WPF cannot capture custom event using Microsoft.Xaml.Behaviors.Wpf (solved)
Suppose i have a project that raise an event. When i click on a button, obviously "Click" event will be raised. Inside the click handler method, i raised a custom event called "FileOpen" event.
I have successfully captured "Click" event by testing whether MessageBox popped or not. However, it fails to capture "FileOpen" custom event, it doesn't pop another MessageBox that tells "FileOpen event captured".
I'm guessing its because i put the wrong name (maybe wrong format, e.g must be fully qualified name or so) in the XAML of line
<i:EventTrigger EventName="FileOpen">
. So how do i capture this custom event?
XAML for the button:
11 Replies
Code behind for this XAML (for sake of this question, ignore the fact its not using viewmodel)
you are listening for the
FileOpen
event on the button, but its your windows CustomEventDemo
that has FileOpen
event 😉yeah i know i am raising the FileOpen event on the button, but since its a bubbling event, it should also raise (or travel) to the windows right? srry for late reply
routing strategy = bubble
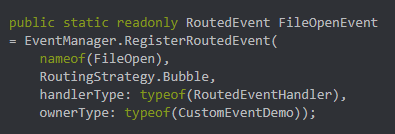
maybe i misunderstood how things work
i fired the
FileOpenEvent
on the button itself when button clicked as you can see in the code-behind screenshot, then i tried to capture that event inside the button itself and on the grid to see if my Microsoft.Xaml.Behaviors.Wpf
bubbling behaviour is working.
I do this because i want to handle my events inside my viewmodel (following MVVM pattern), but when i tried to capture the event without using Microsoft.Xaml.Behaviors.Wpf
by directly handling it in the view (e.g <Button FileOpen="HandlerName">
) it works flawlessly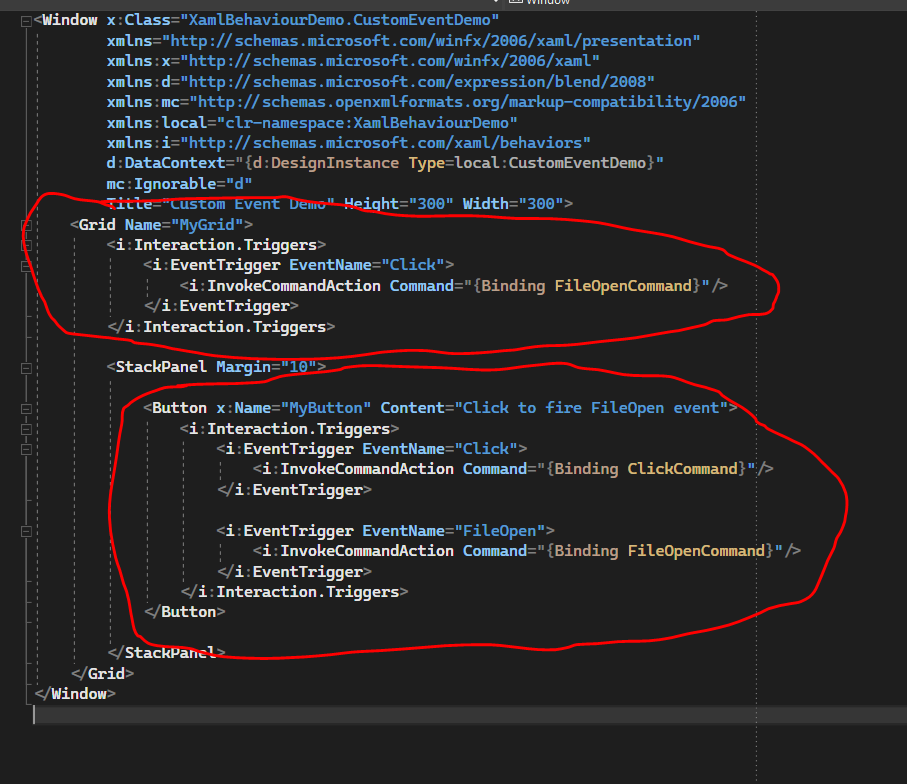
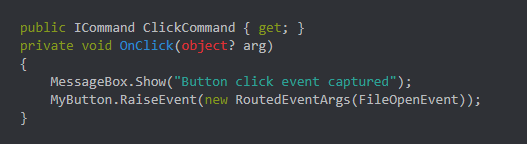
well, my
Microsoft.Xaml.Behaviors.Wpf
can capture event for builtin events, but for custom like mine does notas you can see, it works if i dont handle my event through
Microsoft.Xaml.Behaviors.Wpf
(by handling it using code-behind), but it's ugly, i want to follow MVVM patternoh i somehow missed the use of attached event :/
i dont think this is supported out-of-the-box, but check this out: https://github.com/microsoft/XamlBehaviorsWpf/issues/110
basically create your own
RoutedEventTrigger
:
and use it as your event trigger:
GitHub
[EventTrigger]Support for Attached Event? · Issue #110 · microsoft/...
Is your feature request related to a problem? Please describe. I'm using an attached event on a Border. public class ClickExtensions { public static readonly RoutedEvent ClickEvent = EventM...
oh wow it works. I dont know why it works though because i am new to WPF, at least it does exactly what i want. I will study depper about WPF such as frameworkelement and such eventually. Tysm man, you saved my life
i really appreciate that you spend your time here for helping me
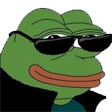
Currently this is how i do to handle events, by capturing it with the view, then i pass it to the viewmodel. I definitely will refactor my code using commands after known this technique
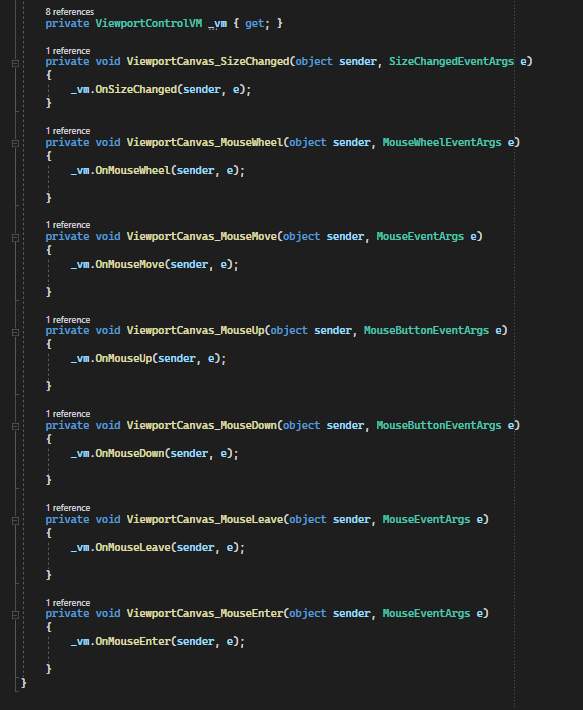