Where does LinQ store its IEnumerable selections?
Say I have the following sample code
Looking at the debugger, you can see that both iterB and iterC are null.
Where exactly does LinQ store its queried data?
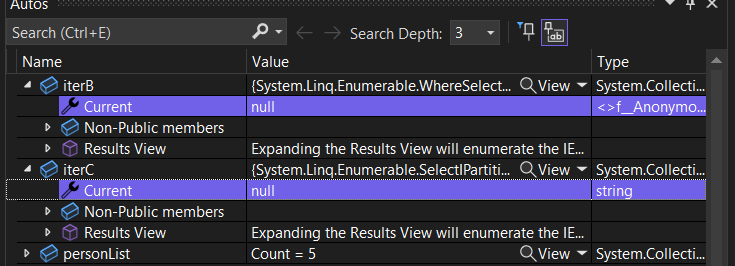
6 Replies
Linq doesn't materialize any data until you explicitly request it
So it's not stored anywhere, it's generated on the fly when you iterate the IEnumerable.
A Linq query is merely a hierarchy of instructions on how to transform an underlying enumerable.
Essentially a Linq query returns a structure like this
That original collection may be an IEnumerable generated by another Linq query, thus you can chain them.
Don't get too hung up on linq.
Where
for instance is essentially as simply as:
(There's some extra error handling and optimizations, but that's essentially it)yep
And note the special syntax here,
yield
again doesn't materialize anything until it's enumerated.Yeah, if you're not comfortable with
yield return
and enumerators/etc, I'd recommend getting comfortable with those before starting on linqI'm more worried about troubleshooting
Because the data should be located somewhere in memory, otherwise how is my method even outputting anything
The next element in the sequence is generated on the next iteration of your foreach loop
That's why I said to get comfortable with
yield return
first