getStaticProps runs multiple times!
Have no idea why this happens, few threads on github but no solution it seems.
When it runs, only one of the runs has the proper params, the rest are just undefined.
While we're at it, I don't totally understand the dataflow here (I followed the SSG Query pattern suggested on the tanstack page) so any explanation would be super appreciated.
12 Replies
I'm thinking the getStaticPaths configuration might be doing it
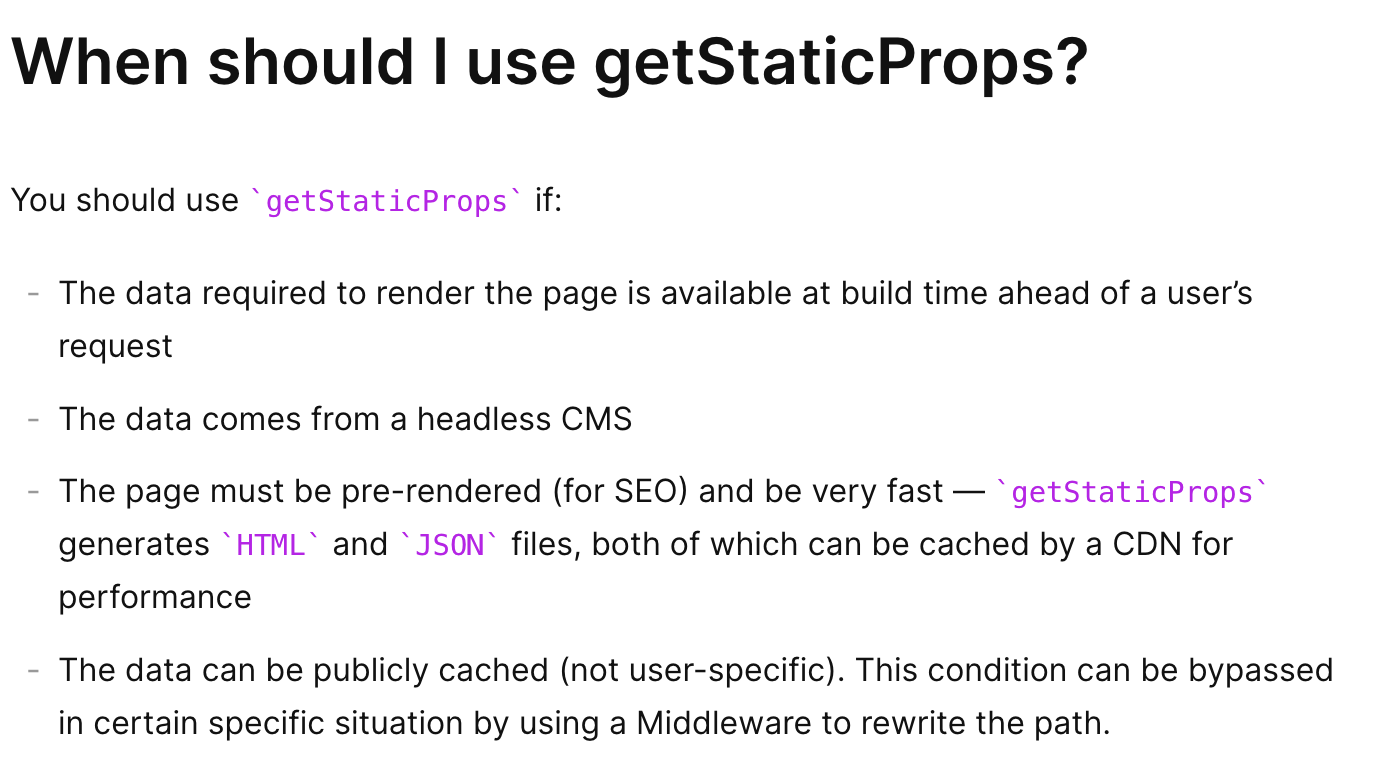
i feel like you are doing a foot-gun
Not gonna lie id just fetch it on the client as its quite dynamic page.
I switched to just fetching client side
I think this is a react query issue/some weird behaviour
One is getting post one is getting post.data
First request resolves perfectly, then it keeps querying
I think you just want data: {post} for them to be equal
Deconstruct inside the page component
Also what is the point in getting server side and the browser side, you just need one tbh
You just need to set up the cache invalidation rules properly, don't use tanstack query to do a second query. The get static paths needs access to 'all posts' so it can loop through them and build all the paths.
ok hold on i scaled it way the heck down because my brain = 🥟 right now
i basically ditched the api route i had and implemented it in gSSP
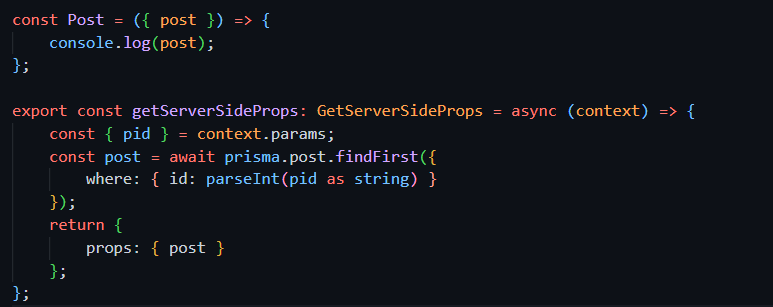
i guess consuming an api i made inside of getserversideprops (or even ssg?) apparently repeats the request tons of times
is there a better way to have done this
dont think im at the point where I can understand this yet :(
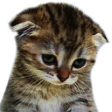
Checkout the ISR example on next JS docs, I'm assuming you're doing all this because you want all the benefits of a static site but built from CRM or a relatively fixed bit of backend data that won't change live very much
Yeah i'll definitely look into this to optimize after i get the mvp out
For now, is there a reason InferGetServerSidePropsType doesn't pick up the type of my prop?
props is just { [key: string]: any; }