Testcontainers must override configuration before it runs the Web API, but it does the opposite.
I'm trying to create an integration test for a Web API whose communication is based on Redis.
The issue is that
TestcontainersBuilder
is first running the project with what's in appsettings.Development.json instead of the overriden configuration by builder.ConfigureAppConfiguration
. In other words, the sequence is messed up. Instead of directly running the Redis container, it is first trying to connect to the old IConnectionMultiplexer
with the old configuration and then it is supposed to replace that IConnectionMultiplexer
with the new one but it makes no sense because it's "the old one" is locally installed and currently not running. The sequence should be 1) override configuration 2) run the web API.
I have services e.g. AddFtxServices
, AddBinanceServices
that I dependency inject based on the configuration which I cannot simply "replace" later on because apparently that's how builder.ConfigureAppConfiguration
works, unless there is a workaround that I'm aware of.14 Replies
you can use ConfigureHostConfiguration
@Wz, but that's part of
IHostBuilder
https://learn.microsoft.com/en-us/dotnet/api/microsoft.extensions.hosting.hostbuilder.configurehostconfiguration?view=dotnet-plat-ext-6.0
protected override void ConfigureWebHost(IWebHostBuilder builder)
that's what i can overrideoverride CreateHost
GitHub
.net6 minimal API test config overrides only apply after app has be...
Describe the bug Using .net 6 minimal APIs and WebApplicationFactory for testing, the test config override is only applied after .Build. This means any interaction with config while configuring ser...
@Wz thanks, it got applied here
unfortunately, it didn't affect
what is suppose to affect AddFtxServices?
@Wz there are exchange configurations in the appsettings such as this one
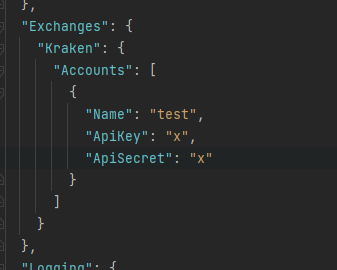
the issue is that appsettings.Development.json defines Kraken configuration and the test one actually uses a different one (ftx)
it resolves the kraken one instead of ftx
You have to add that json specifically
Inside ConfigureHostConfiguration
I actually do
Did you tried absolute path?
GitHub
Unable to load/reference appsettings.json when integration testing ...
Hi there 👋 I'm trying to do a basic integration test for a very simple ASP.NET Core WebApi project. I'm leveraging the WebApplicationFactory class and when I do this, my appsettings...
@Wz
@Wz it works great
thank you very much! 🙂