this code to calculate levenshtein distance:
When
string1
is empty and string2
is "Example", it works.
When string1
is "Ex" and string2
is "Example", it throws an IndexOutOfRangeException
.21 Replies
Which line throws the exception?
I don't remember how to find that
VS should highlight the line in yellow when the exception is thrown. It will also be in the stack trace
It doesn't highlight it in yellow, but it shows up in the Debug output
What exactly do you see in the debug output?
Exception thrown: 'System.IndexOutOfRangeException' in RainBOT.dll
Is something higher up catching the exception?
The method is being run in an async task if that could be causing the issue
I'm guessing it's not being awaited?
Debug -> Windows -> Exception Settings, change the little checkbox next to "Common Language Exceptions" from grey to a tick. Don't get confused by "C++ Exceptions" or "Win32 Exceptions"
(when you're done here, set it back with this button: )

It's already on

That's the one
Click it until it's a tick
Ok
That'll make it break when the exception happens
and you can debug it from there
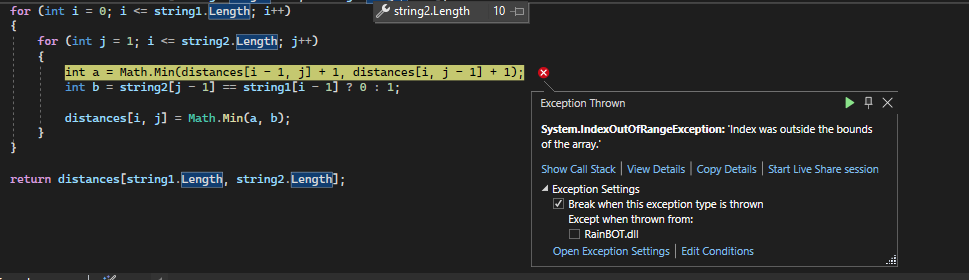
There you go
Now you can inspect those variables and arrays, see what's out of bounds and why
Thanks
👍
✅ This post has been marked as answered!