Check for Slash Command Permission
Hey, I want to check if a user has the permission to use a slash command while taking into account all the different overrides (channel, roles, user etc.).
(Im using this to match my prefix commands permissions with the slash commands)
60 Replies
• What's your exact discord.js
TextChannel#permissionsFor()
Gets the overall set of permissions for a member or role in this channel, taking into account channel
GuildMember#permissionsIn()
Returns
npm list discord.js
and node node -v
version?
• Post the full error stack trace, not just the top part!
• Show your code!
• Explain what exactly your issue is.
• Not a discord.js issue? Check out 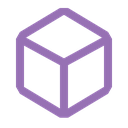
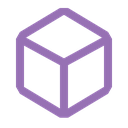
channel.permissionsFor(guildMember)
. Returns permissions for a member in a guild channel, taking into account roles and permission overwrites.Unknown User•3y ago
Message Not Public
Sign In & Join Server To View
thats not for slash commands
only for channel permissions
Unknown User•3y ago
Message Not Public
Sign In & Join Server To View
Aren’t you able to set those up within the server settings?
Yes, im talking about the permission v2 that you can manage under the server settings > integrations
Unknown User•3y ago
Message Not Public
Sign In & Join Server To View
doesnt take channel overrides into account
Unknown User•3y ago
Message Not Public
Sign In & Join Server To View
theres also channel overrides for slash commands though
those arent managed in the channel settings
Unknown User•3y ago
Message Not Public
Sign In & Join Server To View
its just that
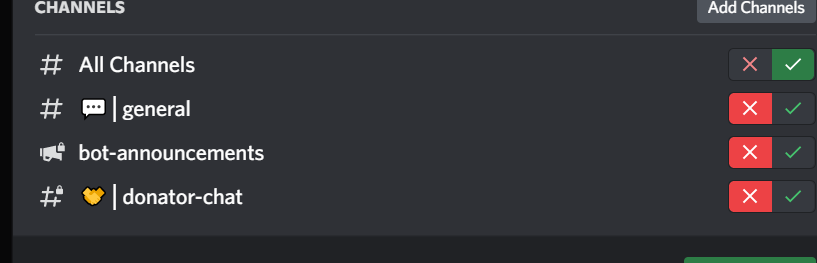
Unknown User•3y ago
Message Not Public
Sign In & Join Server To View
A friend made like a really complicated check for this and i was just wondering if you could do it with less code.
I can send you his code snippet if you want to.
Unknown User•3y ago
Message Not Public
Sign In & Join Server To View
there we go
you might have to load that into an editor because of formatting
Unknown User•3y ago
Message Not Public
Sign In & Join Server To View
Unknown User•3y ago
Message Not Public
Sign In & Join Server To View
oh
Unknown User•3y ago
Message Not Public
Sign In & Join Server To View
pog
they fixedit
Unknown User•3y ago
Message Not Public
Sign In & Join Server To View
ohh wait
another problem
the has method returns true if a permission exists. that permission could also allow the user to access the command
Unknown User•3y ago
Message Not Public
Sign In & Join Server To View
I dont think the channel permissions matter at all
only the slash command overrides
Unknown User•3y ago
Message Not Public
Sign In & Join Server To View
yeah thats what this does
Unknown User•3y ago
Message Not Public
Sign In & Join Server To View
right
thats what this does
Unknown User•3y ago
Message Not Public
Sign In & Join Server To View
how would you shorten it
Unknown User•3y ago
Message Not Public
Sign In & Join Server To View
You can take a look at the code, he did the checks in the order the types are applied
Im not sure about the roles
wdym overrides are missing?
Unknown User•3y ago
Message Not Public
Sign In & Join Server To View
I am pretty sure they completely override the defaults
thats the purpose of the permissions manager
I gtg for today, bye :)
Unknown User•3y ago
Message Not Public
Sign In & Join Server To View
Can I ask what it is you're building this check for?
Unknown User•3y ago
Message Not Public
Sign In & Join Server To View
Okay thank you :D
Ill go through it and compare it to the old function
Im pretty sure the order is like that (all roles = everyone)
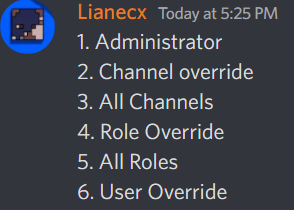
but needs testing
couldnt find any docs anywhere
Unknown User•3y ago
Message Not Public
Sign In & Join Server To View
@lianecx did you use this in anything? I think I need to use something like this too
Discord has made some changes to permissions including a flowchart that should make it easy to implement something like this
https://discord.com/channels/222078108977594368/992166350640386090/1042878335304347758
Yeah i saw the flowchart
still a lot of work 😵💫
I can send you my updated code once its done
Its not released to every server yet so i havent changed it
ooh please do that'd be greatly appreciated
👍
Feel free to ping me if I forget to post it
alr
@lianecx hey, did you end up making this?
I believe discord still has their new app permission system in beta.
I also dont know if spoon feeding my code is welcome here 😅
Am I missing something here? That referenced post was from last year, definitely not a beta anymore
It was opt-in for servers like a month ago
idk if it still is
I think its released
they were planning to release it in late february
I actually stopped support for my prefix commands so this wasnt needed anymore, with the flowchart that discord attached it should be pretty easy to implement this though as the discord.js objects and functions mostly resemble the discord api itself
Yeah I may have to have a stab at implementing the user/roles bit of it for my bot. Sorry for bothering you
No worries, lmk if you need more help
😄
If you still need this, a friend just implemented it for his bot, here it is:
I'll check this out at home, thanks for sharing
No problem!
obviously it's meant to do nothing while false
makes me worry for when I open it up to look at
I was hoping at the time it was in some sapphire library, it's not that I can't write it it's that it's annoying to write 🫠
