Threading Return to old thread
I have the following code where the function on line 13 changes the thread to another id. How can I make it so that when
Task.Run
ends, I am back on the original Thread where CallOnMainThread
started?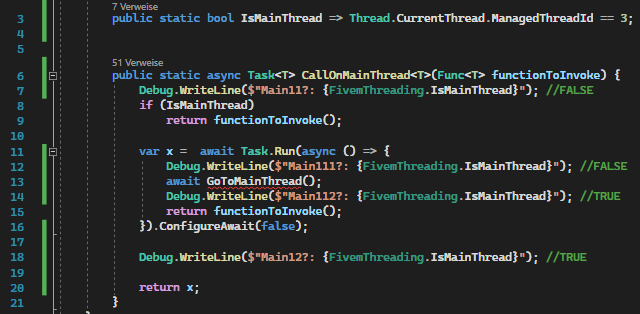
18 Replies
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
Basically the !server!-side code isn't multi-threading friendly and thus you often need to go to the main thread to execute some code.
This helper is supposed to do the following:
If already on main thread, execute the code
If not:
- go to main thread
- execute the function
- return to original (background) thread
(in order to not put too much load on the main thread)
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
The problem is that I need a result back. It's not an Action but a Func<T>
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
I have to rephrase myself. It does not have to return to the original background thread but it should not stay on the main thread because then all following code will be run on the main thread subsequently putting a lot of load on it.
My goal of this function / helper was to translate example code that would look like this:
Because in the first example when you need to go to the main thread more frequently you're going to nest more and more
Task.Run
sYou can check out https://github.com/Microsoft/vs-threading
GitHub
GitHub - microsoft/vs-threading: The Microsoft.VisualStudio.Threadi...
The Microsoft.VisualStudio.Threading is a xplat library that provides many threading and synchronization primitives used in Visual Studio and other applications. - GitHub - microsoft/vs-threading:...
ideally you want one thread which responsibility is to communicate with the game. That thread should look at a queue of operations and execute them and post the result back
you can create
Task<T>
s that resolve when the result is posted back, so the rest of your system is unaware that a specific thread does the work based on a queue
it'll be
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
I don't see why this pattern wouldn't work perfectly for their use case
It could work with that but there'd have to be some way of retrieving the evaluation after it's done.
you could use a
TaskCompletionSource
it basicaly works like this:
note that DoWork immediately returns tcs.Task
and that after a second, the Task.Delay
causes the tcs.SetResult("abc")
but DoWork
returns a Task<string>
. It's invisible when the task actually gets completed and howAhh. That sounds implementable
So I would insert a touple with Func<T> and a TaskCompletionSource<T>
In the worker I would evaluate Func and set the result for the source.
(?)
so you can do the following
Alright. I'll implement that and see where it gets me :)
good luck!
Alright. I think I've got it done.
However I also want to allow Actions (without parameters).
Is there something else I could use for
TaskCompletionSource
or just use TaskCompletionSource<bool>
and set to true?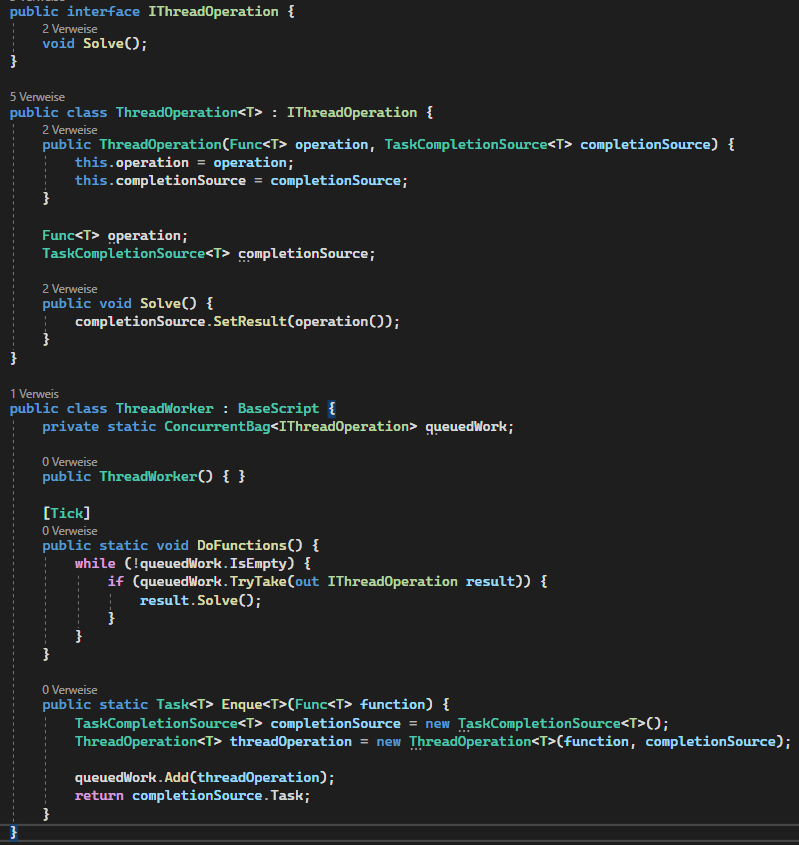
I don't think there is a TCS without generic parameter
I'd use bool or object there yeah