.NET MongoDB Driver - Unsupported Filter Contains
So here's my method in my repository layer... which basically checks: if there were existing questions (it's an interview app for company recruiters) delete only those whose
Practice
property is equal to ANY of the ones that came in the request payload.
The method DeleteMany
calls this function:
Which calls this function from the MongoDB Driver (seems to be version 2.17.1.0):
The problem is that I'm getting an exception related to the use of .Contains
in my filter. Why is this? How would I rewrite the filter expression to avoid using it and achieve the same results?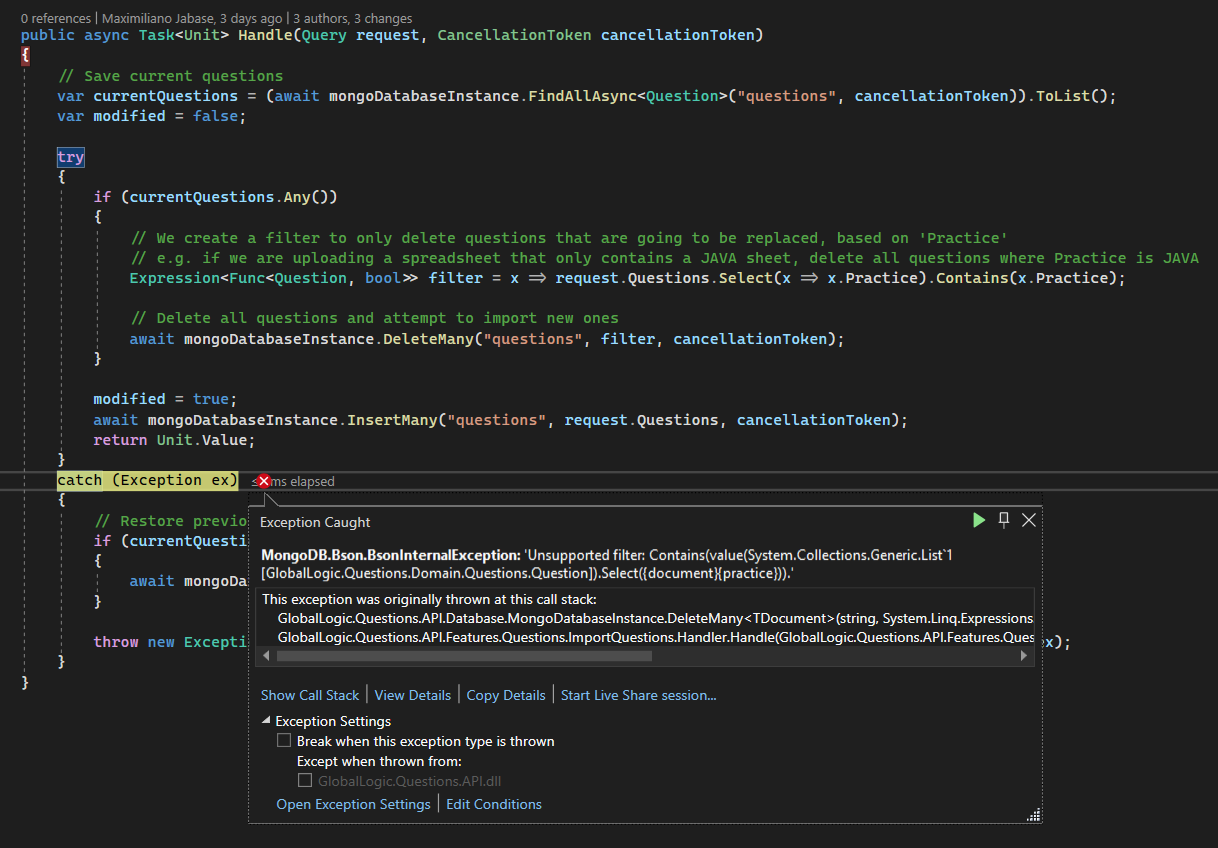
1 Reply
Solved, I had to modify the
DeleteMany
method implementation to receive a FilterDefinition<TDocument>
instead of an Expression<Func<TDocument, bool>>
, so I could formulate and pass the following filter: