Call trpc query after click or change
I am trying to fetch some data on a change event
But I think the query method is not meant to be called like that right?
59 Replies
yeah it is a hook I can't call it like that
hmm ok I see
but still get the error that value is not found here
can't I pass the id as a param?
my bad i just copy pasted your broken code
const result = trpc.client.getOne.useQuery({ id: value }, { enabled: false })
function handleEvent() {
result.refetch();
}
something like this idk check the docs
the point is you want to use enabled and refetch
yeah I get that point, but I can't find a proper example to run queries on a click or something similiar here
https://trpc.io/docs/v10/react-queries
useQuery() | tRPC
The hooks provided by @trpc/react are a thin wrapper around @tanstack/react-query. For in-depth information about options and usage patterns, refer to their docs on queries.
Tried this workaround but does not seem to work aswell
If you want on click behavior you can click at using mutations instead? but either works honestly. The issue with your code seemed to be that value didn't exist
I didnt realize you wanted it not run until you call it but anyway, in your onclick all you have to do is refetch as long as your dependencies are valid. I would honestly make this a mutation since it supports passing in params which I never do with queries/ Idk if its even possible
I can try that, I always thought to use mutations for like creating,updating and deleting stuff
and useQueries for fetching data
Yeah i understand and use that distinction. You can probably make this work but let me look at these docs really quickly
thanks, I have going to make some coffee so no hurry š
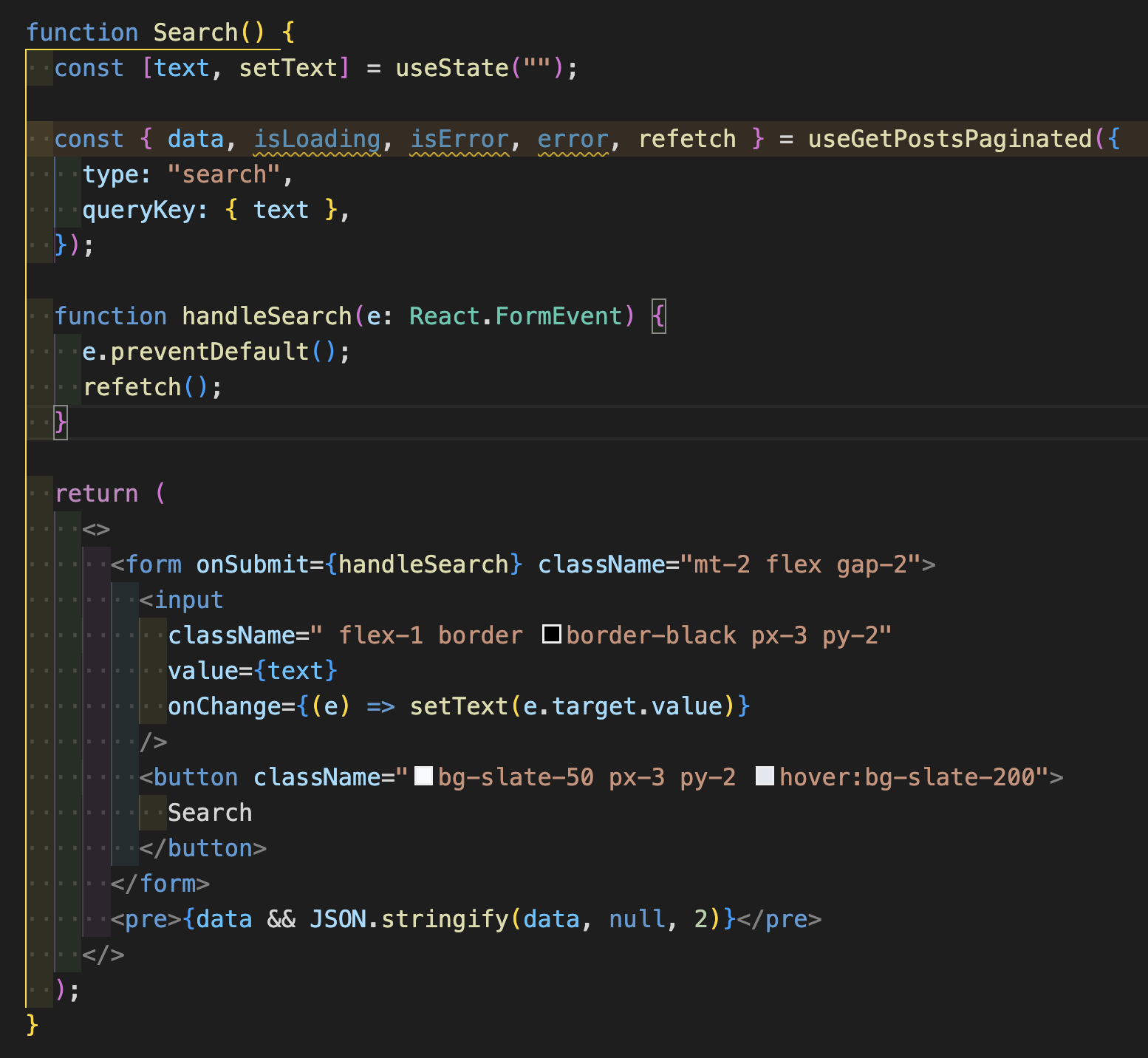
This is definitely the more standard way to do things but uh looking at the docs im trying to see if you can pass in props afterwards
š¤
i think u guys dont understand how react components work
you can just put state in the query param
it makes a new one everytime the component rerenders
Yeah I understand but hes trying to force it thru params
Ive been using react query for a decadeeeeeeeeeeeeee and havent ever reached for what theyre trying to do.
you pass the params when you make the query
Usually you'd use a value from your components context which is from where ever. OP wants the ability to pass in any value or at some point changed his mind on using the standard way
Basically on whatever change event I want to trigger the query with my specific id
but the component rerenders everytime any of those things change
yes yes yes
As long as the value exists you can jsut pass it in as cje did
yea thats what the screenshot i just posted does
on form submit it triggers the query with the text state
oh I see you are using a seperate state for that
Yeah but it doesnt have to be that either
it works with props as well
because guess what happens when props change
IT'LL REFETCH

no not necessarily lol
refetch happens when you trigger the refetch function
Oh i was referring to in general passing props into your query
If you have props attached to your query, changes to them will trigger a refetch of the query itself or atleast a NEW QUERY
Soo you say this should work?
it makes a new query but it doesn't fetch it
Im referring to general behavior, enabled: false ignored in my statement.
Yeah I initially dont want to fetch it
Sorry side tracking your problem
so many things wrong with this
function doesnt need to be async
you dont need to pass anything to the refetch
you dont need to return anything
Why don't I need to pass anything to refetch? š
Your query is dependent on this
yeah I just put that as a "placeholder"
Cje gave you a valid solution where you'd stick to manual refetches but you need to have somekind of state set up to update this value so your query can fetch
Using that string is not going to work since you will always be fetching with that data
ohh
I dont know if refetch supports what you are trying to do and looking at the docs I thinks that true
okok
give me a sec
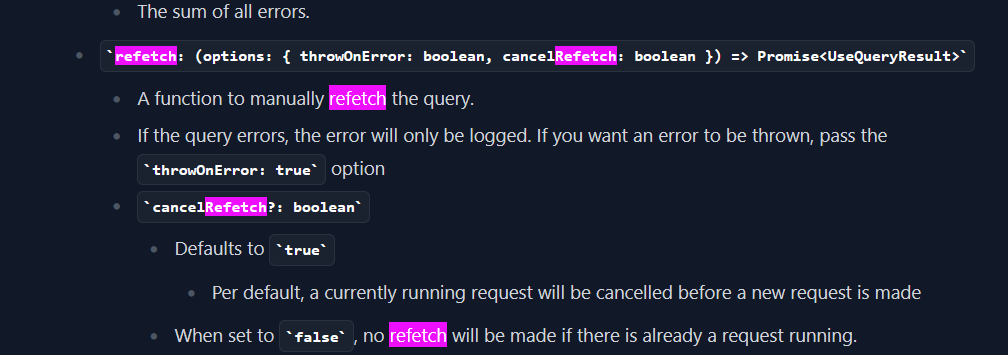
This
Why are you passing in params into the refetch
You have a value just call refetch and it'll do thing
sorry mispasted
I think it works now
š yay
Some other stuff you can do with RQ is you can set your client ID to a hard state like NULL and use that as your existence clause. Your onclick should just update that state. That update to state should trigger a new query which will invalidate the old
more important, I understood it
Thanks guys
Take a look at my last message before u leave forever š„ŗ
RQ is really good with how it handles stuff so you should just use the builtin functionality before reaching to do stuff like that imo
@sockenguy
But I am doing that right now I think
No u arent
I set it in my case to an empty string, with enabled: false it does not query immidiatly, on refetch it queries with my new state set
sure i guess. Your await is unneeded im assuming thats just pseudo code and not actuallywhat you are doing
I was just saying try adjusting how your data flows to simplify things
yeah I am using it to see the logs
the enabled property is really really good if you take advantage of placeholder state such as enabled: !!user.id which means it wont fetch until the user.id results in a truth
true, probably going to need it more for upcoming features š
It wont fetch immediately unless user.id already exists so we can basically do what you want with just using enabled and not having to export the refetch
but upto you if you want to try it, what you have does work
Thanks @CFKeef , appreciate the help!
np np if you do end up trying it and i'm wrong feel free to ping me to correct me, I think it should work? but i'm not testing it rn and it usually works when I work with it in the way I described šø
I will, thanks š
This is how I was describing it gl š
Make sure to make use of the other stuff useQuery exports like isLoading to handle the different states your component is in and you are on ur way to being a menace with RQ