GetStreamAsync() Stream Always Null [Answered]
I'm getting a URI from json on my computer and trying to download an image from it.
Everything works up to actually putting something in
requestBodyStream
. It is forever null.
The next debug Step Into
would be to get the URI, which it shows by going to the model. Then it seems like it skips the next three lines of code and goes to the second object in the foreach loop. Pressing Step Into
again on the last item in the list of cards
finishes the program. Nothing is ever downloaded.
75 Replies
Are you debugging a release build, by any chance?
Probably not, because I don't even know what that means lol.
Unknown User•3y ago
Message Not Public
Sign In & Join Server To View
I might use a try/catch to see if you're getting any errors on the lines where it skips to the next item
I don't think that's what should happen if an exception is thrown, but I have had similar weird things happen before with async exceptions behaving strangely (Though I didn't think this would happen as long as your return type is Task)
that code works fine on my end (same url)
what you should seriously consider is putting
using
in front of many of those
this is all i didThey just get skipped. Breakpoint only triggers when creating the stream variable (inside the foreach loop)
Unknown User•3y ago
Message Not Public
Sign In & Join Server To View
Idk what that is but I'll Google it when I'm free later today
Unknown User•3y ago
Message Not Public
Sign In & Join Server To View
I really appreciate that advice. I'll try it in a few hours when free.
I imagine my method is a task, but I'm noob and maybe I forgot. I'll check.
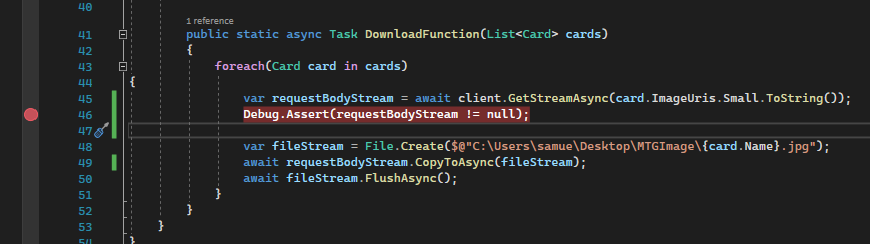
It's like there's no lines after the first
var requestBodyStream
line. Just skips it all completely. :[
Here's the whole thing. I hope this helps someone see what I did wrong.what's the
Card
class look like? what's in your LibrarySample.Json
? can't really do anything without thosesure I'll copy em all to $code
To post C# code type the following:
```cs
// code here
```
Get an example by typing
$codegif
in chat
If your code is too long, post it to: https://paste.mod.gg/I was just hoping there was something obviously wrong with a
Task
or await
or somethingonly thing i could see being a bit problematic is how often you do IO
(creating a lot of files in a short amount of time)
but i don't think that should break the program in any way
what .net version are you on?
how do I tell?
I could have googled that my b
right click the project > properties
net 6
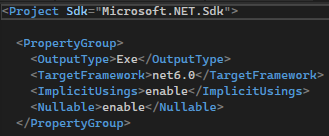
just making sure I read the right thing
👍
BlazeBin - zblyqyosyava
A tool for sharing your source code with the world!
BlazeBin - xdlkvejdugqt
A tool for sharing your source code with the world!
BlazeBin - zdkgmmnsbojo
A tool for sharing your source code with the world!
that's the three files in the project
you have multiple tabs in a single paste
i wasn't sure how it worked tbh
actually I'm not sure what you meant
sorry
the reason i asked about the .net version is because you don't really use block-scoped namespaces, or the explicit
Program
class declaration in .net 6 anymore
when you click the + here, you can paste multiple files
OHHH.
I was wondering about the sets lol
I also couldn't figure out how to name them lol
i don't think you can rename them once they exist
i just always delete the initial newfile.cs and create a new one so i can name it
BlazeBin - wtaoeebjayoe
A tool for sharing your source code with the world!
yeah had to delete the default and create new
to actually name. I fixed it now.
I also spaced and deleted the other ones lol. sorry
does your code ever even get into the function?
It performs the first line of the foreach
that's it
that's genuinely surprising since you're not awaiting
LocalCard()
well it steps into that first line, looks at the Card.cs model (highlighting Get) and then just repeats that for the two cards in the sampleLibrary
are you ignoring a bunch of warnings and messages or did you turn them off deliberately
warnings yes
cause you're missing a lot of null checks
👀
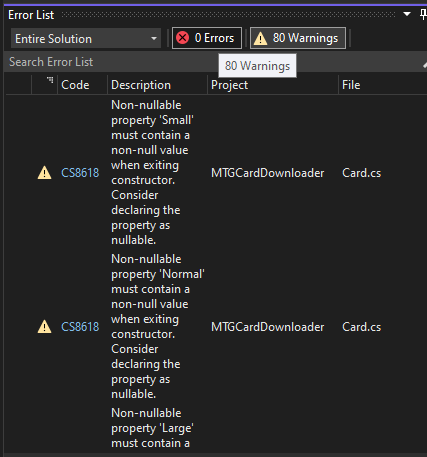
nah the json ones are fine to ignore
i'd just slap #nullable disable at the start of that file
I added await to the LocalCard thing and it did this
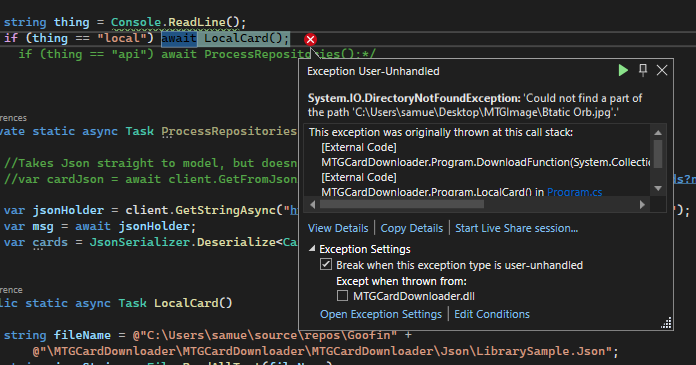
now it's actually executing the code :p
well like i said it'd hit breakpoint on first line of foreach before
which makes absolutely no sense actually
lol
did you turn off a warning for that too?
nope
it should have said "LocalCard is not awaited"
maybe I did and don't remember
ah yeah nevermind, it does execute the code
but i doubt it
but the program finishes before it method does
because you don't await
that's what i was figuring
cool

okay I need to google this await thing and read more.
Now I'm curious though why I got the file not found error
part of the path
var fileStream = File.Create($@"C:\Users\samue\Desktop\MTGImage\{card.Name}.jpg");
Doesn't this create the file for the filestream to dump data in?any one of the directories are missing
i assume you don't have an
MTGImage
folder on your desktopI do lol
I copied the path
to ensure it was correct.
works fine for me with an existing directory
consider switching away from
var
, consider making that httpclient local (with using
)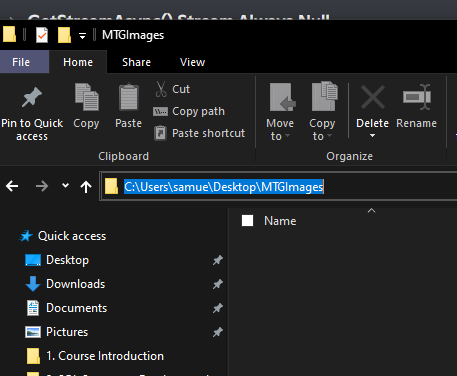
look again
holy fuck
That must have been from a frustration deleting
and not realizing when remaking
ffs
alright now to learn when I should use await lol.
dude tyvm
so I just await everything internet related?
lol
no no, await isn't related to anything web
oh lol
my only reference to await and task is internet stuff
so that was why the assumption
i can't really explain async development, i'm not that good at it either
no worries bud. You saved the day here, thank you
and sorry for sperging out at you the other day
It was my bad to respond that way
You typically await all async functions or functions which return a Task.
There is a bit more finesse to it, but you usually want them to complete before your next line(s) of code executes.
But yeah, that's what was happening - you were getting an exception in your code, but since you weren't awaiting your function, the debugger does not catch the exception since it does not propagate up. It'll look like the function just stopped working at some point
✅ This post has been marked as answered!
tyvm for explanation.