Get multiple substrings from a main string
I feel like this should be basic knowledge, but whatever, imma ask anyways..
Say I have a string
base insert some esab weird strings base here esab
and I wanted to get every bit that is between two other strings, in this case base
as "start" and esab
as "end"
So I would want to get every substring between start, and end.
Beneath is what I expect to happen with the code.
I feel I should probably be using input.Substring(input.IndexOf(start), input.IndexOf(end) + end.Length)
or something, but it's missing something and I can't see it.57 Replies
it needs a loop and some offset from the previous iteration
dont forget that substring takes
startIndex, length
, not startIndex, stopIndex
and how would I do that?
something like:
(I don't think I did the loop right, but you get the gist right?)
yeah you're really close
you'll need a list instead of an array thou, because you dont know the size
Ah fair. (this is why I did
result.Length
but I guess a List would be easier)you also need an exit condition :p
what would be my exit condition though, because rn I don't think my input will ever reach nullOrEmpty 😅
you are not changing your input
hint: what does
.IndexOf
return if it doesnt find anything?-1 right?
correct
so eh,
do { stuff } while (input.IndexOf(start) + 1 != 0)
?
wait, no...
hang on 😅 I got to think with this now..I started with something very similar, but refactored it into a
while(true)
because my IndexOf will never return
-1
because I don't modify the input at allthats why you need the offset
yea I have this now:
but I am missing the offset
how do I offset
should I post my solution?
or do you wanna figure it out
I wanna figure it out, but I would like help in guiding my thoughts to the solution if you don't mind?
I learn more that way than pre-made code 😅
ok so you need an offset for your indexOf
so you dont find the same match forever
this offset needs to live outside the loop obviously
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
not sure how that helps, that part is already figured out
hrm...
😄
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
you are SOOO close to my pre-refactor solution
well lets just not introduce regexes
I deliberately didn't choose for regex, because there are some prerequisites to this that cannot be done with Regex
I know regex enough to do some things, but this is off topic 😅
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
What am I missing for my offset
let me re-iterate: "I cannot do that with regex" 🤣
offset > -1
but this is where I refactored
because I realized I had to set offset to a special value to signal the end
and at that point, it was cleaner to use a
while(true)
yea and I need to change it constantly in the loop
yeah but thats fine
you just set it to indexof end
but doesn't it find the same "iteration" of the end string?
example:
<sub>Some string here</sub> <sub>Another string here</sub>
now if I set offset to mainString.IndexOf(endString)
does it not set it to the very first </sub> every time?tbh I didnt set up unittests and try on a whole bunch of strings
I just ran on your first prompt
okay, lemme make a unit test for this one
it seems I should turn the do/while around. Because it sets itself to -1 and then does another loop??
at this code:
passes 🙂
GetBetween is my function (I made it an extension method)
okay, can I see your extension method then?
that would be my entire solution. you sure about it?
yea, then I can see what I missed
Ah, you did while(true) where I did do{}while() 😅
yup, and the offset needs to be added to the correct place 😛
yea I didn't
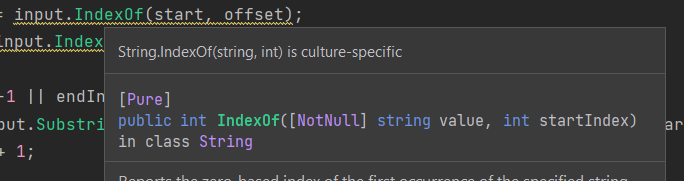
Right,
I'm dumbdumb 🤣 but I see what I did wrong now
Wait, why did you set the offset to
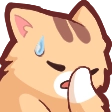
endIndex + 1
?because I did 😄
otherwise it errors out on length
because the index will be greater than the string length, if the end is the very last thing
Guess that works
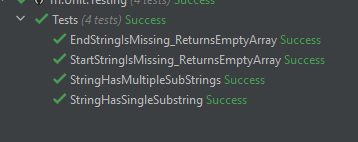
these are the tests I used 🙂
using
Shouldly
for the assertsI'm using
FluentAssertions
😅
but then again, I use nUnit instead of xUnityeah I love that one
its what I use for more serious projects
Heck yes. Plus I get smacks from the senior here if I don't test for failed cases too 🤣
hence the "Is Missing" tests
sure
I can't however test my Azure Function (or don't know how) the same way
lets just say, I didn't bother writing production quality tests for this 😄
hahaha no I recon, though I might have to, so I'll spend the next 45 minutes of the Hackaton here to work out how to Unit Test on Azure Functions
thanks for your help man!
much appreciated
hm? you just test the actual functionality locally
oh you code directly ON the azure function? eugh
No I do it in Rider.
riiight
then just run the tests locally on rider too?
for some reason, I can't even run the azure function in the debugger, and if I call the Azure Function from my nUnit Tests, then I need to pass the parameters that Azure Functions require, which I cannot instantiate from nowhere...
those parameters
anyways, brb. I finally have 5 minutes for a smoke lol