How to remove this listener in another file?
Hello,
So I have set up a listener like this:
notify.js
obviously includes the function that should get triggered once the voice state of the specific user updates.
Now I want to remove that listener again in another file later on. Issue is that I have no idea how to do that.
Can someone help?21 Replies
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
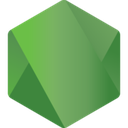
nodeEventTarget.removeListener(type, listener)
Node.js-specific extension to the EventTarget
class that removes the listener
for the given type
. The only difference between removeListener()
and removeEventListener()
is that removeListener()
will return a reference to the EventTarget
.@jaw0r3k I know that function but it doesn't work. Or at least I'm applying it incorrectly. That's what I have:
So, I just went the brutal way and used
removeAllListeners
. But still have an issue. The notify
-function uses the client
variable but when I try to pass it like this:
It will be undefined
in the notify
-functionUnknown User•2y ago
Message Not Public
Sign In & Join Server To View
Means?
oldState.client
and newState.client
both exist
I dont think you need to call the function to remove it
You would do client.removeListener("voiceStateUpdate", notify)
Doesn't seem to work
That should, because you're maintaining a single reference to the function
So it knows exactly what its adding/removing
That's how I remove it:
The bot still reacts to my
voiceStateUpdate
-event. When I use the line above, it doesn'tAnd how is it added?
yeah, that isnt the same function
As soon as you do () => notify, you're declaring a new anonymous function
There's really no need to add client and user, you can access those via
newState.client
and newState.member.user
Ok, I changed that
But when I add it like that:
It trows an error of
oldState is not defined
Please, look at the code I provided
You do not need to call the function
Ah, got it
By passing the variable it calls it for you
Yeah, makes sense...😅
Thank you a lot!
No worries
Another question: Do you know why it calls the function on
voiceStateUpdate
once for each user that the bot listens to?
E.g.: This bot sends a message each time I start to stream or turn on the camera. Now, when I register someone else and the bot starts to listen to it, it triggers the notify
-function twice, 3 times if I add another one and so on, but always for just that one user that initially triggered the eventThats just how Discord works
And listeners
As soon as you bind one voiceStateUpdate listener it will emit for every user that updates
Theres no such thing a single-user listeners
Hmm, ok. I have to implement one other thing anyway. I guess that'll fix this issue aswell. Thanks
Just to let you know how I fixed it all:
When the person first started the stream, I set their "Live State" to
true
in a Collection and then checked that if the person's Live State is set to true, I just returned:
Then I reset that value once the user left or switched the Voice Channel: