get value from parallel for loop using Task.WhenAll
hi all, im trying to implement a parallel for loop because of some timeout issue (the normal loop will loop through large dataset and it's taking lots of time so i try to use Task.WhenAll), im trying to process all items inside this await Task.WhenAll(tasks); line but im wondering how do we get the value returned by ProcessItem method because we put them inside the WhenAll to process, can anyone help to advise? thank you so much in advance..
3 Replies
it's only one item i need to retrieve that's a List, do i have to implement another for loop..?
no, i want the completed result from the ProcessItem method
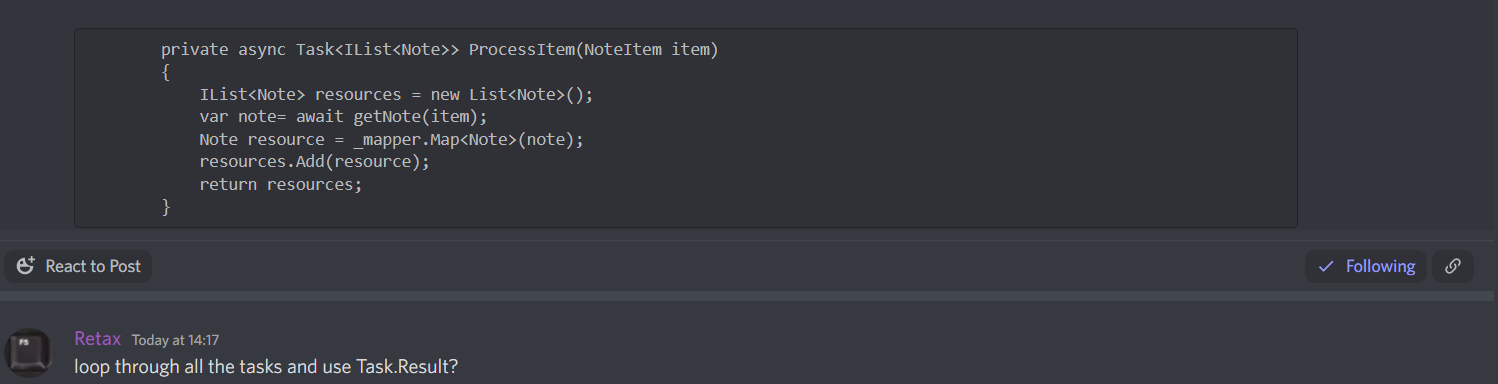
umm. what do you mean?
so how do we get the result from Task.WhenAll..?
is it to implement normal for loop and add the result from Task.WhenAll into another list?
i see.. is it possible if we maintain the result as a IList type instead of array type?
so like we only initialize it directly
IList<Note> resources = await Task.WhenAll(tasks);
i see.. hmm..
okay can.. i'll try it out.. thank you!! 🙏
wait.. i just realized this is not ok..
Cannot implicitly convert type 'System.Collections.Generic.List<System.Collections.Generic.List<Core.Models.Note.Note>>' to 'System.Collections.Generic.List<Core.Models.Note.Note>'
it becomes List of a List if we do var tasks = new List<Task<IList<Note>>>();
but our expected result is only a List
we have to loop through the tasks to add into List<Note> resources = new List<Note>();?
sry i edited the code, bcs the extension only support List instead of IList..
resources = (await Task.WhenAll(tasks)).ToList(); //this line
ohhhh
ohhh.. i try this out...
it's working, thank you for your help!
have a great day!!