Can't access local variable inside an if...else statement [Answered]
When trying to print productChoice which should be a dictionary object. Rider is flagging it as an error, does anyone know why?
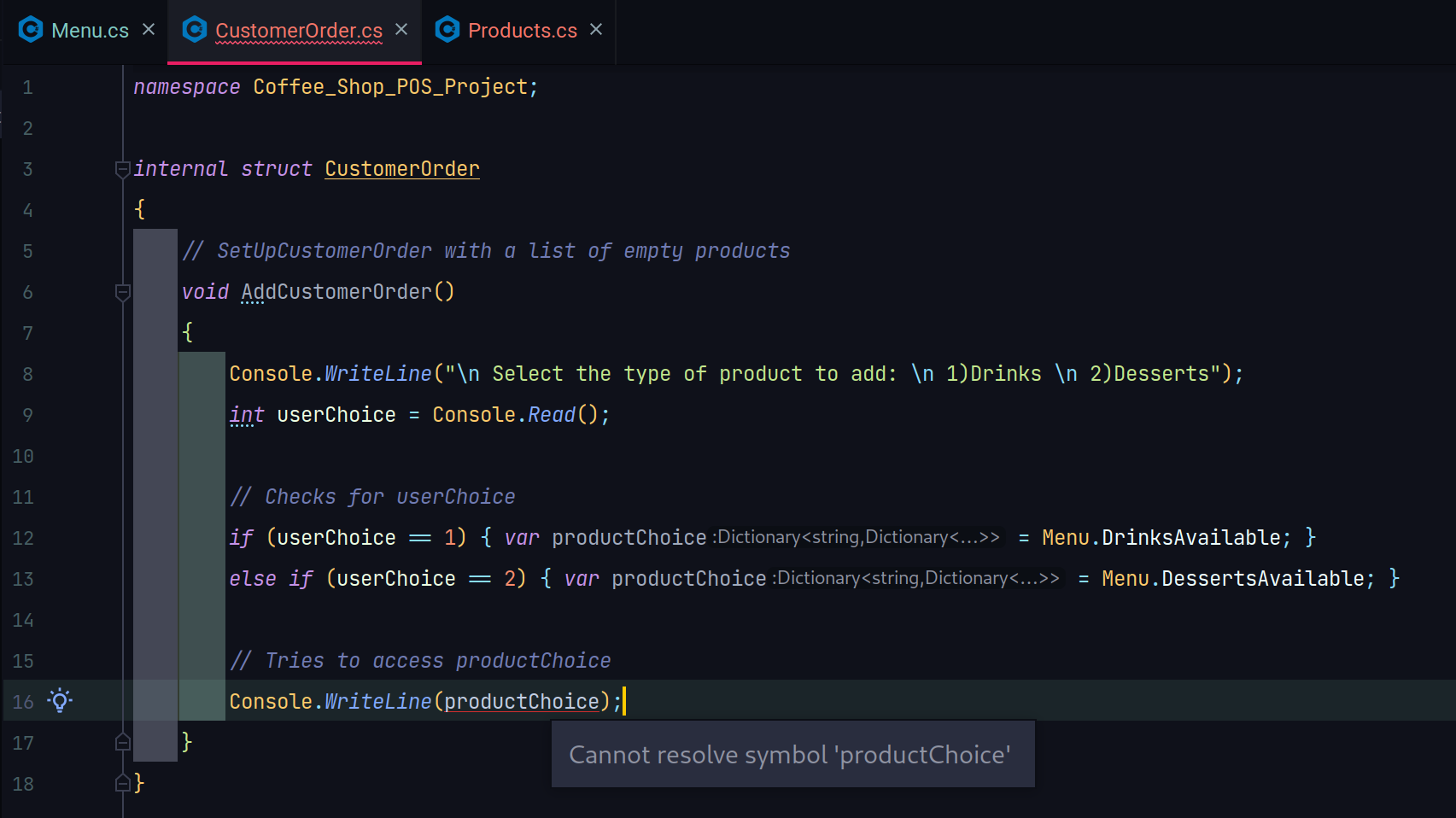
70 Replies
$scope
you probably want
$scopes
$scopes
thing a
is available in scope A
and scope B
thing b
is available only in scope B
@Cyberrex I thought this only applies to methods
Methods and classes
How can I make it such that thing b is also available to Scope A?
you define it in scope a
Yeah but I have to initialize it in scope B
Also if Scope B is just a simple if statement, does that count?
In Python, you'd be able to use variables defined in an if block outside of the if statement
Unknown User•3y ago
Message Not Public
Sign In & Join Server To View
Right but I have a problem because I can't declare an uninitialised var 😓
I tried declaring
var productChouce
without any assignment in Scope A, but that didn't workUnknown User•3y ago
Message Not Public
Sign In & Join Server To View
btw
Console.Read
doesnt do what you think it does
it returns the unicode value of the charUnknown User•3y ago
Message Not Public
Sign In & Join Server To View
Just to clarify. Menu.DrinksAvailable and Menu.DessertsAvailable returns 2 different dictionaries format
What should I be using instead?
ReadLine
or ReadKey
What should I do if both of those methods return 2 different format?
thats horrible
Menu.DrinksAvailable returns
Dictionary<string, Dictionary<string, Tuple<int, int, int>>>
wtf
Menu.DessertAvailable returns
Dictionary<string, Dictionary<string, int>>
it's 2 different dictionaries 😦c# isnt dynamic
thankfully
What should I be doing instead?
To parse that?
you should rethink the design
first of all you probably dont need a dictionary of dictionaries of tuples
What if I were to make Menu.DessertAvailable return
Dictionary<string, Dictionary<string, Tuple<int, int, int>>>
as well? But the last 2 int of the tuples will just be null valuable
Not really sure how else I can accomplish thatbtw
Tuple<>
is old syntax, now you just do (int, int, int)
oooooo
and you can name them
(int foo, int bar, int bla
)
but creating a class/record is usually a better idea
what are you makingUnknown User•3y ago
Message Not Public
Sign In & Join Server To View
I'm making a data structure that can represent the menu above
I'm only covering part of the menu
ok so lets start with the base
Like here's my code so far
do you have a base class?
No I don't, how does inheritance work in this?
I'm making a POS Console Application for this coffee shop
are you familiar with inheritance in other langs?
It's not a client, just a practice project
Yeah, in python and Java
its a good practice project
Thank you 😄
yeah to inheritance in c# is very similar to inheritance in java
sorry i gtg
be back in a bit
im back
so yeah its similar to java
I suggest starting with the most generic class you can, what do all your products have in common? A name and description, a type (dessert, etc), and three prices. So make a class that has all those things. Then you need an Order probably, because that would contain a Product, Quantity, and probably Server and Table (those should be classes too)
Though you'll probably actually not include a Type; a Drink and a Dessert likely becomes its own class, extending Product
a couple of small differences like in c# you have to explicitly define methods as virtual, and
override
is a keywordUnknown User•3y ago
Message Not Public
Sign In & Join Server To View
Can I ommit the "new" keyword safely
see this is why you need classes
>_<
so lets scrap all that
and make an abstract base class for menu items
But I don't understand how inheritance can play a role here, since isn't using a class a big overkill in this case?
no youll see why this is a perfect use case for classes and inheritance
not using classes makes this code messy and hard to maintain
the base class would only have 3 properties, the name of the product, the size of the product (for drinks) and the price. That's it
ooooo
^
nearly impossible to work with if you don't use inheritance tbh, because it's not dynamic. Instead of a bunch of different dictionaries, you'll end up with a List of Products, where a Dessert is a Product, and so is a Drink
and youll have strict typing instead of relying on magic strings and indexing
O
I've seen pluralsight videos on OOP that goes into this into quite a lot of detail
In the context of C#
Though an 'Order' is a misleading name for what I was describing... nor would it have a table... tbh restaurant structures can get complex, lol. But I'd probably make...
MenuItem - something on the menu, has at least one price/size pair (that could indeed be a dictionary, but should be an enum of sizes, and a Dictionary<Sizes,double> to get the price (one of the enum values might be Sizes.Default, for items that don't have a size)), name, description
Dessert/Drink - These would extend and be a MenuItem (but unless you have some special needs for things depending on their type, such as refills, there's no real reason to have these. But you seem to want to display info per-category, so then these are still useful)
ItemOrder - Contains a MenuItem, Quantity, and Size
Customer - Contains a collection of ItemOrders and a seat#, and probably a reference to the Table
Table - Contains a collection of Customers, probably a table#, and a Server
Server - Contains any info you have about your employees, probably just a name for these purposes
But anyway, the important part is just making them first - because in the process of making them, you accidentally design your app. Once they're made, the app becomes straightforward and uses natural language
But there's legit only a few fields which they have in common, a name and a price, and price isn't even all that common either
Price is often 0 in POS systems for free things. And it's not just a name and price, it's a name and possibly multiple prices, and a description
And if you wanted to have an order filled with lots of items, which would also need quantities and other info, you'd have this horrible mess that would be way too hard to work with
even if its just 2 properties, its something in common that defines them
so lets create the
MenuItem
base classDoing it with classes means you can just do something like
table.Customers[0].Orders.Add(...)
and it just makes life way simpler. But my suggested structure isn't necessarily the right way to do it, it depends on your requirements and whatnot. But you absolutely should use classes or recordswhat properties does every menu item have?
They have categories of food and drinks being offered
thats the menu itself
look at this image
every item has a:
category (hot drink, cold drink, desert...)
name
description
sizes
price for each size
There's no description
It's just the English translation of the Vietnamese name above
oh
well you can omit that
so a
MenuItem
contains these properties:
category
name
sizes
price for each sizeAnd I feel prices wouldn't be enough because there's 3 categories of prices
Yeah prices for each size
But what about the food?
The pastries don't have any size
we can have a default size
Size.Regular
for things that dont have sizesWhere size is an enum?
@CyberrexI've been told that using records would be a better alternative to using dictionaries in C# 10
https://app.pluralsight.com/paths/skills/c-10
I'm still figuring my way around, could you point me to the course that talks about records in C# 10?
@D.Mentia @Cyberrex
records are (by default) readonly classes that have value semantics
classes are part of the fundamentals so you need to learn how they work first, in order to really understand records
idk any courses sorry
Not really about courses, but rather which of those courses inside that path talks about records
✅ This post has been marked as answered!
✅ This post has been marked as answered!