Referencing a foreign key in a DBSet [Answered]
I'm trying to create a small coffee POS system, see above for my main DBSet
Product Catalogue
that keeps a database of all of the products available for the coffee shop.
Users (the employees in this case) have the option to add a product of their choice into the order menu. However, I don't let the user type out a string of the exact name matching the product's name because that would unrealistically assume that the employee knows the product off by heart. Instead, I want the console to prompt the user for an item, something like this
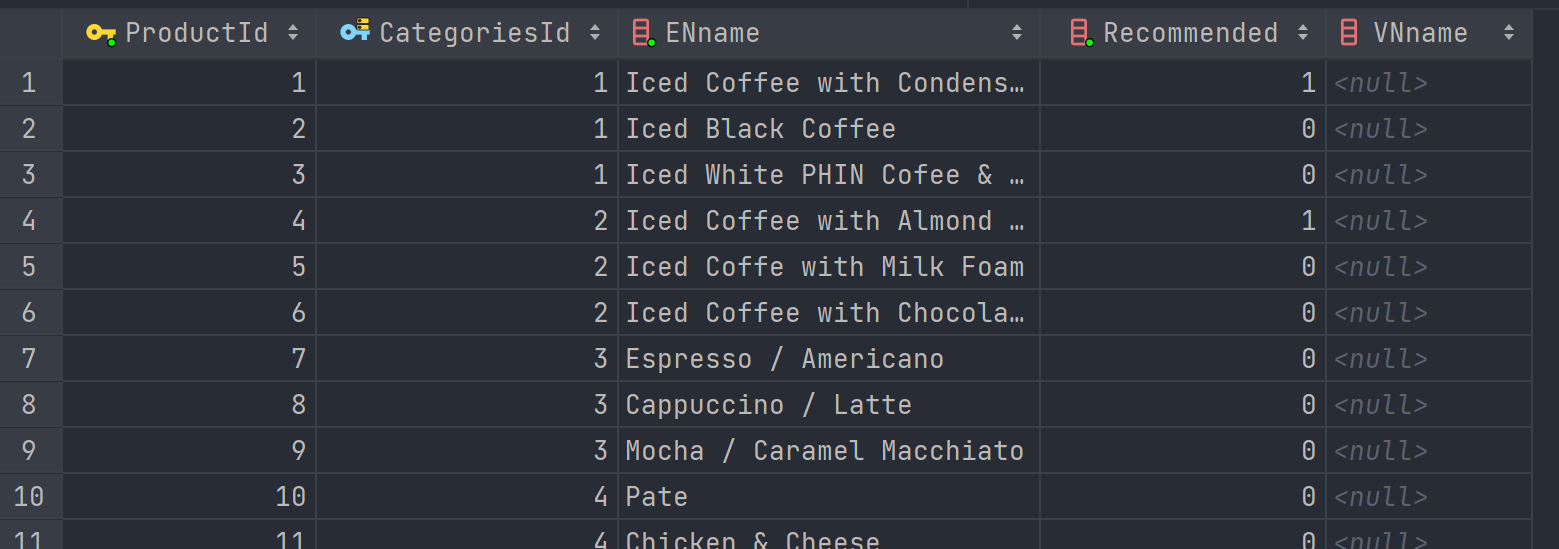
66 Replies
Below is the code to prompt user for a similar sequence of multiple-choice inputs
What I don't understand is this line right here throws an error

Unknown Userā¢3y ago
Message Not Public
Sign In & Join Server To View
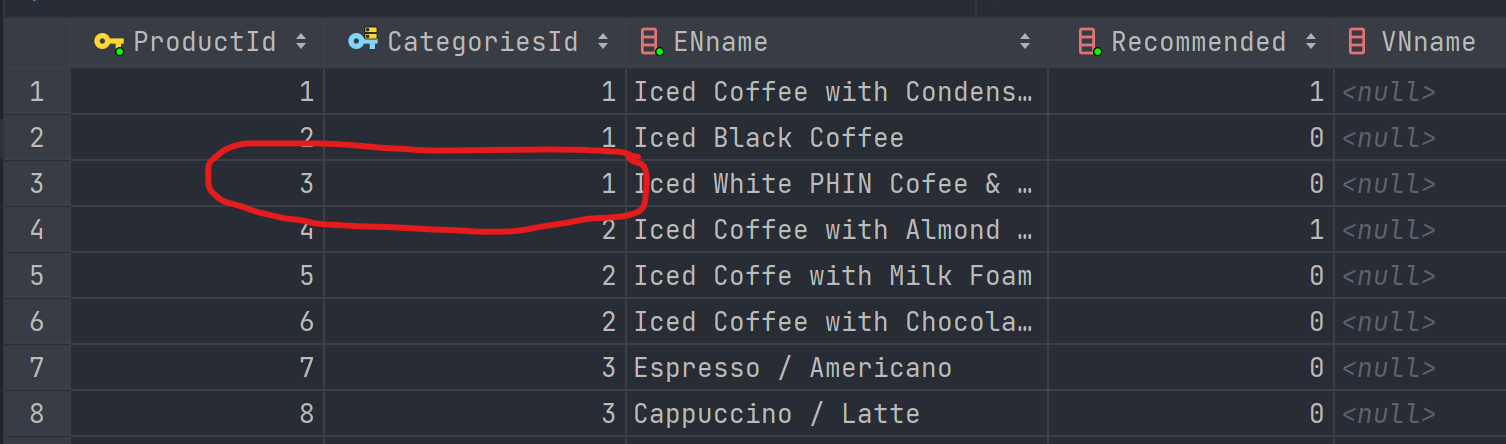
I'm trying to reference a foreign key through the categories table something like above
Unknown Userā¢3y ago
Message Not Public
Sign In & Join Server To View
How do I compare the categories ID? That's the thing, the DBSet I show above doesn't have a property literally named "CategoriesID" it's a foreign key made by EF Core
What's the code to compare 2 ID's?
Unknown Userā¢3y ago
Message Not Public
Sign In & Join Server To View
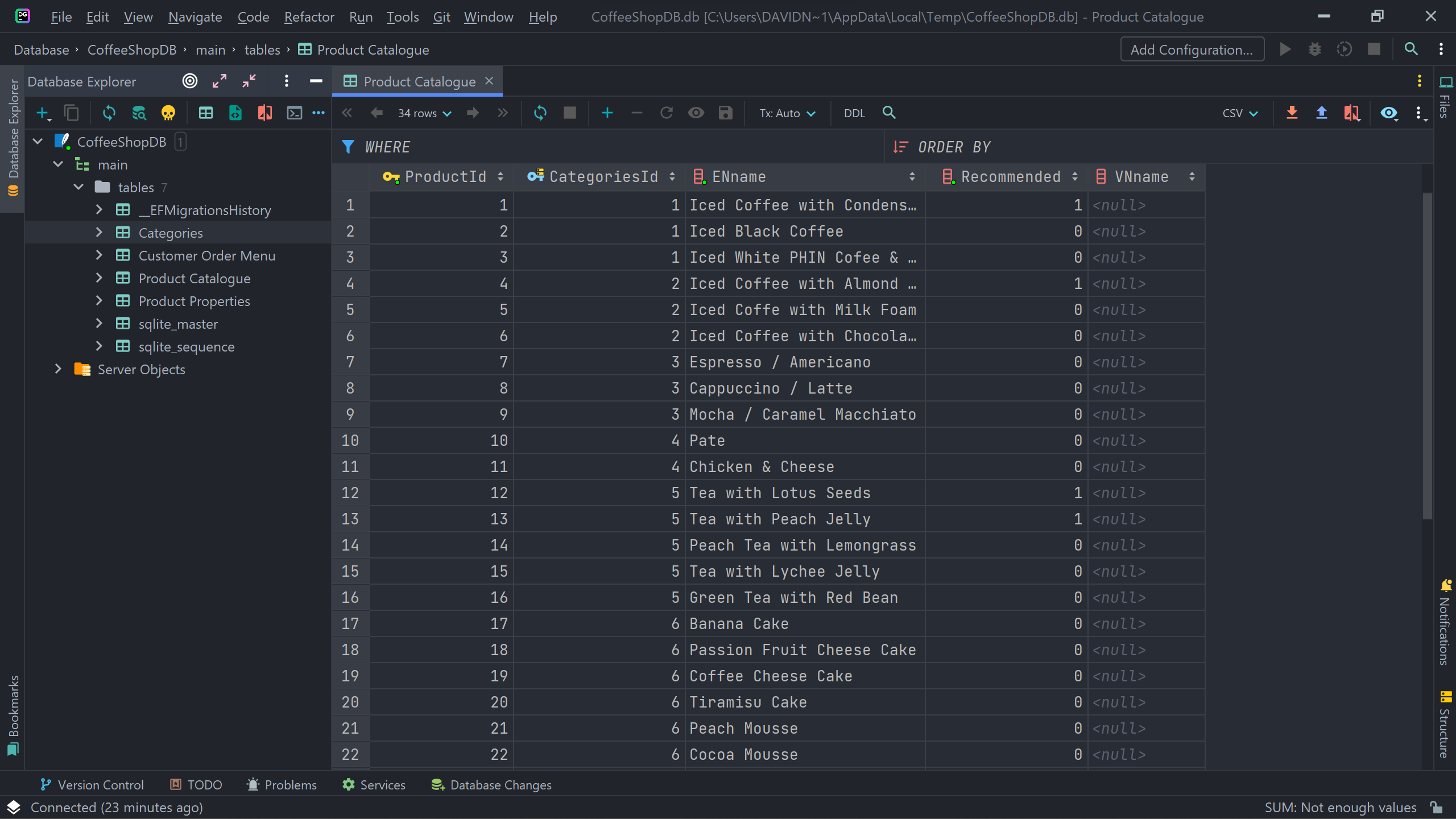
Unknown Userā¢3y ago
Message Not Public
Sign In & Join Server To View
Do I include that in 2 seperate DBSets or into one DBSet that is my
Product Catalogue
Unknown Userā¢3y ago
Message Not Public
Sign In & Join Server To View
ah, what does the
.Include()
method do?Unknown Userā¢3y ago
Message Not Public
Sign In & Join Server To View
yep, and I also have the
ProductId
Do I have to include
In my product catalogue table?Unknown Userā¢3y ago
Message Not Public
Sign In & Join Server To View
where do I write that code?
Unknown Userā¢3y ago
Message Not Public
Sign In & Join Server To View
o-0
Unknown Userā¢3y ago
Message Not Public
Sign In & Join Server To View
That's really weird, even though CategoryID belongs to the Categories class
Unknown Userā¢3y ago
Message Not Public
Sign In & Join Server To View
hold on, if I include the "CategoryId" does "CategoryId" refer to the foreign key specificallt?
Unknown Userā¢3y ago
Message Not Public
Sign In & Join Server To View
*specifically
Unknown Userā¢3y ago
Message Not Public
Sign In & Join Server To View
oooooh, interesting that the Post class has a BlogId and a Blog property
Unknown Userā¢3y ago
Message Not Public
Sign In & Join Server To View
Is that what's required to access the foreign key directly?
š®
Unknown Userā¢3y ago
Message Not Public
Sign In & Join Server To View
Ah, btw just to recap
Unknown Userā¢3y ago
Message Not Public
Sign In & Join Server To View
Do I write it like that or
Unknown Userā¢3y ago
Message Not Public
Sign In & Join Server To View
Like that?
Unknown Userā¢3y ago
Message Not Public
Sign In & Join Server To View
Like does the int have to match with the primary key name of Categories table?
ooooooo
So it's navigation property name followed by ID
Unknown Userā¢3y ago
Message Not Public
Sign In & Join Server To View
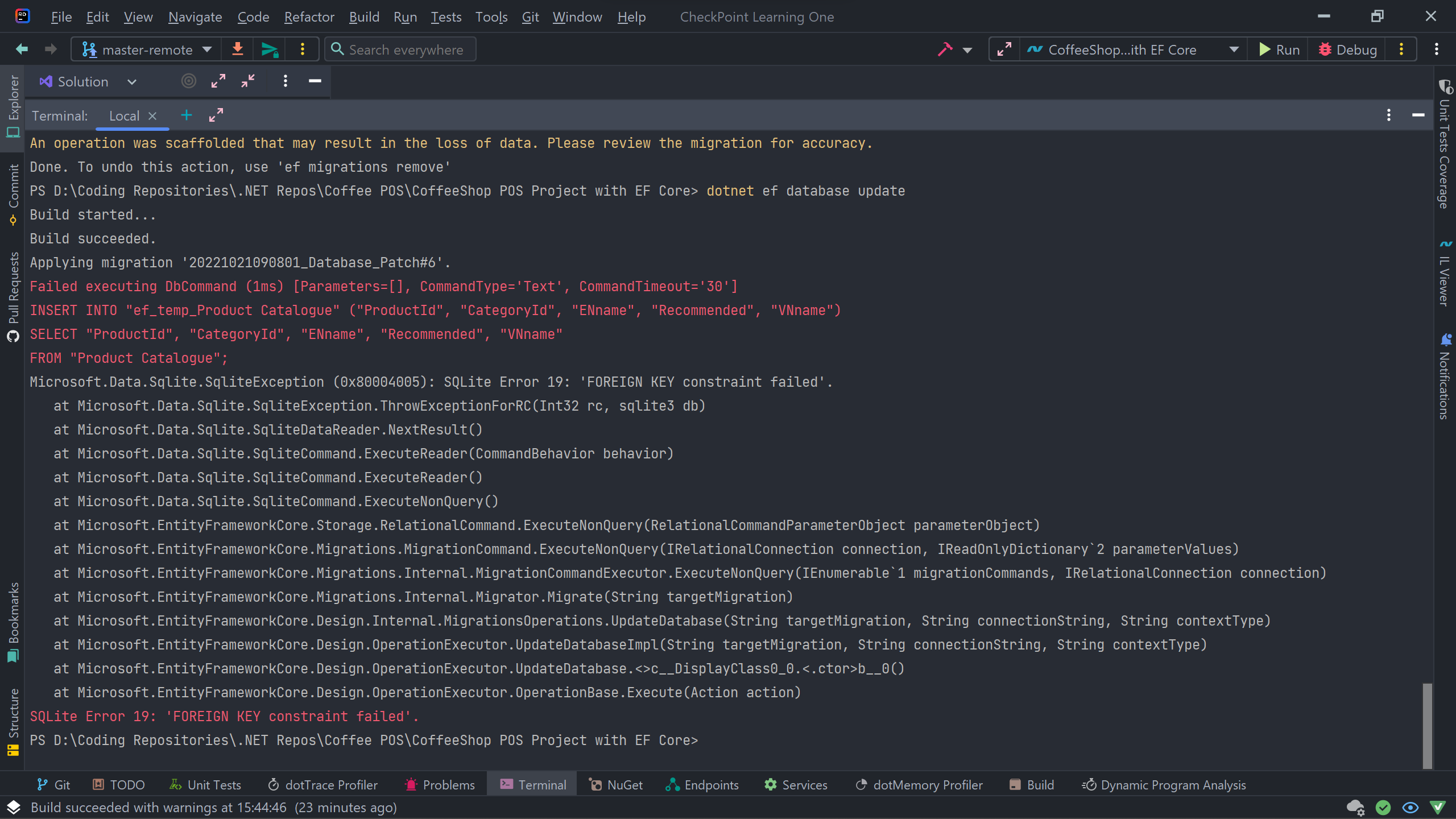
Alright got it here's my new code
Actually I'll just link to you my github repo
That way you can clone it and see the entire source code
Unknown Userā¢3y ago
Message Not Public
Sign In & Join Server To View
GitHub
GitHub - blueboy90780/Coffee-POS-System: A small practice project t...
A small practice project to consolidate all learning materials in "C# 10 Fundamentals" PluralSight course so far - GitHub - blueboy90780/Coffee-POS-System: A small practice projec...
Oh why is that?
btw that's my code
Unknown Userā¢3y ago
Message Not Public
Sign In & Join Server To View
Hi, sorry for going away for a while, is it okay if we call?
@Duke
Unknown Userā¢3y ago
Message Not Public
Sign In & Join Server To View
I'm stuck with this error and I've been agonizing over how to fix this foreign key issue
Unknown Userā¢3y ago
Message Not Public
Sign In & Join Server To View
It's ehre
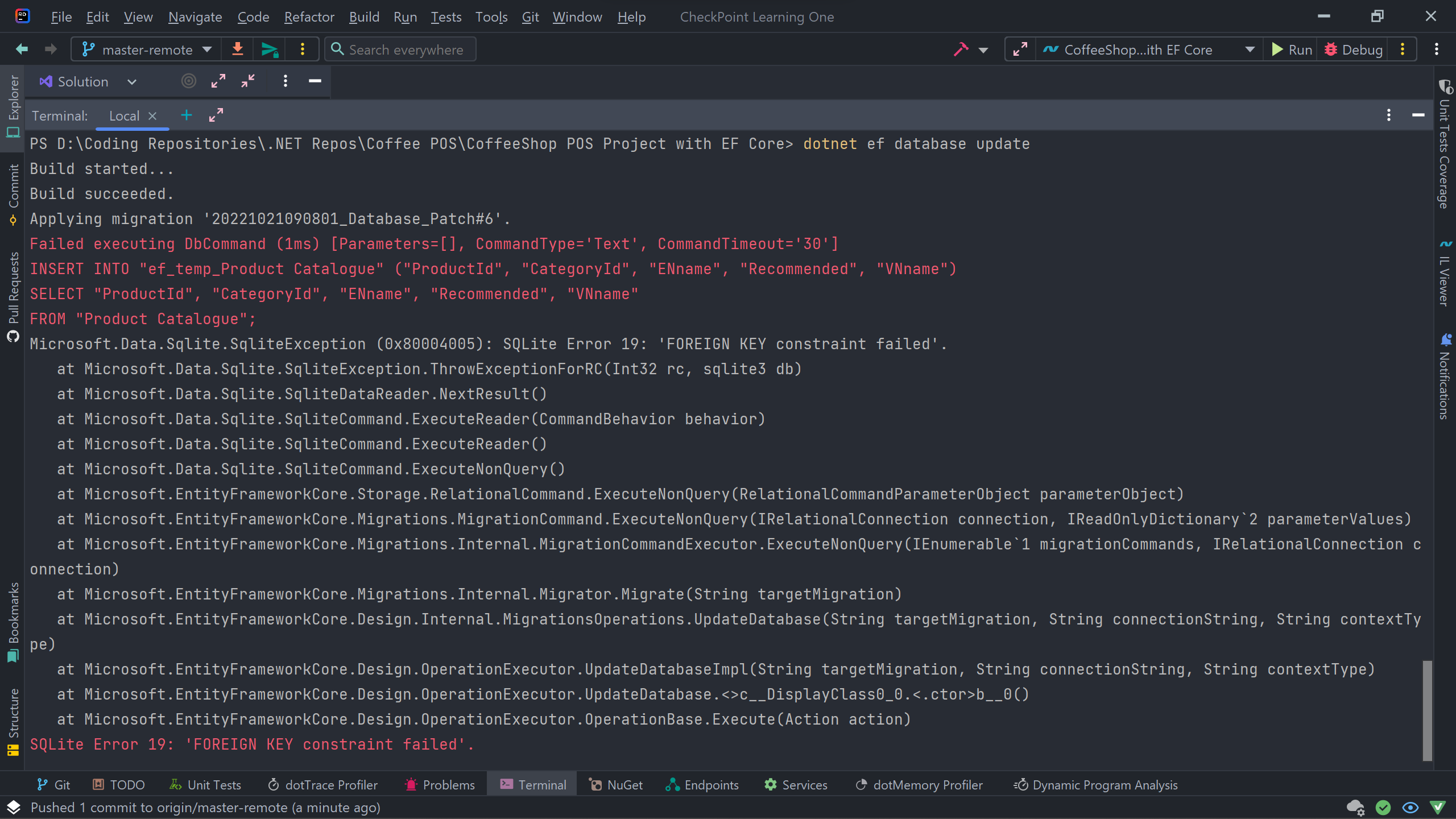
I just made a new remote push btw
The repo is completely updated with my local one
I'm not sure what I'm doing wrong
Unknown Userā¢3y ago
Message Not Public
Sign In & Join Server To View
like all the data in my table?
Unknown Userā¢3y ago
Message Not Public
Sign In & Join Server To View
Delete the entire DB?
Unknown Userā¢3y ago
Message Not Public
Sign In & Join Server To View
ALright I'll do that and then copy the data over to the new databse
*database
Is there a shortcut to delete all of the migrations in one command line?
Unknown Userā¢3y ago
Message Not Public
Sign In & Join Server To View
ooooo
DElete the folder and db right?
THE BUILD SUCCEEDED
WOOOOHOOOOOO
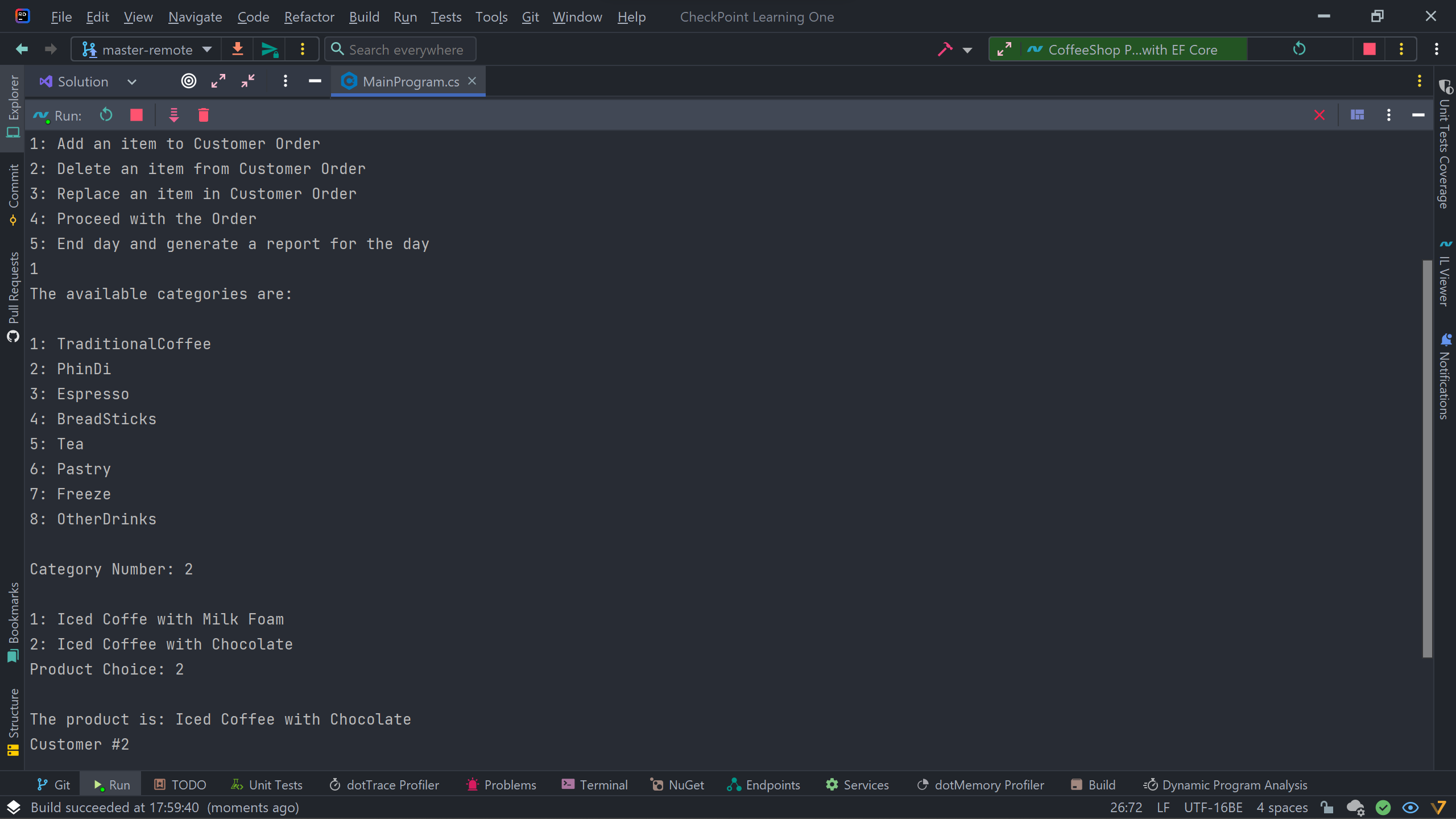
I DID it!!!!!!!!!!!!!!!!!!!
I DID IT!!!!!!!
FUCKING FINALLY
JAWOIDJAIODJOIAWJDIOWJOIDJWIODJ
AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAA
MY PROGRAM FINALLY WORKS AS INTENDED!!!!!
Unknown Userā¢3y ago
Message Not Public
Sign In & Join Server To View
Spectre.Console is a .NET library that allows for easy creation of console UIs, text formatting in the console, and command-line argument parsing.
https://spectreconsole.net/
Spectre.Console - Welcome!
Spectre.Console is a .NET Standard 2.0 library that makes it easier to create beautiful console applications.
I have, but I'm going to get to that once I get the core business logic down
ā
This post has been marked as answered!