Static vs Non-Static classes [Answered]
Please see here for the detailed question and all of the relevant code: https://gist.github.com/blueboy90780/f67813131e7a568d7998fcfea1166bd3
Gist
Used for comparing Static and Non-Static access to static fields
Used for comparing Static and Non-Static access to static fields - NonStaticClass.cs
23 Replies
I've asked my question underneath as a comment, it's hard to articulate this question without code context so a gist is used instead
A static member is not tied to any instance of the class so when accessed internally by an instance, the same static values are shared. You can also access it from outside the class without using an instance
A static class forces every member to be static and as a result, you also can't create instances of that class
Making a method static can be useful to denote that it doesn't reference instance members.
A good use of static method is for creating objects where a constructor doesn't make sense such as
Image.FromFile()
or Color.FromArgb()
Wait seriously? So even if I don't explicitly give the
static
keyword to all my members, they default to static
?
Ah, thank you that's pretty goodYou need to explicitely define everything as static in a static class
It won't compile if any member is not static
Ooooo
Thank you this clarified a lot
Btw when should you use a static method over a non-static method? If a non-static method is able do everything that a static method is able to do and more, then why should we ever use static method?
Is there a functional reason beyond just a syntactical indicator?
Static Constructors are a little different than usual static members, its closer to the
readonly
keyword
Notably, they're called before the first instance (if any) or right before something references it.
What they mean by "references" is using something from that class anywhere in your code. Example: calling a method, creating a new instance, etc
A static constructor can be created in a static or non-static class.
More info here: https://learn.microsoft.com/en-us/dotnet/csharp/programming-guide/classes-and-structs/static-constructors
The performance between the two are negligible (around 9% faster when i tested in favor of statics)
Static vs non-static is based on "Do I need more of this?" or "Does my class inherit anything special"
If you have some file manager that only exists once, then static is the likely bet
If you have something such as a File
A File can have a few methods like
- Delete
- Move
- Current Directory
- Parent Directory
- Get Files (Returns a Collection of Files)
Regarding "Does my class inherit anything special", Unity implements this using Monobehaviours
If a non-static method doesn't reference anything in your instance, you might want to consider making it static.
An example of this would be
Some class instances are automatically created behind the scenes, for example
9% faster is still % faster, so I think I'll be using static whenever it makes sense
What can static methods do that non-static methods can't do?
Like what would be the purpose?
its definitely not 9% faster
where did you get that number
my general rule of thumb is if a method doesnt need any instance members, make it static
You can use a static method without creating an instance of the relevant class.
There are cases where this is useful, e.g. factory methods:
more generally you can think of using/not using static methods as engaging with OOP or not.
sometimes it's useful to model your problem or program in terms of OOP, in which case you don't need static much - other times OOP just gets in your way and you want to 'turn it off'
Generally I veer away from using static because I find it to be a code smell. It encourages massive methods where you pass around fifty parameters, rather than just having a class with some state
Extension methods are only allowed to be created in a static class, and are always static methods - so thats a pretty common usecase.
extension everything when 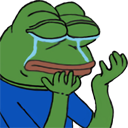
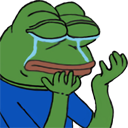
benchmark dotnet
extension events and properties getting no love, gotta make them by hand <a:AA_Satania_Cry:599145877071724555>
and you got 9%? wow
9% is still 9% which is pretty good
these are my results:
BlazeBin - bizzhutqjbwf
A tool for sharing your source code with the world!
on a 5600x
so on other hardware it might be 9% slower but that would be microseconds in difference, which is practically nothing
did a similar test
on a 3600
https://gist.github.com/5b472e01fb7228f2fe2d8814c5f62833
I was unable to replicate yours since
NonStaticTestClass
wasn't included in the paste nor referenced within the benchmark
the ? | ? is the change from frameworks (2 separate projects using the same code)I was unable to replicate yours since NonStaticTestClass wasn't included in the paste nor referenced within the benchmarkoops i was testing something forgot to remove that the benchmark doesnt use that
gotcha, i'll try pasting the test without that class real quick then
oh you were testing fields
but yeah either way the difference in performance is negligible
Ran your test just now and its similar to your results
though yeah, sadly there's no conclusive test (without running a million different scenarios) to test static vs instance
but yeah, still negligible
✅ This post has been marked as answered!