HTTP Post query
I am currently learning the post query using HTTP from the https://ptsv2.com page.
My question is whether what I get in my textbox makes sense as far as it goes, or whether I've just written garbage after all.
so, this was the code
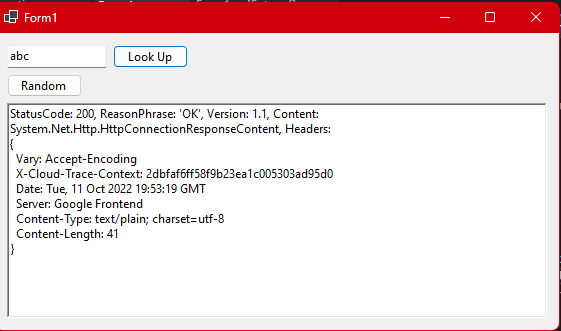
16 Replies
and this is the HeadersMain:
you're just dumping the response, you're not going to get much interesting from that. You need to read the body to see what the response data was
await request.Content.ReadAsStringAsync()
oh, and more not?
what?
So, when I compare the input from the program with the input from the page, the same thing comes out. (I'm really new to this, that's why I get such stupid statements).
rn you are just outputting the header data of the response (
result.ToString()
)
which looks good because it says "StatusCode: 200, ReasonPhrase: 'OK'"
but it also says "Content-Type: text/plain" and "Content-Length: 41", which means the response also has a 41 bytes long-plain text content
you can get the string out of the content by calling await request.Content.ReadAsStringAsync()
(as Cisien pointed out) and output it too, to see what it saysYeah, I already did that.
ok, but you shouldnt just call
.Result
on Task
s
properly await
them π
$asyncFor an introduction to
async
and await
in C#, https://blog.stephencleary.com/2012/02/async-and-await.htmlAsync and Await
Most people have already heard about the new βasyncβ and βawaitβ functionality coming in Visual Studio 11. This is Yet Another Introductory Post.
oh
Wait
no, await π
yes, await
so?
don't chain those
you're still calling Result on the Post
var result = await client.PostAsync(...);
var body = await result.Content.ReadAsStringAsync();
Thanks ! β€οΈ
probably wanna check
result.StatusCode
before you read the body, too π
or call result.EnsureSucessStatusCode()
, it will throw an exception, if the StatusCode does not indicate success πoh, thanks π