EF Core Global Query Filter Exception
Hi friends, I'm try to create a
Global query filter
for my EF Core
, to be honest this is the first time for me with Global Query Filters
In my AppDbContext
=> OnModelCreating
I tried this:
How my IArchivable
looks like:
The Issue:
When I start the app, automatically II get this exception
:
InvalidOperationException: The filter expression 'x => ((Convert(x.IsArchived, Nullable1) != null) OrElse (Convert(x.IsArchived, Nullable
1) == Convert(False, Nullable`1)))' specified for entity type 'ContinuousFormation' is invalid. The expression must accept a single parameter of type 'MBSM.Core.Entities.ContinuousFormation' and return bool.
Which ContinuousFormation
is an Entity type/class
and it looks like :
My Questions:
Please how do I can fix this issue ? and massive thanks in advance <320 Replies
The Exception screenshot:
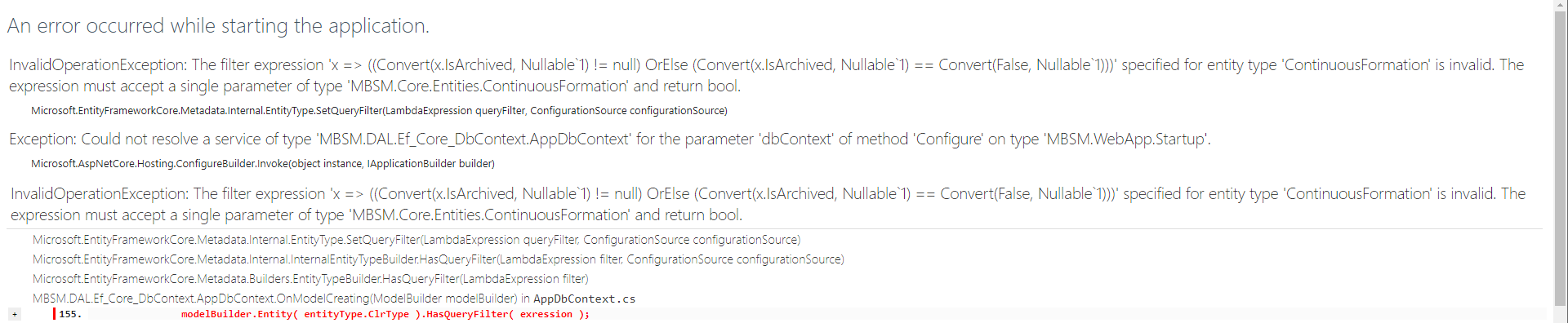
I'd wager you gotta look at getting the proper argument in the expression
which now is set as IArchivable and should be of the formentioned type
so you're going to have to rework how you get the filter expression tree using the parameter p
oh damn, btw I just forgot to let that
parameter
variable there
it is not a part of what I tryno but I believe it'll be part of your solution 😄
your passing an expression that takes IArchivable as argument, and I believe that's why he's complaining
as it expect ContiuousFormation as argument (or well, the entityType.ClrType)
hmmmm
so what should I do/ I change in my code ?
haven't done enough things with expression trees to know by heart, but you need to get a Expresion<Func<ContinuousFormation, bool>>
I imagine by either manually building it (using the parameter before) or trying to do some visitor replacement
omg if I use 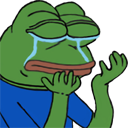
Expresion<Func<ContinuousFormation, bool>>
I need to use it with over than 50 entities
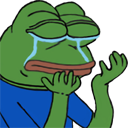
which is why you're better of trying to either replace the expression you made for the interface to use the specific type you have
or you make it more in a programmatic way
hmmmm do you have any peice of code you sggest to achieve that ?'
again I only get by with a little bit of expression tree work, so you'll probably be able to slim this down
and it's not complete but it might get you going
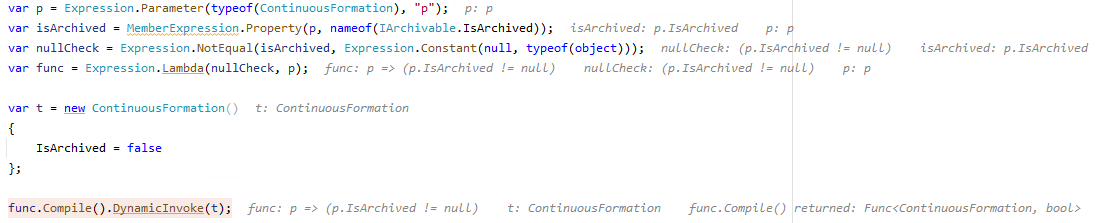
I really appreciate bro, I have another question if I want to add another check because at this time I just checked the
Null
but in the same time I want to check if the IsArchived
Equals false
too, because I want only the entities that are have IsArchived != null && IsArchived == false
Please how do I can transform this to an Expression
every part is another expression and for the most simple ones you have built in support
like isArchived is combined with null using the not equals
combine the expression you have using an andalso with a similar statement checking the value
sorry for annoying I do what you said it works perfectly but Ihave last issue IIdk how to combiine the current check with another check using the
OR
please can you provide a piece of code about it ?
And thanks in advanceyou can keep combining the binary expressions
how ?
Expression.OrElse( Expression.AndAlso(null check, value check), Expression.AndAllso( null check 2, valuecheck 2))
and this should be assigned to a variable ?
the result is another expression, you can use it similar or then use it as the input to convert it to a lambda function
@Greenthy really really appreciate you valuable help, massive thanks for it and your time
the finale solutions looks like