ASP NET Core 6 Launch without Debugging doesn't block Background Service but Debug Does
Hi,
I'm trying to have a ASP.NET Core BackgroundService run really in background, i mean really don't interrupt with request/response part and I've been trying many stuff
That is the code i currently have, and one weird thing is if I debug the code, the service blocks the program execution, while if I use run, it doesn't block and continue as expected.
Is there any mistakes there?
Also, is there other ways to do this? I tried Task.Run(), Task.Factory.StartNew() and they all block.
I'm on Jetbrains Rider on Ubuntu 20.04 if that matters for the threading part.
Thanks in advance!
91 Replies
There's no way to achieve that, all threads are halted when reaching a breakpoint
ah it's not that i put any breakpoints there
i meant this buttons
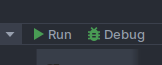
If i started it with Debug, it never made it to the next service
but it works correctly with Run
I have been investigating for a few hours and with Jetbrains' IDE i found that it doesn't get to the TryExecuteBackgroundServiceAsync() and the foreach loop stucks
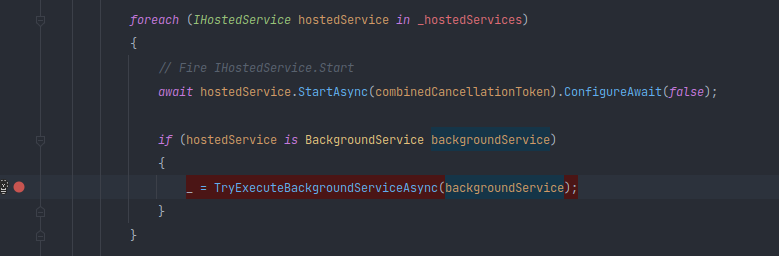
That's weird, do you have specific code that runs for development vs production builds?
Does the foreach loop even execute or is _hostedServices empty?
it is not empty
I have 2 background services and with the debugger breakpoint it didn't evaluate to true for the first background service (this one)
also i dont have any specific code that runs on different configuration
does hostedService.StartAsync await infinitely? (or it's not even reached)
at least not in my own code (non library), and not in this service
does it refer to this backgroundservice?
it is reached
it does have infinite loop
I have updated the code to this
ooh
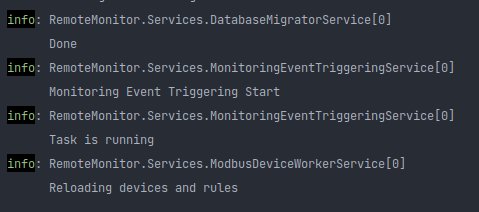
now wonder if it blocks somewhere else
but according to the debugger it doesnt even get to the second backgroundservice i have
Based on these logs, I assume both services seem to be started
i will try putting breakpoint again
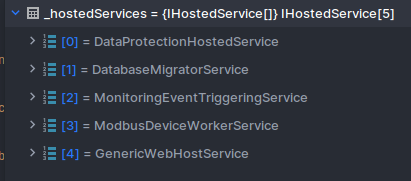
these are the hostedServices
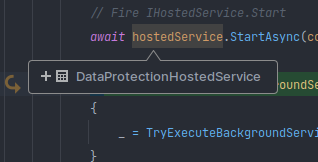
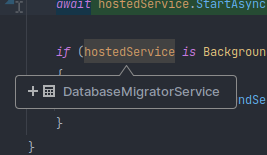
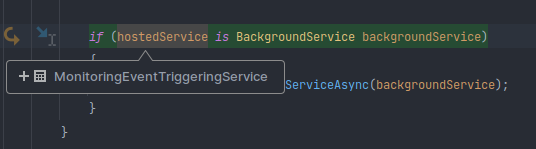
the next step after the last picture is nowhere
and the log is also stuck here
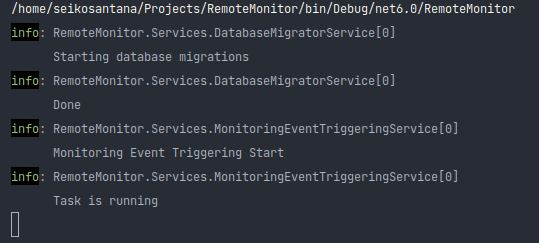
that was Host.cs, class Host in Microsoft.Extensions.Hosting.Internal
but i mean even having breakpoints vs no breakpoints on debug has different result?
Hmm so it's stuck after calling
StartAsync
on the MonitoringEventTriggeringService
, I'm not sure if Host.cs has any conditions for production vs development but I doubt thatit does get to
and then next step is nowhere
Oh
okay just tried it again
this time the next step was the closing curly brackets of the foreach loop, as if the hostedService is not BackgroundService
got to the next enumeration (is it?) in the
in _hostedService
and then next step is nowhereThe condition
hostedService is BackgroundService backgroundServic
fails?
Hmm even if it fails it should continue loopingjust tried again
it didnt continue after the hostedService is BackgroundService part
i mean even if it fails, it may be throwing something right?
But what would throw?
is T
just checks if the instance is of that type, if so it would run the code inside the braceswhile it does inherit from BackgroundService
idk if it would help, but what version of ASP.NET Core are you using
ASP.NET Core MVC 6
maybe i should also try it on Windows and see if it happens
This SS is for the release build, right 

nope
Okay..in that pic it
ModBus
is run normallyif i didnt put breakpoint at all, even with Debug, it reaches that part
it does start, but it doesnt run normally
like it reaches some point and not continue
but if i put some breakpoints and it hit, it doesnt get to even initiate the next service
and sometimes it doesnt get to that part of log too (ends on Task is running) on debug
Can you do something real quick, wrap all your code that uses
Task.Run
with try catch, and in catch log any messagemmmm
wrap in the Task.Run() delegate?
so like
Yeah
Do you have any other service/s that calls
Task.Run
?i dont
I have also commented out other services
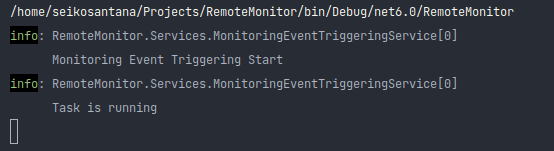
still it didnt make it to the web service
Hitting the pause button on debug doesnt even pause it
Ctrl + C doesnt shut it down
You can replace
with
right?
Yeah, HostedServices are singletons by default
but I wasnt able to resolve it on the other service without registering it as singleton
also I now have this instead to try it out:
still stuck there
that's a lot of commented out code :)
yeah
but what's the problem? You don't see console output from the task?
Nope
you might want to put a timer in the
while
, not to check EventQueue.Count
every nano secondthe app seems seems to be stuck because this service
yeah doing this works, like putting a Task.Delay() and await it
that works but is not as robust, the
PeriodicTimer
is really good for these use casesbut still it is weird that if I start the app without debugging it, it works
run without debugging
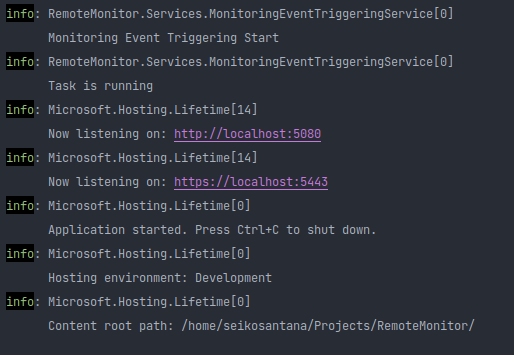
but you shouldn't have to make a thread or task in your ExecuteAsync
meanwhile run with debugging
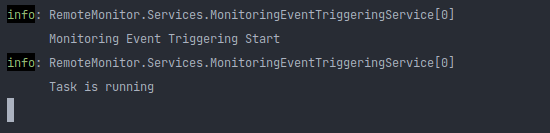
mmmm
hmm
are you hitting a break point during debugging?
i dont
but i mean if the host is implemented this way, and the service is background service, then it shouldn't be blocking right?
yeah I think it's not blocking
it should create a thread for the background service
even if i create a thread, with IsBackground set to true
it still blocks
hmmm
but not if i start without debugging
still no luck,
i tried with break when any exception is thrown
also did another try with mute breakpoints
didnt see any clue
hmmm, can you try adding a log message at the top of Task.Run delegate?
sure
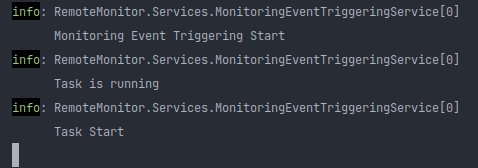
also tried with
and it's spamming Task alive
That's really weird... 😅
I'm honestly lost at what could cause that....
using logger to log Task alive blocks
but weirdly i have that Console.Write(".")
that somehow caused it not to block

But thanks a lot for at least having a look
where did you write the
.
?
in what line of code?
but Monitoring Event Triggering Start
or Already running
is not logged?In the while loop
It is, everything is logged, just covered with the dot spam from that service
try adding a
await Task.Yield();
in the while loop, I'm curiousWill try when i'm back to linux
Now i'm trying in Windows and i can debug
But my Rider version is a few version older
Updating it
.NET 6 version is also a few version older on my windows machine
anyway i also tried this thing before on linux:
I started it without debugging, then attach the debugger on the currently running ASP.NET Core 6 app with this same, then attach the debugger
And the service stops
updated Rider and SDK version on Windows and it still can debug
Seems to be linux specific
WOW

tried this
await the Task.Yield
oh sorry
seems to be the same
but having it in the while loop helps
I'm using
await Task.Yield()
for nowI also opened an issue at Github
https://github.com/dotnet/aspnetcore/issues/44706
GitHub
Launch Without Debugging Doesn't Block BackgroundService and Whole ...
Is there an existing issue for this? I have searched the existing issues Describe the bug I have a BackgroundService that looks like this using RemoteMonitor.Classes; using RemoteMonitor.DatabaseCo...
It seems like it's a runtime problem
Even a very simple code like this
Halts after the first Task is started
I tested it on WSL running Debian distro
I see
even without ASP.NET Core 6?
Yup, just a very simple console app
i see
if you don't mind i'll include these as well into the issue
Yeah sure, go ahead
thanks
It was also "fixed" by using await Task.Delay or await Task.Yield, so it seems like infinite loops makes something break on Linux
well not so infinite on my previous code, at least checks for
stoppingToken
True, but practically it was infinite, because stoppingToken doesn't cancel before the whole program halts
ah yeah